
Extracting metadata from .dm3 files
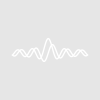
daggaz
Advice/tips much appreciated. Thanks.
EDIT: dm3 is a file format used by Gatan Digital Micrograph and are common in the microscopy world. Its basically a tiff with some metadata tucked in. I threw in an example file (its soot imaged with HRTEM if anybody is curious), need to rename it from .jpg to .dm3 as I couldnt upload it otherwise.
Here igor always complains with "out of memory" if I try to load your file (renamed to tiff before).
November 20, 2014 at 03:51 am - Permalink
November 20, 2014 at 04:59 am - Permalink
A.G.
WaveMetrics, Inc.
November 20, 2014 at 12:45 pm - Permalink
November 21, 2014 at 12:31 am - Permalink
No guarantees that this is right.. but it should help you get something. Maybe someone can tidy up the code and make it more robust.
The meta data is put into a Notebook. I have suppressed the image data itself being put there.
Hope this helps,
Kurt
Code as follows:
November 21, 2014 at 01:16 am - Permalink
I had almost given up on this, and got distracted by work for some time, only to come back and find all this great help. I was otherwise looking at doing this manually for several hundred images (and that is just one week's work).
I love these forums, really.
EDIT: Acquisition time is given as the ushort data (all the meta data is provided in the raw format), I've decoded the following
0-9 = 48-57
: = 58
space = 32
P = 80
M = 77
A should follow from this to be equal to 65 (77 - 12)
Now to hijack this script so that it pulls this out from a group of files, translates it and writes it to a wave. Fun fun =)
May 5, 2015 at 05:20 am - Permalink
Is it possible to search the entire open file for a specific string and then get that string position? This would be significantly faster, I imagine.
Here is the modified code if anybody wants to use it. Its ugly after I got done with it.
May 13, 2015 at 05:44 am - Permalink
Just a thought - you could use
FStatus
to find the length of the file, then usePadString
andFBinRead
to read the entire file into a string variable. Then finally search this for your specific string usingstrsearch
.I don't know whether this would be faster.
HTH,
Kurt
May 13, 2015 at 11:43 pm - Permalink
May 18, 2015 at 12:13 am - Permalink