
String2Pict and Pict2String functions
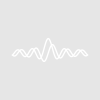
patg
//==================================================================================================================================== // stringToPICT // ------------ // * SUMMARY: 'stringToPICT' turns a string containing picture file data into a global picture, returning a name for the picture // // * DETAILS: The string is written out to a temporary file and reloaded a picture, overwriting an existing picture if requested. // // * ARGUMENTS: // - iStr: string of the input. Use 'svStr' if calling from a function to save the overhead of copying the string, // in which case the 'iStr' parameter is ignored. // // - [svStr]: Optional string reference. If supplied, 'iStr' is ignored, and input taken from this string reference. // // - [pictName]: Optional picture name as a string. If supplied this name will be used for the picture, overwriting any // existing picture of that name. If missing a name of the form 'PICT_n' (n=0,1,...) will be generated. // // - [errMsg]: Optional pass-by-reference string to receive error message. If present the function will not abort. // // * RETURNS: Picture name as a string. // // * ON ERROR: If 'errMsg' is passed: // - for no error, sets 'errMsg'="", returns 0; // - for error, sets 'errMsg'=<error string>, returns V_abortCode. Does not abort. // If 'errMsg' is not a parameter: returns 0 for no error, otherwise aborts with error message, prints the error to // history, and Sets the global string S_Error and V_Error to the error string and V_AbortCode. // // * SOURCE: Patrick Groenestein, 2009 // // * UPDATES: 20090512 Created. // //------------------------------+---------------------------------------------------------------+------------------------------------- static FUNCTION/S string2PICT(iStr [,svStr,pictName,errMsg]) string iStr,&svStr,pictName,&errMsg; variable err=0,i,n,isSV,refNum; string eMsg="",oStr="",file="",uStr=""; string tmpPath=specialDirPath("Temporary",0,0,0); if( !paramIsDefault(errMsg)) errMsg = ""; endif; TRY isSV = !paramIsDefault(svStr); if( !(strlen(tmpPath)>0)) tmpPath = specialDirPath("DeskTop",0,0,0); abortOnValue !(strlen(tmpPath)>0),2; endif; for(i=0 ; ; i+=1) file = tmpPath+"QQF_S2PTmp_"+num2iStr(i); getFileFolderInfo/Q/Z file; if(V_flag!=0) break; endif; endfor; if(strlen(file)) open/T="????"/Z refNum as file; abortOnValue V_Flag!=0,2; if(isSV) fBinWrite refnum,svStr; abortOnRTE; else fBinWrite refnum,iStr; abortOnRTE; iStr = ""; endif; close refNum; refNum = 0; if(paramIsDefault(pictName)) for(i=0 ; ; i+=1) pictName = "PICT_"+num2iStr(i); if( !strlen(PictInfo(pictName))) break; endif; endfor; endif; loadPICT/O/Q file,$pictName; abortOnRTE; deleteFile file; abortONRTE; file = ""; oStr = pictName; endif; abortOnRTE; CATCH variable ev; string es=""; if(V_AbortCode<0) es = getRTErrMessage(); ev = getRTError(1); elseif(V_AbortCode>0) es = "No error. [BUG]\n"; // V_AbortCode 0, not used es += replaceString("\n",eMsg,"<LF>",0,0)+"\n"; // V_AbortCode 1 es += "Cannot write files on this system.\n"; // V_AbortCode 2 es += "<Undocumented error> [BUG]\n"; // V_AbortCode <undocumented> ev = min(V_AbortCode,itemsInList(es,"\n")-1); es = stringFromList(ev,es,"\n"); endif; ENDTRY; // Add cleanup here if(refNum) close refNum; endif; if(strlen(file)) getFileFolderInfo/Q/Z file; if(V_Flag==0) deleteFile/Z file; endif; endif; if(V_AbortCode) es[0] = getRTStackInfo(1)+" error <"+num2iStr(V_AbortCode)+">: "; if(paramIsDefault(errMsg)) variable/G V_Error=V_AbortCode; string/G S_Error=es; print es; abort es; else errMsg = es; endif; endif; return(oStr); END //==================================================================================================================================== // PICT2String // ------------ // * SUMMARY: 'PICT2String' turns a picture into a string containing picture file data. // // * DETAILS: The picture is written out to a temporary file and reloaded as a string. // // * ARGUMENTS: // - pictName: name of the picture, as a string. // // - [errMsg]: Optional pass-by-reference string to receive error message. If present the function will not abort. // // * RETURNS: string result, filled with the picture data. // // * ON ERROR: If 'errMsg' is passed: // - for no error, sets 'errMsg'="", returns 0; // - for error, sets 'errMsg'=<error string>, returns V_abortCode. Does not abort. // If 'errMsg' is not a parameter: returns 0 for no error, otherwise aborts with error message, prints the error to // history, and Sets the global string S_Error and V_Error to the error string and V_AbortCode. // // * SOURCE: Patrick Groenestein, 2009 // // * UPDATES: 20090512 Created. // //------------------------------+---------------------------------------------------------------+------------------------------------- static FUNCTION/S PICT2String(pictName [,errMsg]) string pictName,&errMsg; variable err=0,i,n,refNum; string eMsg="",oStr="",file="",uStr="",suff="",type=""; string tmpPath=specialDirPath("Temporary",0,0,0); if( !paramIsDefault(errMsg)) errMsg = ""; endif; TRY if( !(strlen(tmpPath)>0)) tmpPath = specialDirPath("DeskTop",0,0,0); abortOnValue !(strlen(tmpPath)>0),2; endif; uStr = PICTInfo(pictName); abortOnValue !strlen(uStr),3; type = stringByKey("TYPE",uStr,":",";"); i = whichListItem(type,"Unknown type;PICT;PNG;JPEG;Enhanced metafile;Windows metafile;DIB;Windows bitmap;",";",0,0); i = i<0 ? 0 : i; suff = stringFromList(i,";.pct;.png;.jpg;.emf;.wmf;.dib;.bmp;",";"); for(i=0 ; ; i+=1) file = tmpPath+"QQF_P2STmp_"+num2iStr(i)+suff; getFileFolderInfo/Q/Z file; if(V_flag!=0) break; endif; endfor; if(strlen(file)) uStr = PICTInfo(pictName); abortOnValue !strlen(uStr),3; savePICT/PICT=$pictName as file; abortOnRTE; open/R/T="????" refnum as file; abortOnValue !strlen(S_FileName),4; fStatus refNum; abortOnValue !V_Flag,4; oStr = padString("",V_LogEOF,0); abortOnRTE; fBinRead refNum,oStr; abortOnRTE; close refNum; refNum = 0; deleteFile file; abortONRTE; file = ""; endif; abortOnRTE; CATCH variable ev; string es=""; if(V_AbortCode<0) es = getRTErrMessage(); ev = getRTError(1); elseif(V_AbortCode>0) es = "No error. [BUG]\n"; // V_AbortCode 0, not used es += replaceString("\n",eMsg,"<LF>",0,0)+"\n"; // V_AbortCode 1 es += "Cannot write files on this system.\n"; // V_AbortCode 2 es += "<"+pictName+"> is not a valid picture.\n"; // V_AbortCode 3 es += "Cannot read file <"+file+">.\n"; // V_AbortCode 4 es += "<Undocumented error> [BUG]\n"; // V_AbortCode <undocumented> ev = min(V_AbortCode,itemsInList(es,"\n")-1); es = stringFromList(ev,es,"\n"); endif; ENDTRY; // Add cleanup here if(refnum) close refnum; endif; if(strlen(file)) getFileFolderInfo/Q/Z file; if(V_Flag==0) deleteFile/Z file; endif; endif; if(V_AbortCode) es[0] = getRTStackInfo(1)+" error <"+num2iStr(V_AbortCode)+">: "; if(paramIsDefault(errMsg)) variable/G V_Error=V_AbortCode; string/G S_Error=es; print es; abort es; else errMsg = es; endif; endif; return(oStr); END
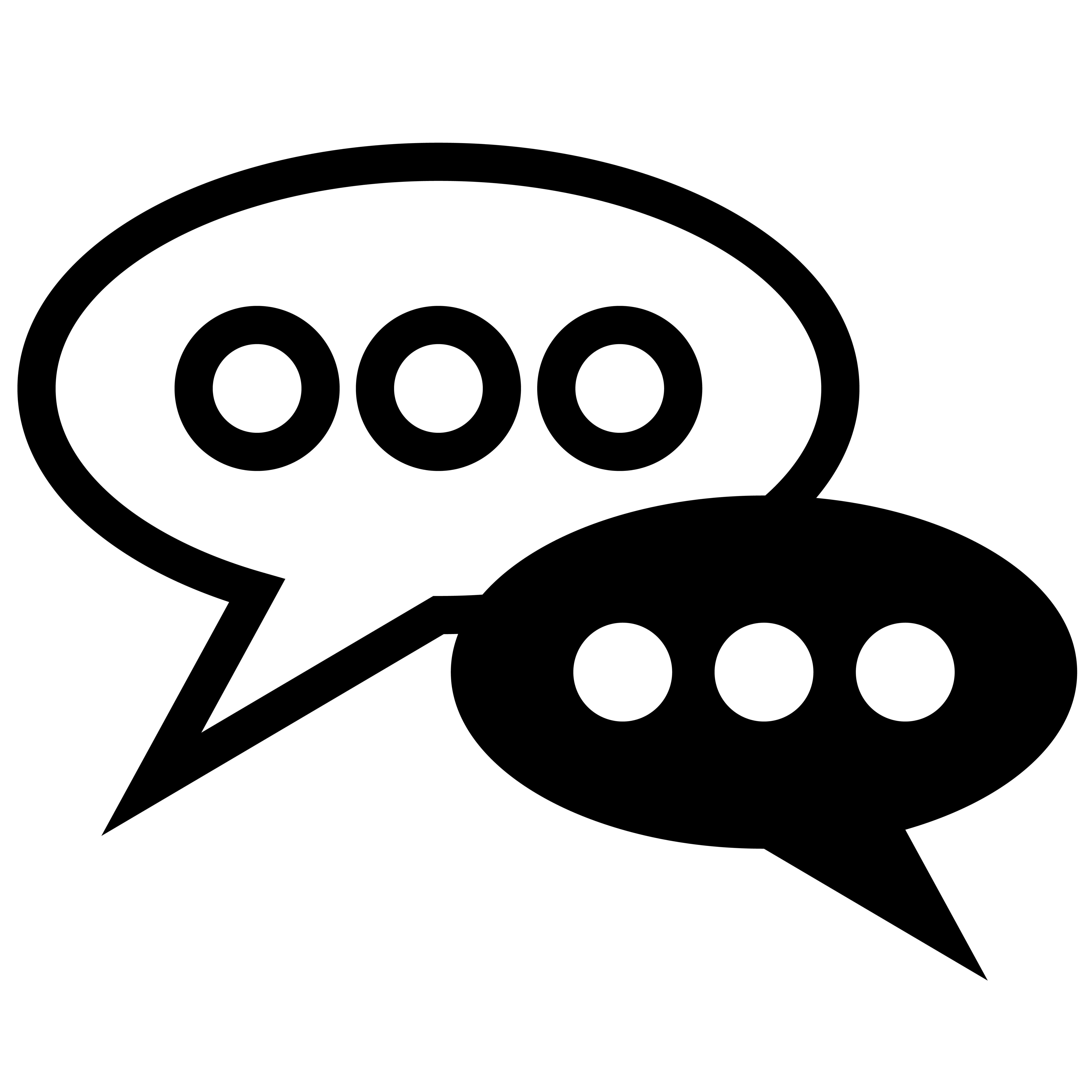
Forum
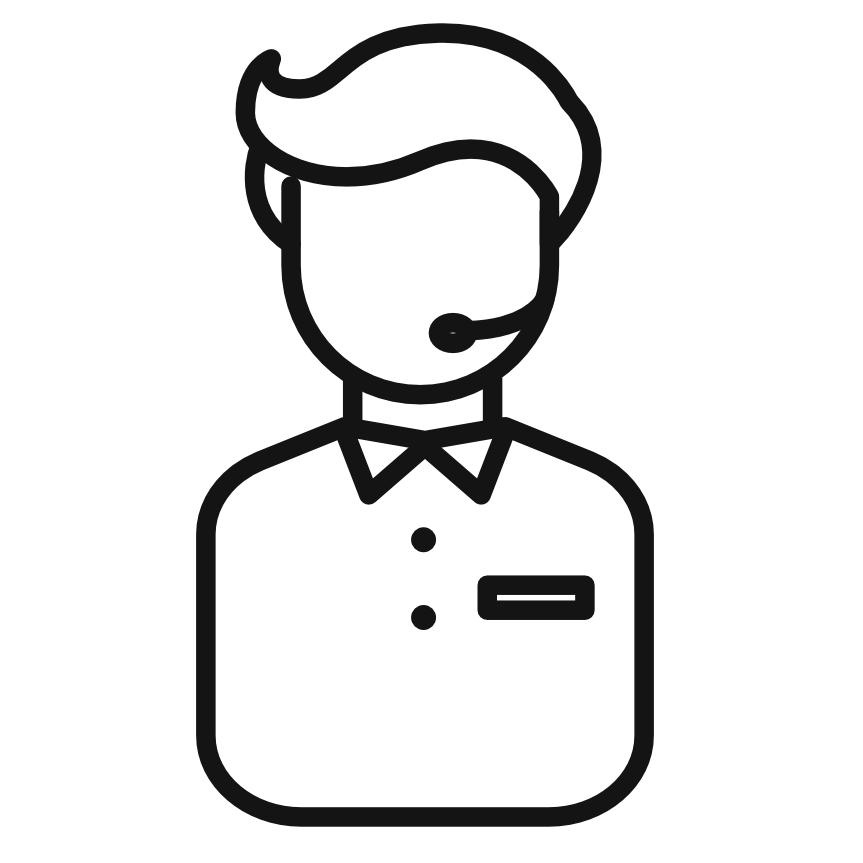
Support
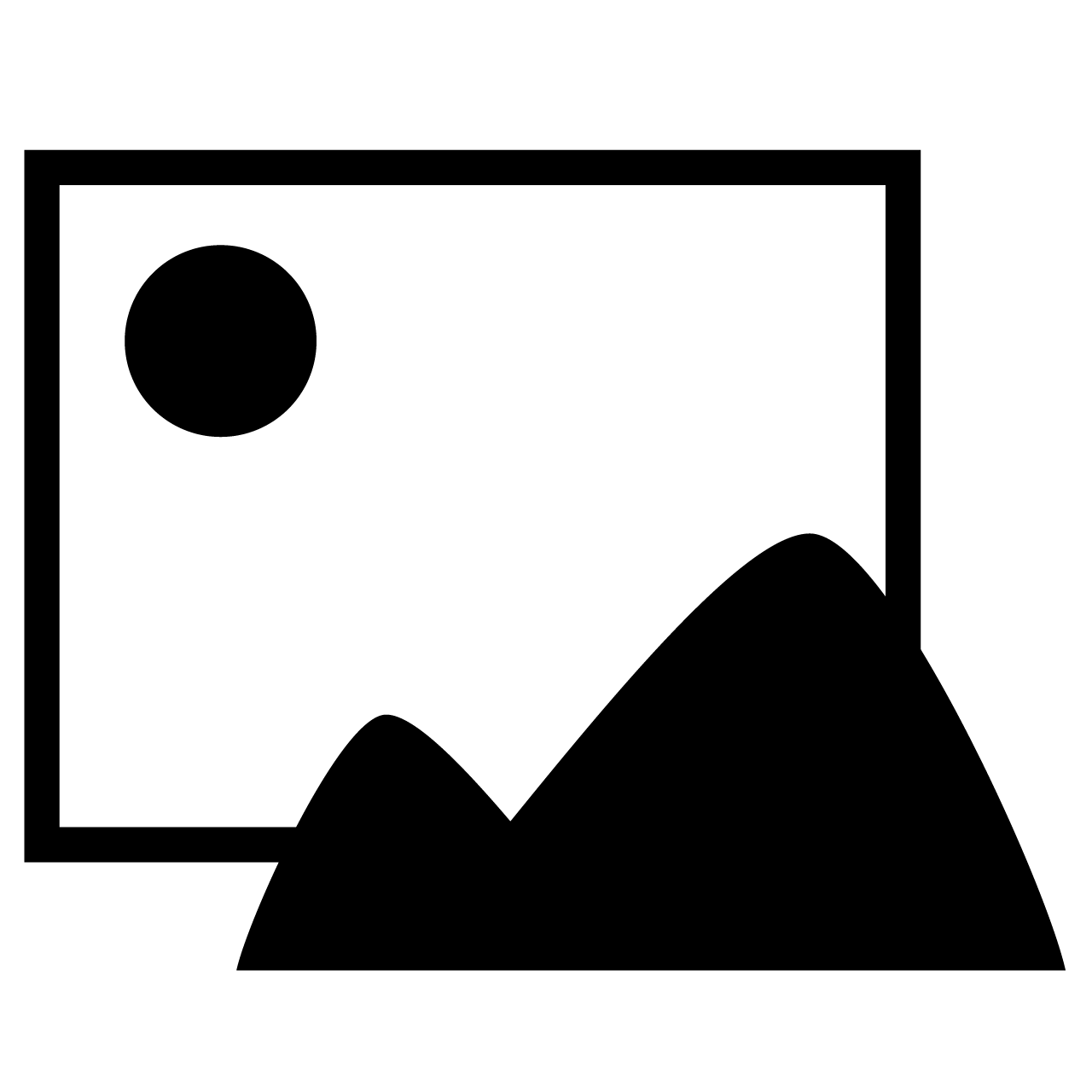
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More