
Troubleshoot: remove NaNs from a numeric name
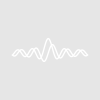
ychen344
I am trying to delete NaNs from a numeric wave with the following. The function removes most of the NaNs but there are still NaNs (blank) left in wave0. Any idea?
wavestats/Q wave0 //wave0 is a numeric wave for (p = V_npnts-1; p >= 0 ; p -= 1) if (numtype(wave0[p]) == 2) deletePoints p, 1, wave0 endif endfor
Thanks,
Judy
If it's a 1D wave, you can use
WaveTransform zapnans wave0
There's a MatrixOp equivalent for multidimensional waves, but I think WaveTransform is what you are after.
Your code probably isn't working because you are using `p` as a counter and it is reserved for the point number. Probably if you replace the `p` with `i` it would run, but anyway WaveTransform will do what you want.
June 8, 2024 at 11:52 am - Permalink
There are two problems with your original code.
1. You did not declare the variable p. (p is a built-in function so it is better to avoid using p as a variable but you can do it.)
2. WaveStats returns the number of "normal" (not NaN or INF) points via V_npnts, not the total number of points in the wave.
Here is a version that will work:
Make/O wave0 = {0,1,NaN,3,NaN,5}
WaveStats/Q wave0
Variable p
Variable totalPoints = V_npnts + V_numNaNs + V_numINFs
for (p = totalPoints-1; p >= 0 ; p -= 1)
if (numtype(wave0[p]) == 2)
DeletePoints p, 1, wave0
endif
endfor
End
If is better to use numpnts than to use WaveStats for this purpose as WaveStats does much more than you need. Here is a version that does not use p as a variable and uses numpnts instead of WaveStats:
Make/O wave0 = {0,1,NaN,3,NaN,5}
Variable pointNum
Variable totalPoints = numpnts(wave0)
for (pointNum = totalPoints-1; pointNum >= 0 ; pointNum -= 1)
if (numtype(wave0[pointNum]) == 2)
DeletePoints pointNum, 1, wave0
endif
endfor
End
As sjr51 says, you can use:
WaveTransform zapNaNs, wave0
June 8, 2024 at 07:16 pm - Permalink
Thank you for your prompt response!
I tried different ways that you recommended, and they all worked! You are right about V_npnts and NaNs. If I go with wavestats route, V_endRow should be used.
Best,
Judy
June 9, 2024 at 08:45 am - Permalink