
How to assign slices of one wave to another?
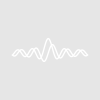
bendrinksalotofcoffee
I'm trying to make a slider that creates a new wave dynamically from two others by combining them at the index selected by the slider. This would be incredibly easy in Python. If I had two inputs, A and B, and an output C, in Python, using lists, I would write:
C[:sliderValue] = A[:sliderValue]
C[sliderValue:] = B[sliderValue:]
C[sliderValue:] = B[sliderValue:]
I wouldn't even have to know how big the lists were, as long as they were all the same size.
How would I do this in Igor? Here's my slider proc:
Function SliderProc(sa) : SliderControl
STRUCT WMSliderAction &sa
switch( sa.eventCode )
case -3: // Control received keyboard focus
case -2: // Control lost keyboard focus
case -1: // Control being killed
break
default:
if( sa.eventCode & 1 ) // value set
Variable sliderValue = sa.curval
wave/z a=root:input_a
wave/z b=root:input_b
wave/z c = root:output
if (waveexists(c))
// This is where I need to set the output
endif
endif
break
endswitch
return 0
End
STRUCT WMSliderAction &sa
switch( sa.eventCode )
case -3: // Control received keyboard focus
case -2: // Control lost keyboard focus
case -1: // Control being killed
break
default:
if( sa.eventCode & 1 ) // value set
Variable sliderValue = sa.curval
wave/z a=root:input_a
wave/z b=root:input_b
wave/z c = root:output
if (waveexists(c))
// This is where I need to set the output
endif
endif
break
endswitch
return 0
End
Bonus question: what does Igor call a slice? Is it a subwave? Subset? I can't find anything on this topic without weeding through image processing posts.
C[sliderValue,] = B
DisplayHelpTopic "Understanding Wave Assignments"
September 18, 2024 at 01:13 am - Permalink
I'd also recommend getting acquainted with the MatrixOP operation as it might help you write more efficient code. In particular, checkout MatrixOP functions subRange(), subWaveC() and subWaveR().
September 18, 2024 at 09:19 am - Permalink
Note that Tony's solution specifically uses the lowest dimension of a wave / data (which is also the only dimension of a 1D wave). You need to think a bit more about the assignment in case of multi-dimensional waves such as images. Also, regarding your 'bonus' question, I think you want to look for 'subrange'. While it is not easily possible to pick multiple individual elements of data in a single wave assignment in Igor such as with using 'slice()' in python, you usually can achieve something similar without too much hassle. If you want to look into subranges for wave assignments you can find this in the help chapter mentioned by Tony, specifically here:
DisplayHelpTopic "Indexing and Subranges"
September 19, 2024 at 01:12 am - Permalink