
Split and Shuttle Wave Order
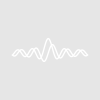
thatguy91
Hello,
I am attempting to generate a function that will take the list of waves on a plot, split them in half, and then integrate the top half and bottom half. Similar to this:
XXXXaaaa
to
XaXaXaXa
I am a bit stumped on the best way to go about this. I have a script from a previous colleague used to flip the order of traces on a graph and have attempted to modify it but have not been successful.
Any tips or tricks on how to go about this would be greatly appreciated.
Thanks!
Function ShuffleSort() // Mix bottom half of waves into top half
String Traces = TraceNameList("",",",1)
String TopTraces = Traces
if( strlen(Traces) )
Traces= ReverseList(Traces, ",")
Traces= RemoveEnding(Traces, ",")
String cmd="ReorderTraces _front_,{"+traces+"}"
Execute cmd
endif
End
String Traces = TraceNameList("",",",1)
String TopTraces = Traces
if( strlen(Traces) )
Traces= ReverseList(Traces, ",")
Traces= RemoveEnding(Traces, ",")
String cmd="ReorderTraces _front_,{"+traces+"}"
Execute cmd
endif
End
Function/S ReverseList(list, sep)
String list
String sep
Variable i, n = ItemsInList(list, sep)
String reversed=""
for (i = 0; i < n; i += 1)
String item=StringFromList(n - 1 - i,list, sep)
reversed += item + sep
endfor
return reversed
End
String list
String sep
Variable i, n = ItemsInList(list, sep)
String reversed=""
for (i = 0; i < n; i += 1)
String item=StringFromList(n - 1 - i,list, sep)
reversed += item + sep
endfor
return reversed
End
I'm sure there's a more elegant way to do this, but I think this should get you there. It basically iterates over the second half of the trace list and reorders them one at a time into the first half. For code like this where the logic can be hard to read, it helps to step through the code in the debugger, and have it print out the reordered trace list each time through the loop.
String Traces = TraceNameList("",",",1)
Variable nTraces = ItemsInList(Traces, ",")
Variable middleTrace = ceil(nTraces / 2)
Variable i
for (i = 0 ;i < middleTrace - 1; ++i)
String middleTraceName = StringFromList(middleTrace + i, Traces, ",")
ReorderTraces $StringFromList(i + 1, Traces, ","), {$middleTraceName}
endfor
End
July 24, 2024 at 09:35 am - Permalink
Hi,
here is a quick stab at a function that makes use of listtotextwave and point shuffling and assuming that the list in is semi-colon separated.
wave/T temp= listToTextWave(listin,";")
duplicate/O/T temp ,temp2
temp2[0,;2]= temp[floor(p/2)]//set even points
temp2[1,;2]= temp[floor(numpnts(temp)/2)+floor(p/2)]//set odd points
string listout
wfprintf listout,"%s;",temp2
return listout
end
Andy
July 24, 2024 at 09:40 am - Permalink
Here's my crack at it:
WAVE wIn // Assumed to have an even number of points
int numPoints = numpnts(wIn)
String wOutName = NameOfWave(wIn) + "_out"
Duplicate/O wIn, $wOutName
WAVE wOut = $wOutName
wOut = NaN
wOut = (p&1) ? wIn[(numPoints-1+p)/2] : wIn[p/2]
return wOut
End
Function Demo()
Make/O testWave = {0,2,4,6,8,1,3,5,7,9}
Print testWave
WAVE wOut = ReorderWavePoints(testWave)
Print wOut
End
July 24, 2024 at 10:47 am - Permalink
Not knowing how to code this but, assuming the order is all X's then all a's ... Transform a 1-D wave of 2N points to a 2-D matrix of (Nx2) points, transpose the matrix, and transform back to 1-D wave of 2N points. Would this shuffle the sequence properly?
July 26, 2024 at 01:06 pm - Permalink
In reply to Not knowing how to code this… by jjweimer
Cool idea, it does work pretty nicely. This code assumes an even number of points.
Variable npnts = DimSize(w,0)
Redimension/N=(npnts/2, 2) w
MatrixOP/O w = w^t
Redimension/N=(npnts) w
End
July 26, 2024 at 06:21 pm - Permalink