
One way of sending mail from IGOR
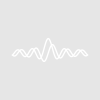
andyfaff
Here is some sample code that demonstrates using the SMTP LOGIN protocol (another option may be PLAIN, see http://www.technoids.org/saslmech.html#appA) :
string mailServer = "mail.mymailserver.com" string username = base64encode("myusername") string password = base64encode("mypassword") variable sock //holds the SOCKIT reference make/t buf //holds the output from the SOCKIT connection sock = sockitopenconnection(mailServer,25,buf,"","",1) //open a SOCKIT connection to the mail server sockitsendmsg(sock,"AUTH LOGIN\n") //SMTP LOGIN, NOT VERY SECURE sockitsendmsg(sock,username+"\n") sockitsendmsg(sock,password+"\n") sockitsendmsg(sock,"HELO whatever.whatever.com\n") //say HELO to the server sockitsendmsg(sock,"MAIL FROM: username@mymailserver.com\n") //say who you are sockitsendmsg(sock,"RCPT TO: poo@mymailserver.com\n") //say who the recipient is sockitsendmsg(sock,"DATA\n") //start the message sockitsendmsg(sock,"Subject:test a message\n\n") //subject line, note double newline is required sockitsendmsg(sock,"This is a test message\n") //the message sockitsendmsg(sock,".\n") //finish the message and send sockitcloseconnection(sock) //close the SOCKIT connection
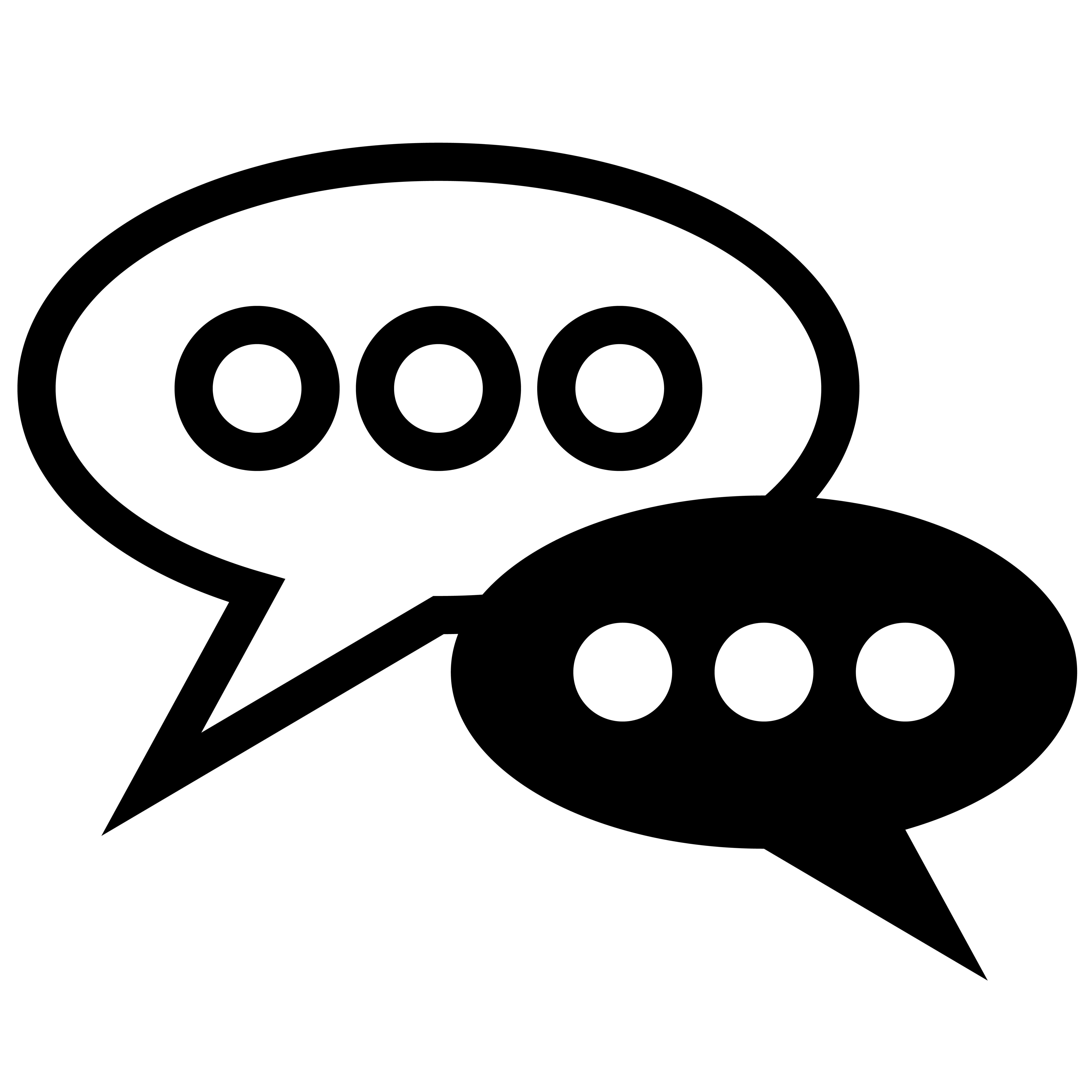
Forum
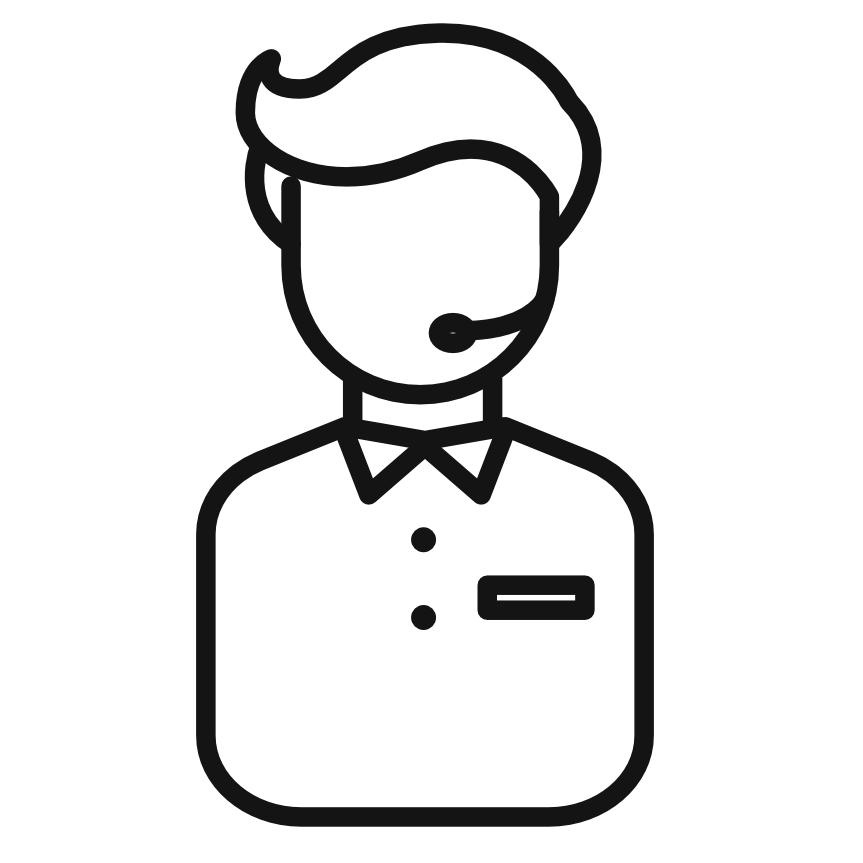
Support
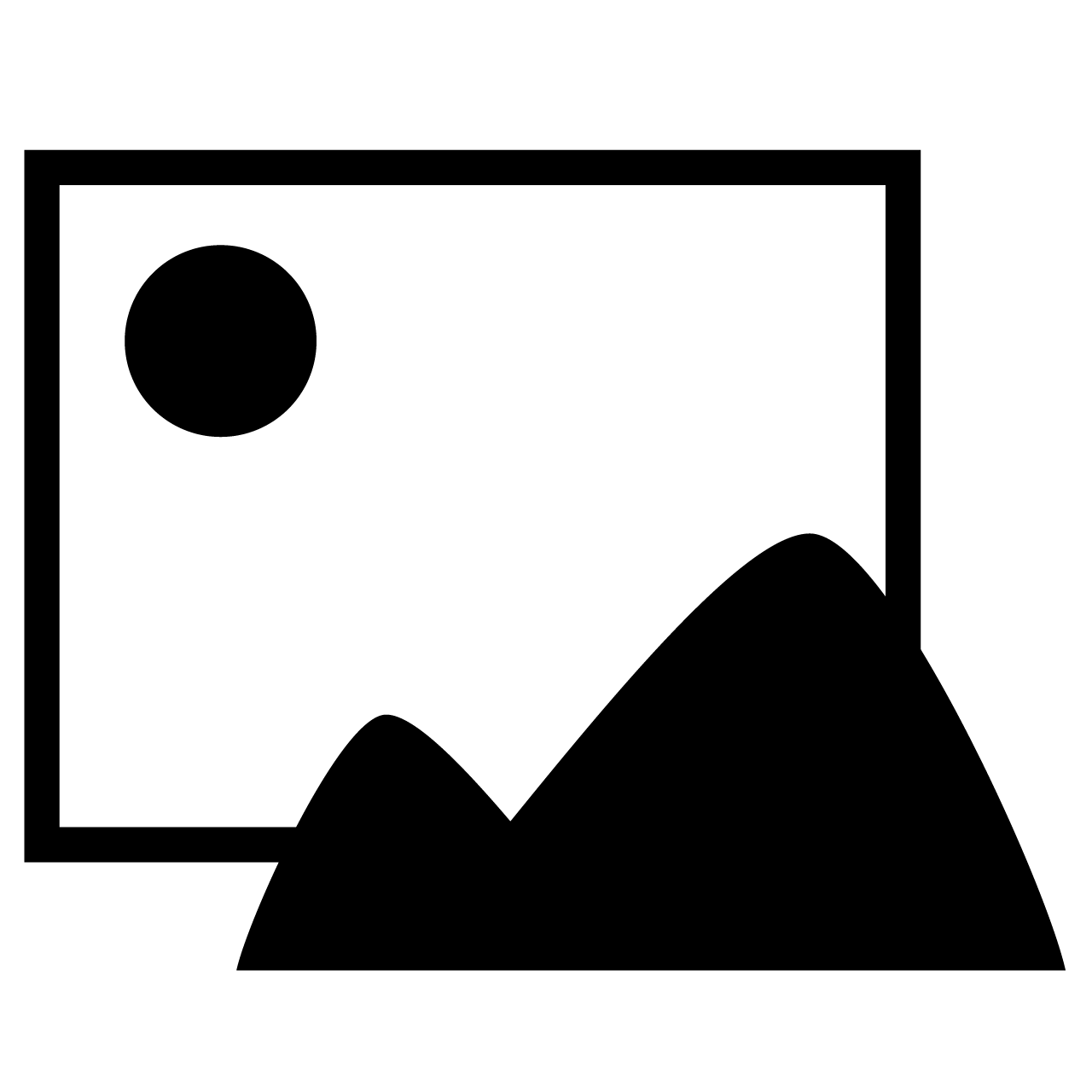
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More
For some reason, this only works without the /q flag in sockitiopenconnection. Maybe printing output slows Igor down enough to let messages get sent before the socket is closed?
August 2, 2010 at 11:15 am - Permalink
The only trouble is that the server may barf because the whole message arrives at once.
August 2, 2010 at 04:18 pm - Permalink