
Speed of wave assignments using wave reference waves
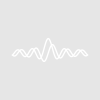
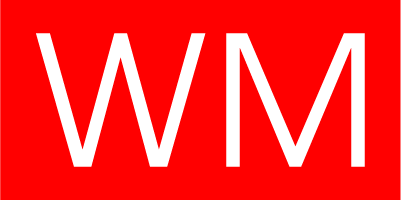
I've found that when I'm using a wave reference wave, my code actually runs faster if I declare each wave I want to use as it's own wave object before operating on it:
... = myWave * x
as opposed to just operating directly on the indexed wave reference wave:
... = waveRefWave[0] * x
In this test function, I make two 3D waves that are just added together into an output wave. The first loop does the extra wave assignment step, and is always a decent bit faster than the second loop, which operates directly on the wave reference wave. This doesn't make a lot of sense to me, but I might just misunderstand something about wave reference waves that goes on under the hood. Thoughts?
//Make two 3D waves and a wave reference wave
Make/O/N=(500,500,200) root:wave0,root:wave1
Wave/WAVE waveRef = ListToWaveRefWave("root:wave0;root:wave1;")
//Make the output wave
Make/O/N=(500,500,200) root:outputWave
Wave outWave = root:outputWave
//Run the loop WITH a separate wave assignment step
Variable ref = StartMSTimer
Variable i = 0
Do
Wave theWave = waveRef[i]
Multithread outWave += theWave
i += 1
While(i < 2)
print "Assigned:",StopMSTimer(ref)/(1e6),"s"
//Run the loop WITHOUT the separate wave assignment step
ref = StartMSTimer
i = 0
Do
Multithread outWave += waveRef[i]
i += 1
While(i < 2)
print "Not assigned:",StopMSTimer(ref)/(1e6),"s"
End
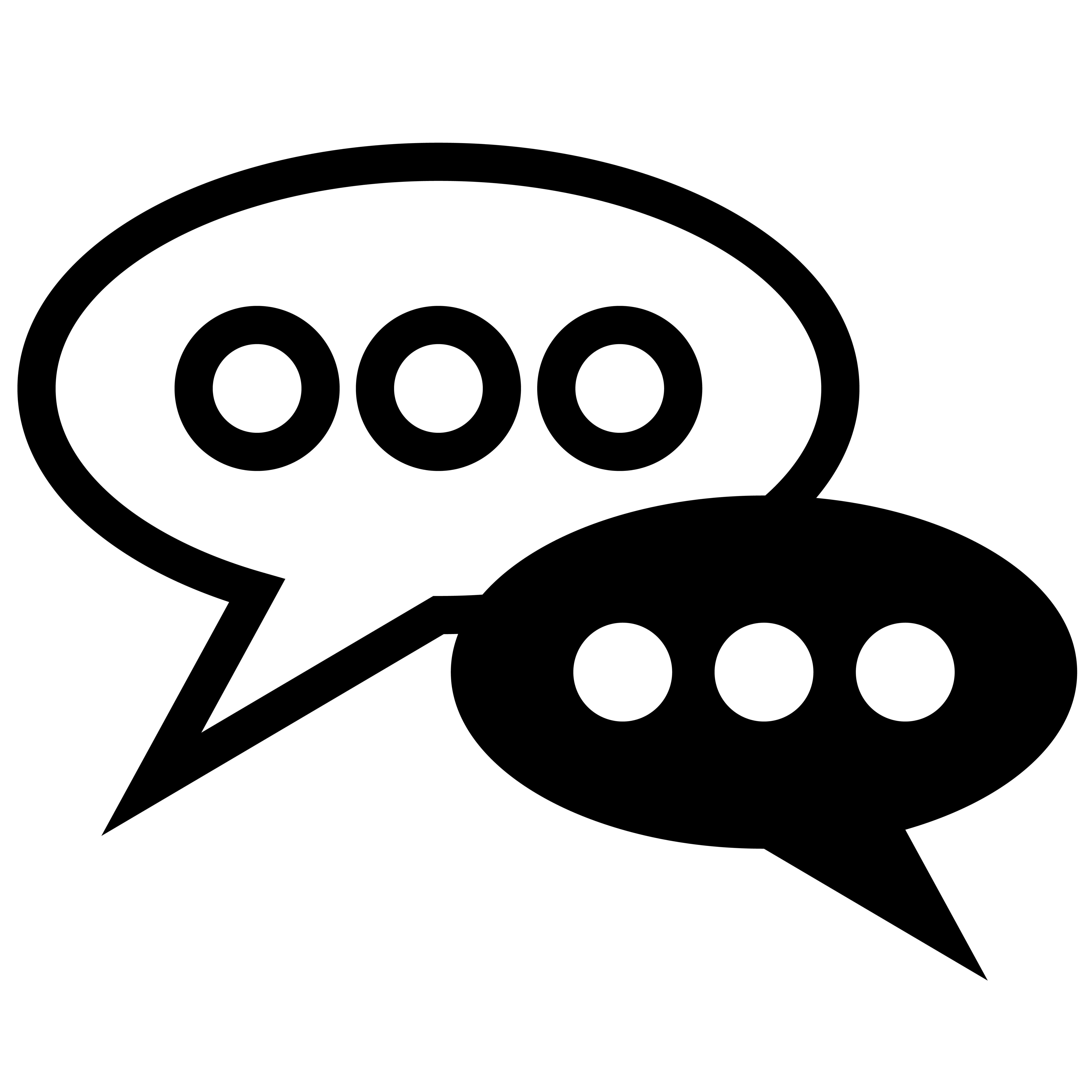
Forum
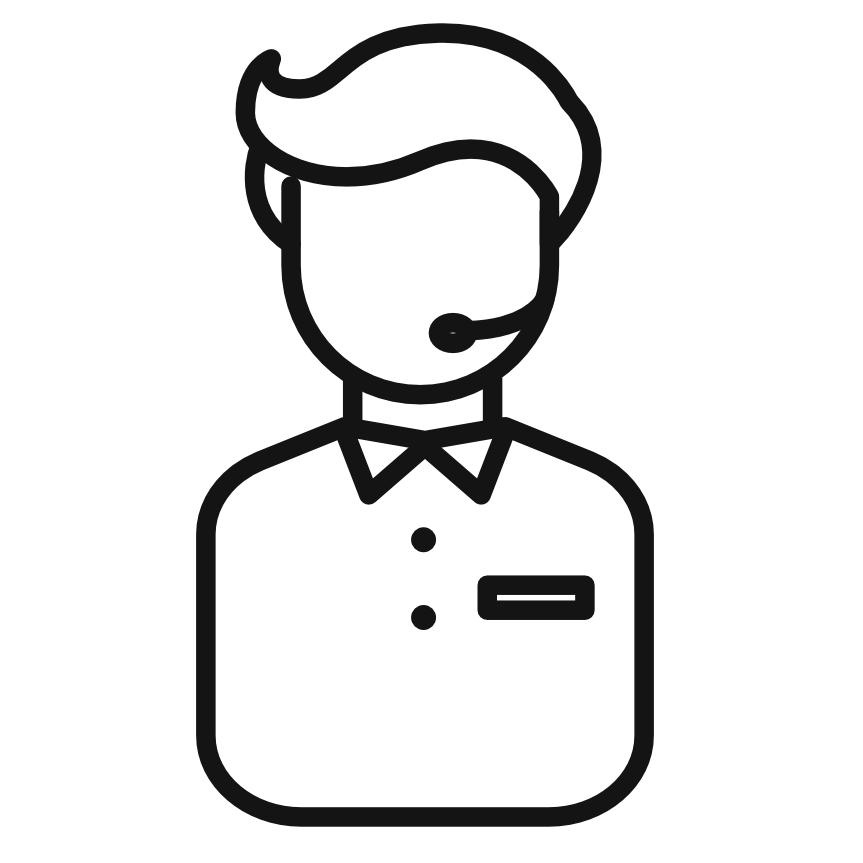
Support
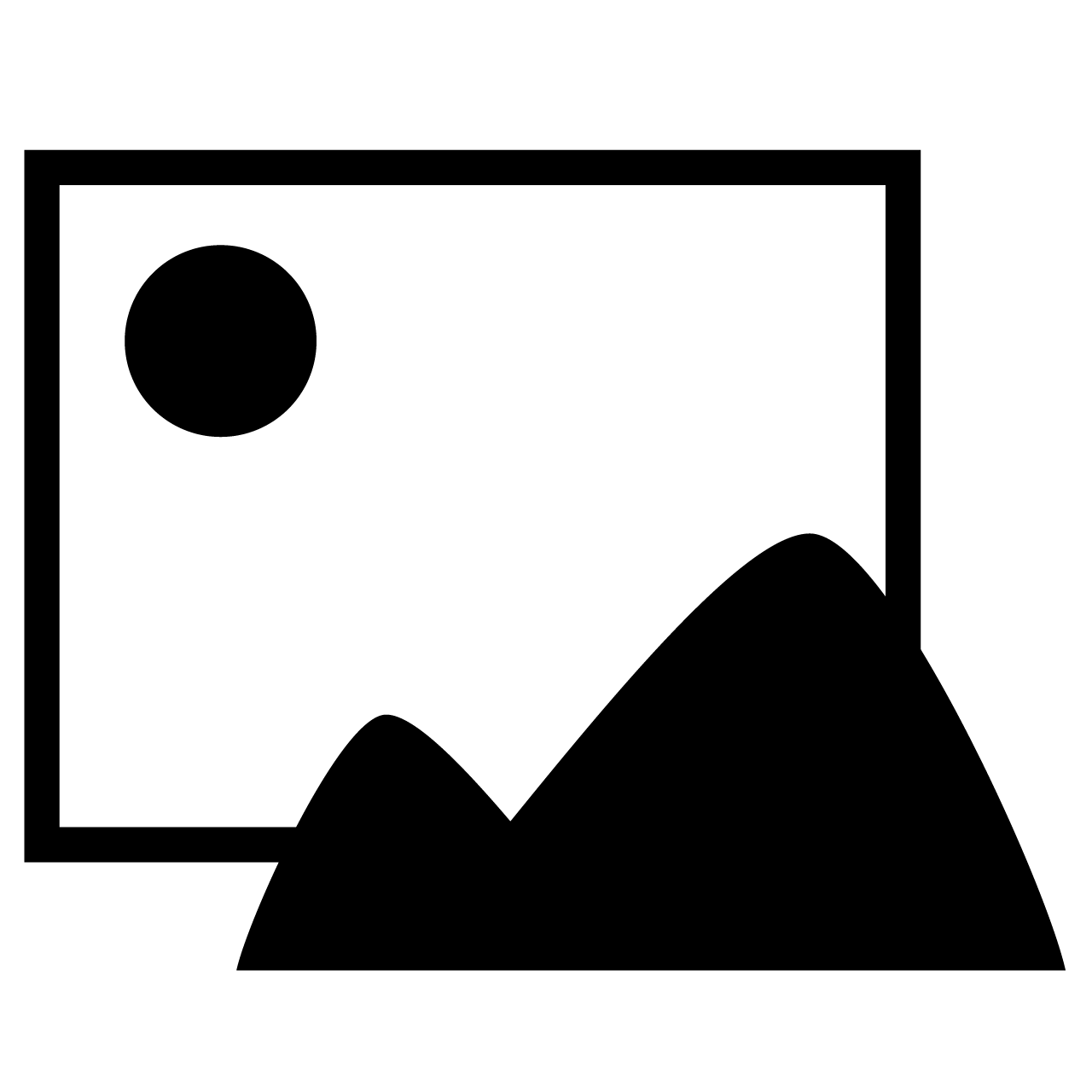
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More
If you write something like MyWave[] = waveRefWave[0] * x I assume the wave declaration is done over and over again for each point of MyWave
February 16, 2020 at 03:08 am - Permalink
How many enries does the wave reference wave has? I suppose two is just for playing around? If yes, have you seen https://www.wavemetrics.com/code-snippet/using-wave-reference-waves-mul…? This would allow you to get rid of the do/while loops and thus should be much faster.
How much is a decent bit faster?
February 16, 2020 at 11:40 am - Permalink
My tests with Igor 8.04 on Windows, using a machine with an 8 core/16 thread processor, show no consistent difference between the two:
Assigned: 0.500324 s
Not assigned: 0.477222 s
•testfunc()
Assigned: 0.453647 s
Not assigned: 0.516939 s
•testfunc()
Assigned: 0.481404 s
Not assigned: 0.483658 s
February 16, 2020 at 07:26 pm - Permalink
In reply to My tests with Igor 8.04 on… by aclight
Interesting. I just did 20 repeat tests of it and the 'assigned' version was faster every time.
Assign: 1.29 s avg
Not assigned: 1.47 s avg
I'm on 8.05 Mac, 4 core i7
February 17, 2020 at 08:15 pm - Permalink
In reply to If you write something like… by olelytken
@olelytken, that might make sense, but my (mistaken?) impression was that the wave is already declared as waveRefWave[0], so no red-declaration has to happen.
February 17, 2020 at 08:17 pm - Permalink
In reply to How many enries does the… by thomas_braun
@Thomas, yes just two entries for the purposes of testing this out. It's about 170 ms faster for me on this code, so about 11%. It seems to be constant though-- if I take out the multithreading, 'Not assign' is about the same amount slower than 'Assign', even though the overall runtime is much slower. And I hadn't seen that before, but that's very useful thank you!
February 17, 2020 at 08:22 pm - Permalink
OK, I see the problem, my original unassigned loop wasn't doing what I thought and may just be copying the wave reference itself into all the rows/cols/layers of the output wave. Looks like I do need to explicitly declare each wave reference in the wave ref wave before using it.
February 17, 2020 at 11:30 pm - Permalink
In reply to OK, I see the problem, my… by Ben Murphy-Baum
This seems like a bug to me. I'll report this to the author of that code.
February 18, 2020 at 08:02 am - Permalink
@aclight Could you post here once that is resolved? I'd like to have a look if and how that influences my code.
February 19, 2020 at 08:14 pm - Permalink
In reply to @aclight Could you post here… by thomas_braun
Larry has changed Igor 9 so that this gives a compile error on the dd += ww[i] line:
Make/O wave0 = 1, wave1= 2
Make/O ddd = 0
Make/O/N=2/WAVE ww
ww[0]=wave0
ww[1]=wave1
Variable i = 0
Do
ddd += ww[i]
i++
While (i<2)
End
Instead, you would need to write the loop like this:
WAVE theWave = ww[i]
ddd += theWave
i++
While (i<2)
February 20, 2020 at 07:53 am - Permalink