
Check 1D wave for non-zero values and count them
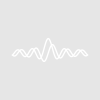
Physicist92
Hi all,
Could you please tell me, how to check rows of matrix for non-zero values? For example, 0th row consists only of zeros, 1st row also consists only of zeros, 2nd row constists of zeros and non-zeros, and so on. How to implement the following:
for (i=0; i<512; i+=1)
if (i-th row consists only of zeros)
continue
elseif (i-th row contains non-zero values)
count number of non-zero values in this row
endfor
Seems like MatrixOP sumRows would result in a zero for any row that is all zeroes.
July 23, 2019 at 05:10 pm - Permalink
In reply to Seems like MatrixOP sumRows… by johnweeks
and any other row that sums to zero...
if there is a possibility of that happening, sum(abs()) is more robust
July 24, 2019 at 02:03 am - Permalink
For integer waves you could use:
for floating point waves you could use some tolerance:
Variable tol=1e-15
A.G.
July 24, 2019 at 11:28 am - Permalink
johnweeks, tony, Igor, thank you!
July 24, 2019 at 11:50 am - Permalink
I have an image that is (512,512) matrix, and wave scaling (in millimeters) is applied to this matrix along X and Y axes.
How to extract i-th row from scaled 2D wave?
For example, if I try to extract 2nd row:
print image1[2][]
it doesn't work (Igor says: Syntax Error - expected operand).
But if I write:
print image1[2][510]
i.e. specifying the column number, Igor prints the corresponding value. But I need to extract the entire row.
July 25, 2019 at 12:50 pm - Permalink
Try:
MatrixOP/O myRow=row(image1,rowNumber)
July 25, 2019 at 01:12 pm - Permalink
In reply to Try: MatrixOP/O myRow… by Igor
Thank you!
Another variant that I found on this forum (https://www.wavemetrics.com/forum/general/extracting-data-2d-wave) is:
Duplicate/O/RMD=[2][] image1,tmp
This variant preserves scaling.
I also have a question about 1D wave filling. Is it possible to create empty wave and then fill it with numbers on every step in loop (i.e. append numbers to the list like in Python)? I need empty wave because I don't know in advance what size of wave is necessary.
July 25, 2019 at 01:43 pm - Permalink
Please note that both variants produce a 2D wave that contains 1 row by N columns. If this is a problem you can add ^t to the MatrixOP command or use Redimension in the other approach.
July 25, 2019 at 02:28 pm - Permalink
Variable npnts = numpnts(wave0)
Variable i
for (i = 0; i < npnts; i++)
wave0[i] = sqrt(i)
endfor
This particular use of the loop is not efficient; anytime you want to fill a wave with values it is more efficient to use a wave assignment statement like this:
wave0=sqrt(p)
But my loop shows you how to put values into each point of a wave inside a loop.
Be sure to read about wave assignments: DisplayHelpTopic "Waveform Arithmetic and Assignment"
July 25, 2019 at 05:17 pm - Permalink
Igor, johnweeks, thank you!
July 26, 2019 at 04:34 am - Permalink
In reply to WAVE wave0 Variable npnts =… by johnweeks
Probably you misunderstood me. I meant that I'd like to create wave without any elements. And then, in for-loop, depending on fulfilling if-conditions, I'd like to fill the wave step by step. I tried to use
test1[0]=10
The first command creates empty wave, but I can't work with this wave further - second command doesn't work, IgorPro says: "Command Error, the wave has zero data allocated". So it appears that it is mandatory to allocate space for the wave. But what if I want to create totally empty wave and then gradually fill it with numbers? Is it possible? I've read "Waveform Arithmetic and Assignment" but I didn't find answer.
July 26, 2019 at 05:59 am - Permalink
In reply to johnweeks wrote: WAVE… by Physicist92
try test1[0]={10}
July 26, 2019 at 06:02 am - Permalink
In reply to Physicist92 wrote: make… by tony
It works! Thank you!
July 26, 2019 at 06:05 am - Permalink
In reply to tony wrote: … by Physicist92
A useful shorthand for adding a point to a wave and assigning its value is
wave[numpnts(wave)]={newpointvalue}
July 26, 2019 at 06:08 am - Permalink
Please be aware that inserting points one at a time is quite inefficient, as it requires re-allocating the memory for the wave each time. It is better if you can figure out the number of points you need ahead of time. I realize that it can be quite difficult to do that, though.
If you only need a few points to a few hundred points, the inefficiency may not be important.
July 26, 2019 at 10:31 am - Permalink
tony, johnweeks, thank you!
johnweeks,
I need few hundred points.
July 26, 2019 at 10:55 am - Permalink
To follow up on John's note about adding points ... you could create a wave that has the maximum number of points in it that you think you will ever need, set it completely to NaN, and then fill sequentially with 1 or 0 as needed.
July 26, 2019 at 12:02 pm - Permalink
In reply to To follow up on John's note… by jjweimer
Thank you!
July 26, 2019 at 01:23 pm - Permalink