
Quaternion to Euler angle converter?
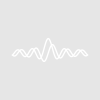
pmazzoni
I would like to write a conversion function from (unnormalized) quaternions to Euler angles representing a 3D rotation (from accelerometer data). Does anyone have such a routine already written and is willing to share it? I know I am being lazy but I am new to quaternions and my head is already spinning (pun intended).
I wrote this some time ago, maybe it is of some use?
https://quantixed.org/2019/10/08/rotation-using-quaternions-in-igor-pro/
October 7, 2023 at 01:57 pm - Permalink
Thank you! I was looking for the inverse of your function, to convert quaternions back to angles, but I see that it's not that difficult to write, and the explanations on your post were very helpful.
October 7, 2023 at 03:12 pm - Permalink
It is not clear how you want to actually use or calculate the Quat-->Euler transformation. There is a method which avoids all calculation; simply use Igor's built in Gizmo operations. If you setup a dummy Gizmo, then (as an example)
From this example, you should be able to write a simple wrapper function for the Quaternion input, that returns the global Euler angle outputs.
October 9, 2023 at 05:48 am - Permalink
First note that I do not recommend using Euler angles. There is a fair amount of literature that explains why using Euler angles is usually not a good idea.
However, if you need to convert from a quaternion to Euler angles and you have decided which formats you are using I'd get there in two steps:
1. Convert your quaternion to a rotation matrix. This is described e.g., here: https://automaticaddison.com/how-to-convert-a-quaternion-to-a-rotation-…
2. Convert the rotation matrix to Euler angles. This is described e.g., here:
http://eecs.qmul.ac.uk/~gslabaugh/publications/euler.pdf
Also, check out MatrixOP functions for quaternions: quatToEuler(qIn,mode) and quatToMatrix(qIn).
October 9, 2023 at 05:07 pm - Permalink
Thank you both to s.r.chinn and Igor.
s.r.chinn: I was not familiar with the capabilities of gizmo that you pointed out. Those are exactly the operations I was looking for. Thank you!
Igor: I think I am aware of the reasons not to use Euler angles in general. My purpose is to extract quaternion data into a form that I can use to create a plot. The links you provided will help me better understand quaternions and decide whether my intuition about how to visualize the rotations they represent is incorrect. Thanks!
October 18, 2023 at 07:58 pm - Permalink
I am getting back to this task after learning more about quaternions and gizmo. I am trying to implement the suggestion by s.r.chinn to use Gizmo's built-in rotation function,
I am unclear about (at least) two steps. Here is what I'd like to do.
I have a 3-column matrix time series, Accelerometer, of acceleration obtained from a wearable sensor. The columns are for Sensor(x, y, z), meaning the sensor's coordinate frame. There are about 200,000-500,000 rows (30-60 minutes of recording at 128 Hz). The sensor is strapped to the chest at some arbitrary angle.
I'd like to obtain a new Accelerometer_Rot time series that is a rotated version of Accelerometer, such that the columns of Accelerometer_Rot are aligned with x, y, z in external space. I'd like to apply arbitrary rotations around the Sensor-x, y, z axes and visualize the resulting Accelerometer_Rot wave so I can determine the desired rotation by visual inspection.
Based on s.r.chinn's suggestion, I generated the following code:
•newGizmo •AppendToGizmo Accelerometer,name=path0 •ModifyGizmo displayLastObject •AppendToGizmo axes=defaultAxes,name=axes0 •ModifyGizmo showAxisCue=1
The example commands that rotate the path, suggested in the Igor manual p II-428, are
My questions start here:
1. what is the difference between the setQuaternion and the appendRotation commands?
2. I understood quaternions to contain {real, imaginary-x, imaginary-y, imaginary-z} components. Thus the appendrotation command above says, "Rotate around the y axis by pi/2 and around the z axis by pi/2". Is this correct?
3. Then what rotation is specified in the setQuaternion command above? What axis is intended by the first element specified?
4. After I have applied the rotation, I would like to recover the rotated 3-column wave. How do I access the resulting rotated wave? If I execute
I get S_ObjectNames, which contains: path0;axes0;
Is "path0" the rotated time series? How do I access it?
September 21, 2024 at 04:19 pm - Permalink
Update:
I got this to work. The gizmo approach was not a good match for what I wanted. Instead, I programmed all necessary calculations based on the clear tutorial I found here:
https://danceswithcode.net/engineeringnotes/quaternions/quaternions.html
Here is the code in case it's of use to anyone else. The first function takes a point XYZ, a rotation angle, and a rotation axis, and creates a rotated version of that point. The second function takes a time series of XYZ points and calls the first function for each row, and generates a rotated version of the 3D time series.
September 30, 2024 at 03:06 pm - Permalink