
Print Folders and Files Recursively
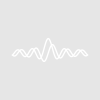
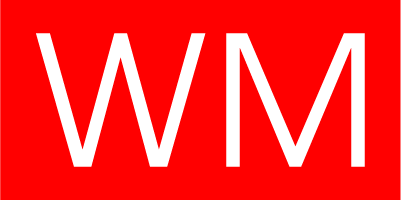
hrodstein
// PrintFoldersAndFiles(pathName, extension, recurse, level) // Shows how to recursively find all files in a folder and subfolders. // pathName is the name of an Igor symbolic path that you created using NewPath or the Misc->New Path menu item. // extension is a file name extension like ".txt" or "????" for all files. // recurse is 1 to recurse or 0 to list just the top-level folder. // level is the recursion level - pass 0 when calling PrintFoldersAndFiles. Function PrintFoldersAndFiles(pathName, extension, recurse, level) String pathName // Name of symbolic path in which to look for folders and files. String extension // File name extension (e.g., ".txt") or "????" for all files. Variable recurse // True to recurse (do it for subfolders too). Variable level // Recursion level. Pass 0 for the top level. Variable folderIndex, fileIndex String prefix // Build a prefix (a number of tabs to indicate the folder level by indentation) prefix = "" folderIndex = 0 do if (folderIndex >= level) break endif prefix += "\t" // Indent one more tab folderIndex += 1 while(1) // Print folder String path PathInfo $pathName // Sets S_path path = S_path Printf "%s%s\r", prefix, path // Print files fileIndex = 0 do String fileName fileName = IndexedFile($pathName, fileIndex, extension) if (strlen(fileName) == 0) break endif Printf "%s\t%s%s\r", prefix, path, fileName fileIndex += 1 while(1) if (recurse) // Do we want to go into subfolder? folderIndex = 0 do path = IndexedDir($pathName, folderIndex, 1) if (strlen(path) == 0) break // No more folders endif String subFolderPathName = "tempPrintFoldersPath_" + num2istr(level+1) // Now we get the path to the new parent folder String subFolderPath subFolderPath = path NewPath/Q/O $subFolderPathName, subFolderPath PrintFoldersAndFiles(subFolderPathName, extension, recurse, level+1) KillPath/Z $subFolderPathName folderIndex += 1 while(1) endif End
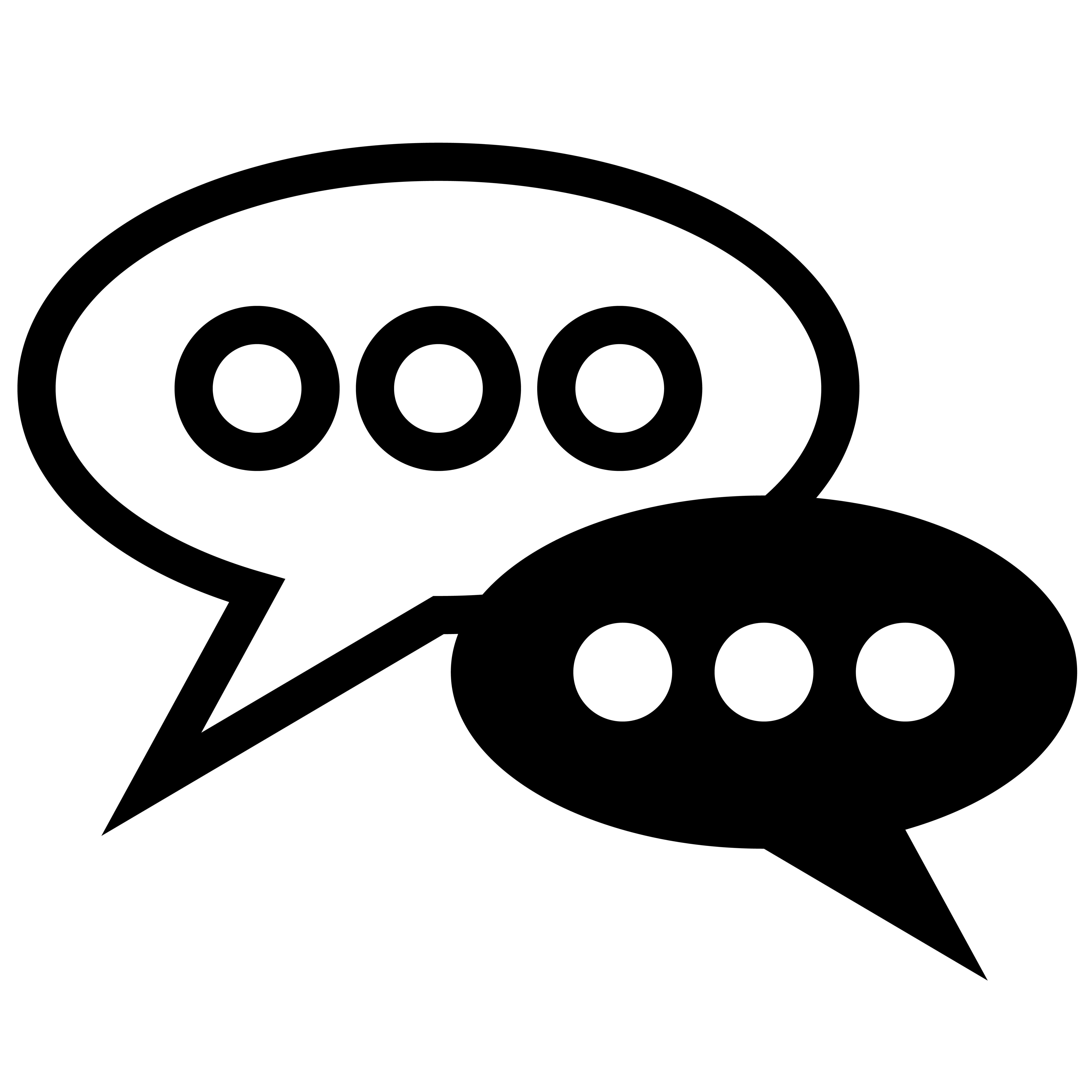
Forum
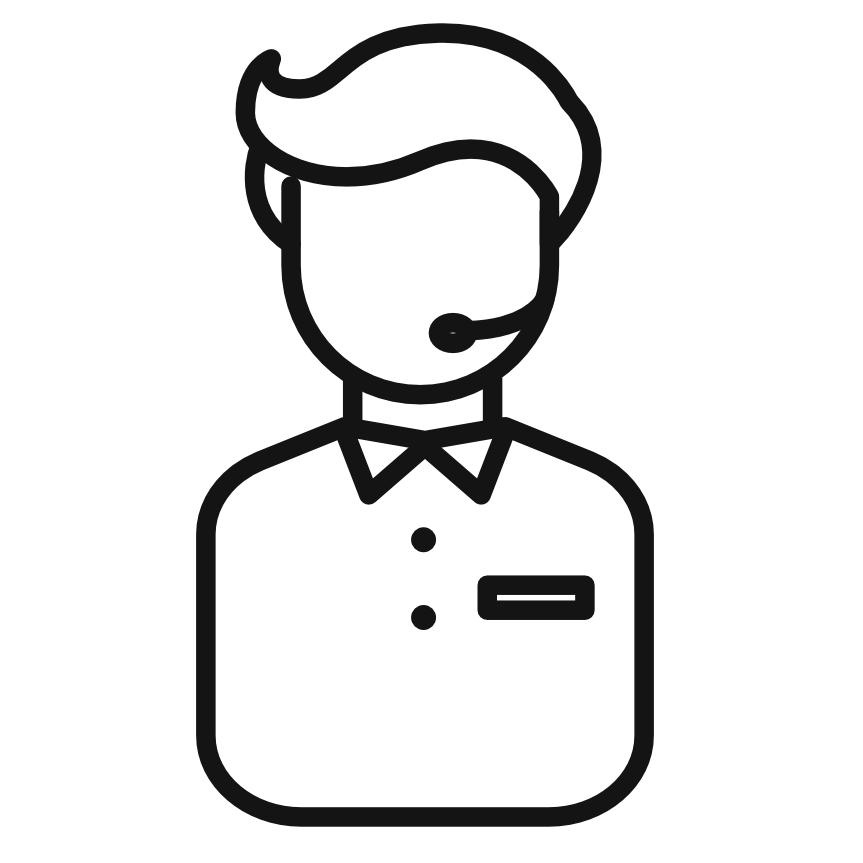
Support
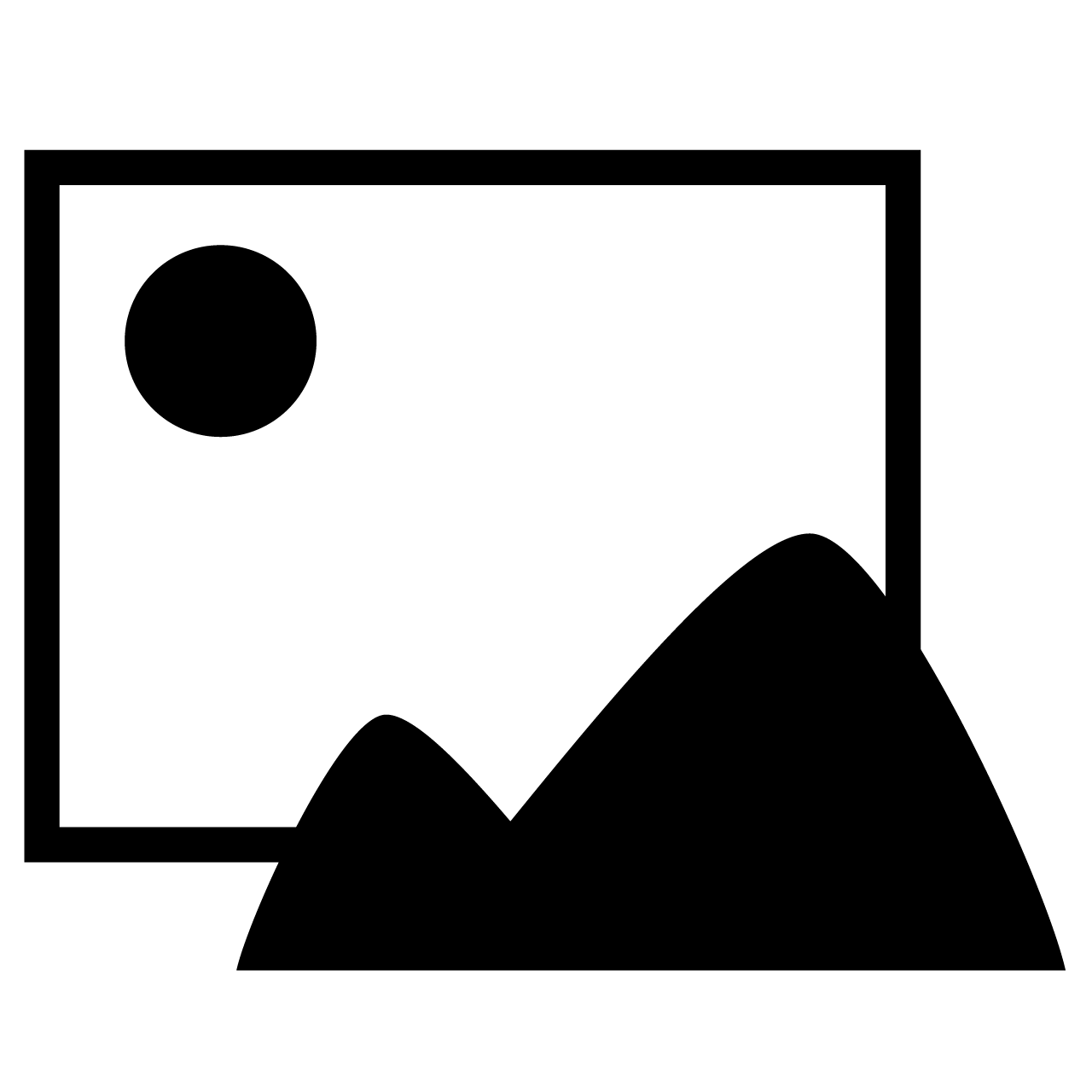
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More
Hi
I'm trying to use the procedure above to load excel files from different subdirectories from my PC. However, I cannot place a running index somewhere in the code such that it increases by one for each file that's loaded. How can I do that?
Cheers
Silvan
October 27, 2020 at 08:18 am - Permalink
If I understand your question, the only simple way to do this is to create a global variable initialized to 0 in your top-level function and increment it in PrintFoldersAndFiles where the fileIndex variable is incremented.
A cleaner but more complicated solution is to add a pass-by-reference parameter and increment it in PrintFoldersAndFiles where the fileIndex variable is incremented. This is cleaner because it avoids the use of a global variable.
For details on pass-by-reference parameters, execute:
DisplayHelpTopic "Pass-By-Reference"
October 27, 2020 at 10:23 am - Permalink