
opening a file algorithmically
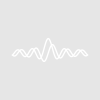
gordon.shaw@nist.gov
I am trying to open files algorithmically in an igor procedure. My approach is to generate a text wave containing all the filenames and then to use a while loop to get the file path from the wave, open the file, perform the analysis, and then move on to the next loop iteration.
Here's what I have so far that doesn't work:
Function IndAn() wave filenamewave //This is the text wave with the file paths in it variable odex=0 do string tempnamestring=filenamewave[3] //try to make a string that I can use in the line below LoadWave /D /E=0 /G /H /K=0 /L={112,113,0,0,0} /O /W/A tempnamestring odex+=1 while (odex<(numpnts(filenamewave)) end
I've also tried using "filenamewave[3]" directly in the LoadWave command. In both cases I get the error:
got "filenamewave" instead of a string variable or string function name.
Any advice on the easiest way for me to pull out a string from a wave and use it in a command this way?
If indeed filenamewave is a text wave then you need to have:
Wave/T filenamewave //This is the text wave with the file paths in it
The /T flag tells the program the correct format. Then you can assign a certain entry of this wave to a string, but I guess you rather wanted to write this:
string tempnamestring=filenamewave[odex] //try to make a string that I can use in the line below
By the way, be careful with do-while loops, since they can easily soft-lock Igor if you have a mistake and the loop runs forever. This is a classic case where you rather want to use a for-loop. do-while loops are useful in cases where the number of iterations are not fixed from the start.
July 30, 2021 at 12:33 pm - Permalink
You need to declare the string outside the loop and give it a value inside the loop. I also added your counter to the filenamewave
Have you checked that your LoadWave line works on a single file?
Just for inspiration here is an example where the user selects the waves to be loaded
July 30, 2021 at 12:48 pm - Permalink