
trouble shoot: WaveRefIndexed
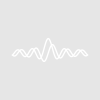
ychen344
I am trying to obtain a list containing all the wave paths in a table using "WaveRefIndexed(windowNameStr, index, type)" as the following:
FFTWaveList = WaveRefINdexed ($tableName, "", 1)
A few questions:
1. "$" is not allowed: how do I refer to pre-defined "tableName", e.g, if tableName = "FFT_R1_trial1"?
2. What do I put for "index" if I want to collect all the columns in the table? I tried "", which failed to compile.
Thanks,
Judy
NOTE: I changed the name of the GetWaveListFromTable to GetWaveRefsFromTable to be more accurate.
The first parameter to WaveRefIndexed is documented as windowNameStr. The "Str" suffix indicates that the parameter is a string, not a name. $ converts a string to a name but we want a string here, not a name, so $ is not needed.
To get a list of all wave references for all waves in the table you will need a loop.
Here is a lightly-tested solution:
Make/WAVE/N=0/FREE refs
int index = 0
do
int wType = 1 // Data columns only
WAVE/Z w = WaveRefIndexed(tableName, index, wType)
if (!WaveExists(w))
break
endif
Redimension/N=(index+1) refs
refs[index] = w
index += 1
while(1)
return refs // Free wave reference wave
End
Function Demo()
KillWindow/Z DemoTable
Make/O demoWave0, demoWave1
Edit/N=DemoTable demoWave0, demoWave1
WAVE/WAVE refs = GetWaveRefsFromTable("DemoTable") // Free wave
int i
int numWaves = numpnts(refs)
for(i=0; i<numWaves; i+=1)
WAVE w = refs[i]
String name = NameOfWave(w)
Printf "%d: %s\r", i, name
endfor
End
The GetWaveRefsFromTable function returns a free wave containing wave references. For details on free waves, execute:
DisplayHelpTopic "Free Waves"
For details on wave reference waves, execute:
DisplayHelpTopic "Wave Reference Waves"
August 29, 2024 at 09:11 pm - Permalink
Thank you for helping with the WaveRefIndexed function. After I obtain the wave references for all waves in the table, I need to average the waves using:
fWaveAverage(waveReferences, "", 0,0,"AveWave","")
I am having trouble finding the "fWaveAverage" function in the manual book. Looks like the first part in the parenthesis should be "refs". What do the other elements in the parenthesis stand for?
Also, when I manually averaged waves within a table using "Analysis-Package-Average Waves," the function outputted 2 parameters: W-WaveAverage and W_WaveAveError. How do I accomplish the same with "fWaveAverage"?
Thanks,
Judy
August 30, 2024 at 09:11 am - Permalink
NOTE: I changed the name of the GetWaveListFromTable (above) to GetWaveRefsFromTable to be more accurate.
fWaveAverage is a function added by the "Waves Average.ipf" WaveMetrics procedure file. To use it you need to add this to your procedure file:
The first parameter to fWaveAverage is a string list of wave names or paths to waves, not a wave reference wave.
Here is a function that illustrates using fWaveAverage. As noted in a comment, it works only if all of the waves are in the same data folder:
Function Demo2(String tableName)
KillWindow/Z DemoTable
Make/O demoWave0={1,2,3}, demoWave1={2,3,4}
Edit/N=DemoTable demoWave0, demoWave1
String optionsStr = "WIN:" + tableName
String list = WaveList("*", ";", optionsStr)
String outputErrorWaveName = "StdDevOfWavesInTable"
String outputWaveName = "AverageOfWavesInTable"
int errorType = 1 // Standard deviation
int errorInterval = 1 // I don't know what this parameter means
fWaveAverage(list, "", errorType, errorInterval, outputWaveName, outputErrorWaveName)
AppendToTable $outputWaveName, $outputErrorWaveName
End
Here is a function that works with fWaveAverage even if the waves are not in the same data folder. It uses the GetWaveRefsFromTable function above:
Function Demo3(String tableName)
KillWindow/Z DemoTable
NewDataFolder/O root:DF0
Make/O root:DF0:demoWave0={1,2,3}
NewDataFolder/O root:DF1
Make/O root:DF1:demoWave1={2,3,4}
Edit/N=DemoTable root:DF0:demoWave0, root:DF1:demoWave1
WAVE/WAVE refs = GetWaveRefsFromTable(tableName) // Free wave
String list = ""
int i
int numWaves = numpnts(refs)
for(i=0; i<numWaves; i+=1)
WAVE w = refs[i]
String path = GetWavesDataFolder(w, 2) // Full path including wave name
// Printf "%d: %s\r", i, path
list += path + ";"
endfor
String outputWaveName = "AverageOfWavesInTable"
String outputErrorWaveName = "StdDevOfWavesInTable"
int errorType = 1 // Standard deviation
int errorInterval = 1 // I don't know what this parameter means
fWaveAverage(list, "", errorType, errorInterval, outputWaveName, outputErrorWaveName)
AppendToTable $outputWaveName, $outputErrorWaveName
End
August 31, 2024 at 09:26 am - Permalink
Thank you, hrodstein! I updated my script by including "fWaveAverage" (script attached). The program is able to return the average and standard deviation. However, there are a couple of glitches as below.
(1) When I ran the script, looks like "newdatafolder" did not pass, leading to the following error message. (The glitch in the script bolded.) The outputted strings, "newdatafolderName" and "path" look appropriate since I can use them in Command window to create the desired new datafolder and setdatafolder to the newly created folder.
(2) Also, "Analysis-Package-Average Waves" returns standard error whereas the "fWaveAverage" returns standard deviation. Is there another errorType that can return standard error?
=============================================================
menu "Macros"
"average FFTs", createAverageFFTsDialog()
End
Function createAverageFFTsDialog()
variable age_min, age_max, sexNum1, sexNum2
string PCAstatus
prompt age_min, "Enter minimum age"
prompt age_max, "Enter maximum age"
prompt sexNum1, "Enter sex1"
prompt sexNum2, "Enter sex2"
prompt PCAstatus, "Enter PCA status", popup, "Aging;noAging;all" //aging is PCA<-1, noAging is PCA>=-1
DoPrompt "Enter group specs", age_min, age_max, sexNum1, sexNum2, PCAstatus
//create new data folder
string newdatafolderName = "'avgFFTs_artRatio15Perc_"+num2str(age_min)+"-"+num2str(age_max)+"_"+num2str(sexNum1)+"-"+num2str(sexNum2)+"_"+PCAstatus+"'"
string path = "root:" + newdatafolderName
newdatafolder path
setdatafolder path
//create "numWavesAverage" to summarize the number of waves averaged in each table
make/T/FREE outputNameList = {"PDmm", "R1", "R2", "B1", "B2", "postR", "postB"}
make/WAVE/N=0/FREE FFTWaveList //create a wave to hold the list of wave references from each table
string list //list is the string list of all waves in the table
string pathName //pathName is the full path including wave name for each waves in the table
variable trialNumber, c, i
Int index, numWaves
string FFTavgName, FFTavgErrorName
string tableName
for (trialNumber=1; trialNumber<=2; trialNumber+=1)
for (c=0; c<7; c+=1)
tableName = "FFT_"+outputNameList[c]+"_trial"+num2str(trialNumber)
//obtaining wave references of all waves in the table
index = 0
Do
WAVE/Z w = WaveRefIndexed(tableName, index, 1)
if(!WaveExists(w))
break
endif
redimension/N=(index+1) FFTWaveList
FFTWaveList[index] = w
index += 1
while(1)
//obtaining string list of all waves in the table
list = ""
numWaves = numpnts(FFTWaveList)
for (i=0; i<numWaves; i+=1)
WAVE w=FFTWAVEList[i]
pathName = getwavesdataFolder(w, 2)
list += pathName + ";"
endfor
//averaging waves in the table
FFTavgName = "W_WaveAverage_"+outputNameList[c]+"_T"+num2str(trialNumber)
FFTavgErrorName = "W_WaveAveError_"+outputNameList[c]+"_T"+num2str(trialNumber)
fWaveAverage(list, "", 1, 1, FFTavgName, FFTavgErrorName)
endfor
endfor
end
==========================================================================================
Many thanks,
Judy
September 2, 2024 at 07:18 am - Permalink
To insert Igor code in a post, click "Insert Code Snippet". This will format the code properly. For details, see https://www.wavemetrics.com/forum/general/formatting-code-forum-posts
NewDataFolder and SetDataFolder take a path as a parameter, not a string, so you need to use $path instead of path as the parameter.
Change the third parameter of the fWaveAverage call from 1 to 3. I discovered this by right-clicking "fWaveAverage" and reading the comments for the parameters.
September 2, 2024 at 08:27 am - Permalink
Haaa - I wondered how to make the pasted code look original. Thank you for the tips!
Where do you right-click? I tried within the Procedure window and at the bottom of the Data Browser window, and I had trouble seeing the comments.
Thanks,
Judy
September 2, 2024 at 08:47 am - Permalink
You can right-click the word "fWaveAverage" in your procedure file and choose "Go to fWaveAverage". This opens the "Waves Average" procedure file and displays the code for fWaveAverage.
September 2, 2024 at 03:57 pm - Permalink