
How to find the last nonzero element in an array where zeros are sprinkled throughout?
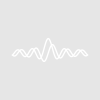
bendrinksalotofcoffee
Suppose I have a 1D wave that looks like:
{2,5,8,0,1,4,33,6,3,7,0,8,0,3,1,8,99,0,0,0,0,0,0,0,0}
I want to find the index of 99, which of course won't always be 99. It's just the last nonzero value before a trail of zeroes. I have hundreds of these waves and the last nonzero value is in a different place each time. The waves are also different lengths.
In Python, I would write
idx = numpy.max(numpy.nonzero(wave))
Or, without numpy:
idx = [index for index, item in enumerate(wave) if item != 0][-1]
But Igor doesn't have anything so elegant. Do I have to make a new reversed wave, then search element-by-element until I find something nonzero, then figure out what the non-reversed index is?
Hi,
One approach
replace 0 with nans, zapnans, read last value.
Alternative
loop from the end
then the value is wave[i] and the point where that occurs is i
Andy
September 30, 2024 at 09:23 am - Permalink
Hmm... somehow Andy's code is very broken today. Here is how this should look:
Try:
September 30, 2024 at 09:47 am - Permalink
Here is another solution that works with your data:
September 30, 2024 at 10:21 am - Permalink
In reply to Hmm... somehow Andy's code… by chozo
That it no more decaf! Thanks for the corrections Chozo.
Andy
September 30, 2024 at 10:38 am - Permalink
Here is another solution that eliminates the need for Reverse using /R with swap parameters:
September 30, 2024 at 10:50 am - Permalink
... or you can use MatrixOP:
The index of the last non-zero element of the wave ddd is:
The data at that wave point is:
print ddd[aa[0]]
September 30, 2024 at 04:42 pm - Permalink
I think that you need to subtract one from the index in hrodstein's solutions if you want to find the last non-zero point.
You can construct for yourself an equivalent to numpy's nonzero:
October 1, 2024 at 01:53 am - Permalink
Here's yet another solution. It may be that Extract does more work than necessary, but it's simple and easy to understand:
October 1, 2024 at 09:41 am - Permalink
Tony is right. My solutions found the last zero value. I have corrected them. Thanks Tony.
October 1, 2024 at 09:52 am - Permalink