
Read Patient Header and Data From the Same File
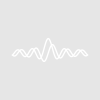
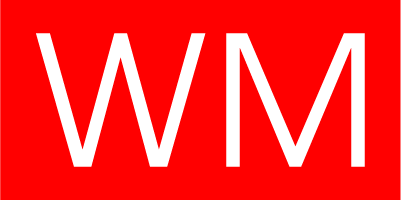
hrodstein
This snippet is a solution for an Igor user who wanted to use header information to construct the name of a wave to be loaded from a data file. This solution uses the technique of storing the header information in a list of keyword-value pairs and later querying that list for specific fields.
For background information and links to other solutions, see https://www.wavemetrics.com/code-snippet/reading-header-and-data-same-f…
#pragma TextEncoding = "UTF-8" #pragma rtGlobals=3 // Use modern global access method and strict wave access #pragma DefaultTab={3,20,4} // Set default tab width in Igor Pro 9 and later // Reads header information and numeric data from a text file with a format like this: // Patient Data // Patient Group: 33 // Subject ID: ABCD 99W // Location ID: XYZ 22X // Record Number: 55 // // Variables // Level = 80 // AudFreq = 4000 // Fade = 2.5 // Gain = 1.00 // // Data: // 123.456 // 234.567 // 345.678 // The user wanted to use the values of the "Subject ID" field and the Level and AudFreq // variables to form the name of the resulting wave. // // To understand this file it is best to read it from the bottom (high-level) up. // GetFieldNameAndValueStrFromHeaderLine(headerLine, fieldName, valueStr) // headerLine is a possible line of header from the file. // In this example, the header can contain fields and variables. For example: // Subject ID: ABCD 99W // Subject ID is a field // Level = 80 // Level is a variable // For our purposes, we treat both as header fields and require no conflicts // between header field names and header variable names. static Function GetFieldNameAndValueStrFromHeaderLine(headerLine, fieldName, valueStr) String headerLine // Input String& fieldName // Output String& valueStr // Output int lineLength = strlen(headerLine) // Header lines start with either "<Field Name>: " or "<Var Name> = " int isVarName = 0 int pos = strsearch(headerLine, ": ", 0) if (pos < 0) // Did not find <Field Name>: " pos = strsearch(headerLine, " = ", 0) if (pos < 0) // Did not find <Var Name> = " return -1 // Failure endif isVarName = 1 endif fieldName = headerLine[0,pos-1] if (isVarName) valueStr = headerLine[pos+3,lineLength-2] else valueStr = headerLine[pos+2,lineLength-2] endif return 0 // Success End // ReadHeaderInfo(pathName, fileName, maxHeaderLines) // Returns a list of field names and values as colon-separated keyword-value pairs. static Function/S ReadHeaderInfo(pathName, fileName, maxHeaderLines) String pathName // Name of symbolic path. Ignored if filePath is full path. String fileName // File name, relative path or full path int maxHeaderLines // Maximum lines of header at start of file Variable refNum Open/R/P=$pathName refNum as fileName if (refNum == 0) // File not opened - bad pathName or fileName return "" endif String resultStr = "" int lineNumber = 0 do String line FReadLine refNum, line if (strlen(line) == 0) break // No more text in file endif String fieldName // e.g., "Subject ID" or "Level" String valueStr // e.g., "ABCD 99W" or "80" if (GetFieldNameAndValueStrFromHeaderLine(line, fieldName, valueStr) != 0) // No field on this line lineNumber += 1 continue endif resultStr += fieldName + ":" + valueStr + ";" lineNumber += 1 if (lineNumber >= maxHeaderLines) // There are no more header lines to examine break endif while(1) Close refNum return resultStr End // DemoReadPatientHeaderAndData(pathName, fileName) // Demonstrates reading header information and data from a file like the "Patient Sample Data.txt" file. // In this case, the file contains about 30 header lines followed by one column // of numeric data. The user wants to use certain header fields to form the wave name. // // To display an Open File dialog, execute: // DemoReadPatientHeaderAndData("", "") // // To load a file without an Open File dialog dialog, execute: // Create a symbolic path named "PatientData" and then execute // DemoReadPatientHeaderAndData("PatientData", "Patient Sample Data.txt") // If you are not familiar with Igor's "symbolic path" concept, execute this: // DisplayHelpTopic "Symbolic Paths" // // If the wave already exists, this routine overwrites it. Function DemoReadPatientHeaderAndData(pathName, fileName) String pathName // Name of an Igor symbolic path or "" to get an Open File dialog String fileName // Name of file or full path to file or "" to get an Open File dialog // If necessary, display an Open File dialog to get a valid reference to a file if ((strlen(pathName)==0) || (strlen(fileName)==0)) // Display dialog looking for file Variable refNum String fileFilters = "Data Files (*.txt,*.dat,*.csv):.txt,.dat,.csv;" fileFilters += "All Files:.*;" Open /D /R /F=fileFilters /P=$pathName refNum as fileName fileName = S_fileName // S_fileName is set by Open/D if (strlen(fileName) == 0) // User cancelled? return -1 endif endif // Read header information String headerInfo = ReadHeaderInfo(pathName, fileName, 100) String subjectIDStr = StringByKey("Subject ID", headerInfo) double AudFreq = NumberByKey("AudFreq", headerInfo) double Level = NumberByKey("Level", headerInfo) // Printf "subjectID=%s, AudFreq=%g, Level=%g\r", subjectIDStr, AudFreq, Level // For debugging only // Remove spaces which complicate wave names subjectIDStr = ReplaceString(" ", subjectIDStr, "") // Create a standard name because liberal names create complications String name sprintf name, "%s_%d_%d", subjectIDStr, AudFreq, Level // e.g., "ABCD99W_4000_80 name = CleanupName(name, 0) // Load wave String columnInfoStr = "N=" + name + ";" LoadWave/G/P=$pathName/A/O/B=columnInfoStr/Q fileName if (V_Flag != 1) // We expect 1 wave to be created return -1 // Failure endif // Create wave reference WAVE w = $name // Store header information in wave note String noteText = ReplaceString(";", headerInfo, "\r") // Each field on separate line Note w, noteText return 0 // Success End
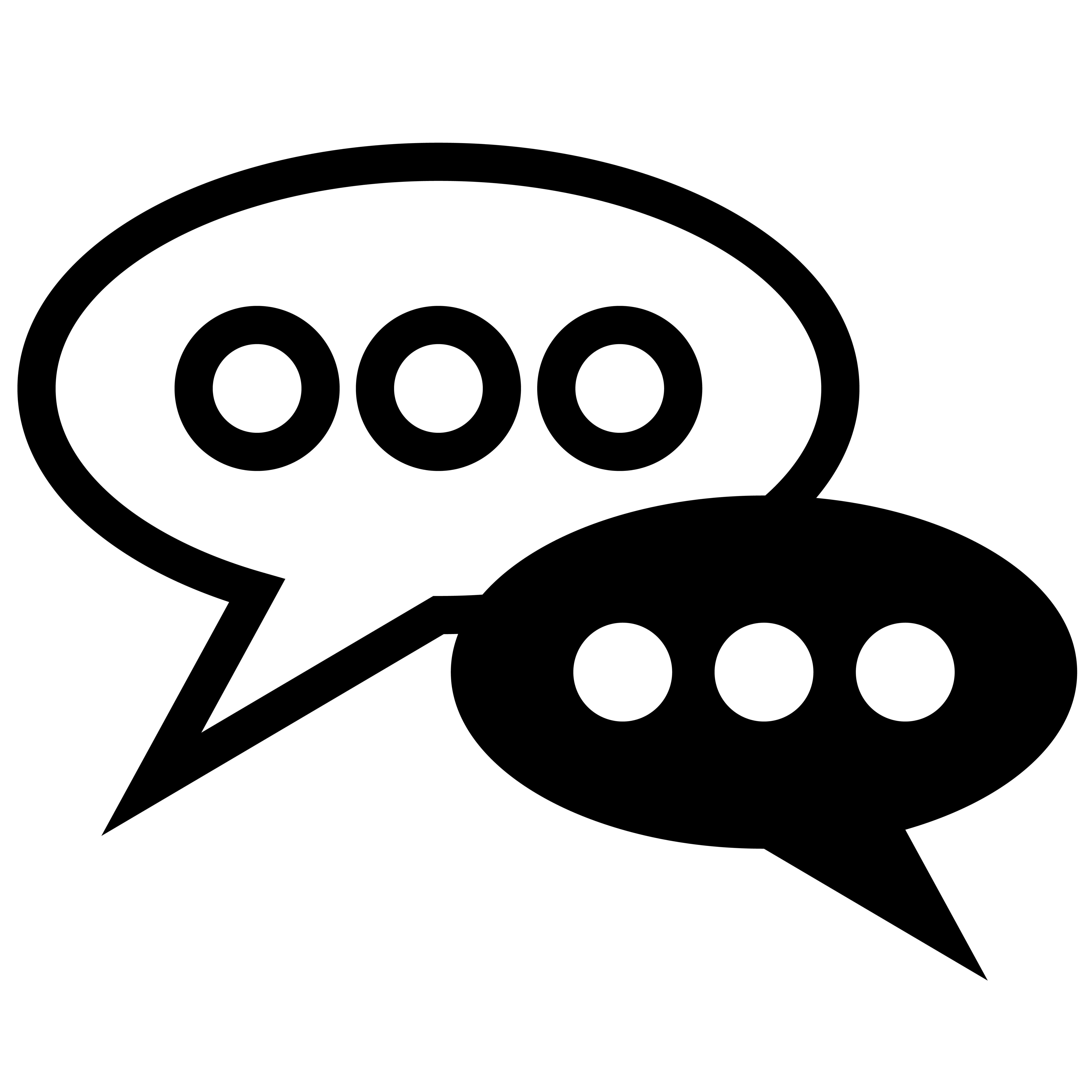
Forum
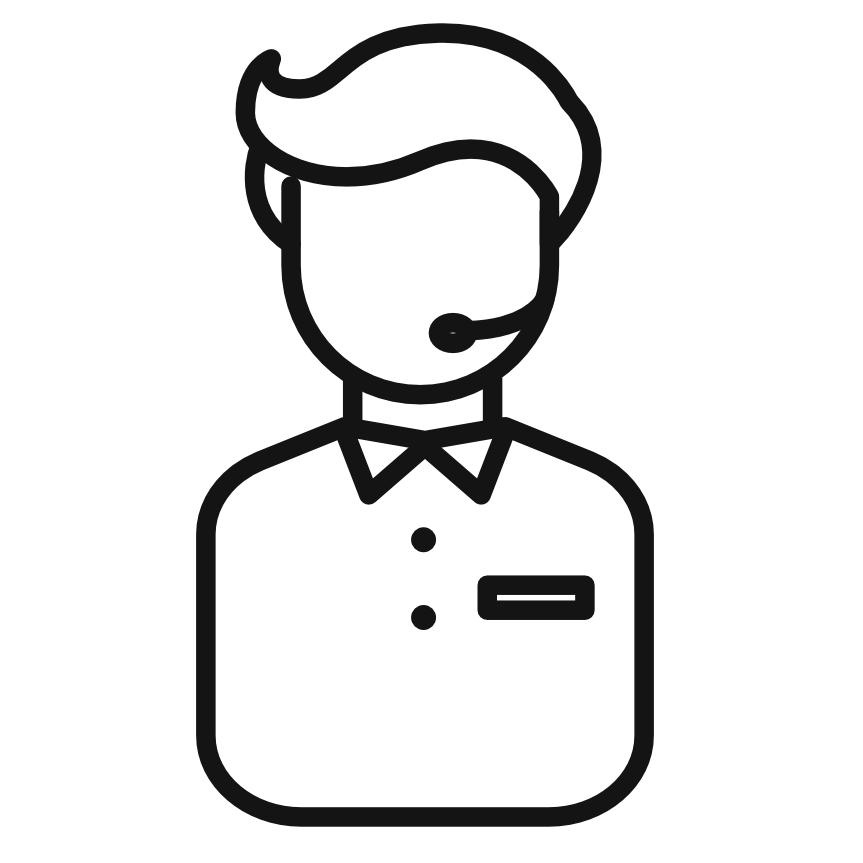
Support
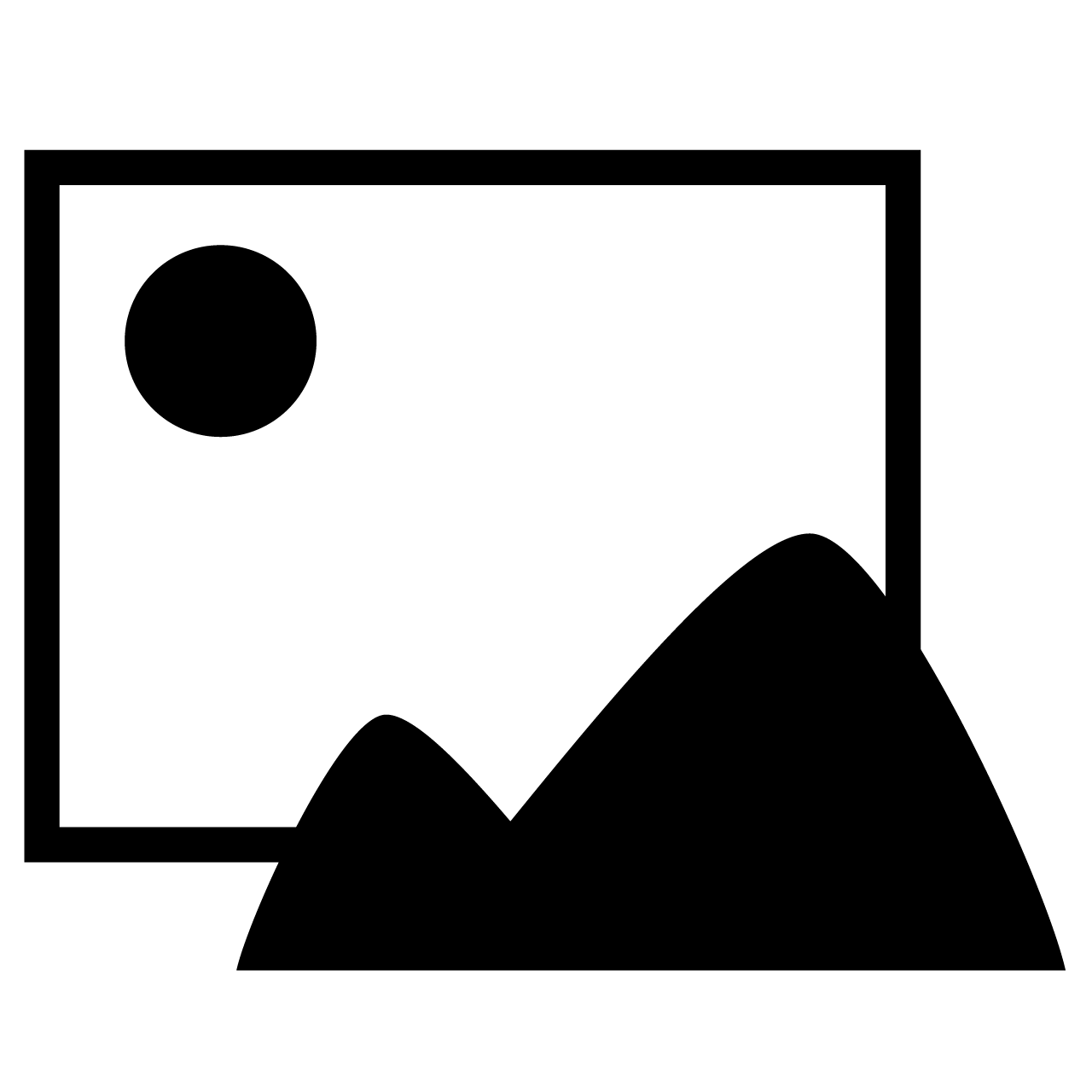
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More
Sample patient data file.
September 13, 2021 at 01:30 pm - Permalink