
Load Patchmaster 2X90 File
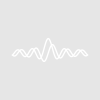
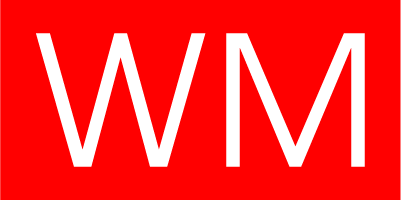
hrodstein
#pragma TextEncoding = "UTF-8" #pragma rtGlobals=3 // Use modern global access method and strict wave access. // Procedures to load a Patchmaster 2X90 file. // // The file format is unconventional as it contains both spaces and commas // and, more problematic, uses letters instead of exponents for numbers. // For example: // 4.3765M means 4.3765E+6 // 124.10m means 124.10E-3 // -10.084p means -10.084E-12 // 255.44f means 255.44E-15 // // These procedures were written for a particular user's Patchmaster which // started like this: // // Series_1_1 // Sweep #, Mean-80[A], Mean80[A], Timer[s], R-series[Ω], C-slow[F] // 1, -10.084p, 255.44f, 124.10m, 4.3765M, 10.982p // <more data here> // // If your file has a different organization, you will need to customize // these procedures. Menu "Load Waves" "Load Patchmaster 2X90 File...", LoadPatchmaster2X90File("", "", 0, 1) End // FixText(textIn) // Returns cleaned up version of text without bad line breaks. // To keep it simple, this routine assumes that each original line of data // has been split into exactly two lines. // We also assume that the terminator is CRLF. // We also assume that there is a terminator for the last line of the file. // NOTE: You will need to tweak this for your situation static Function/S FixText(textIn) String textIn String textOut = ReplaceString(" ", textIn, "") // Remove spaces textOut = ReplaceString(",", textOut, "\t") // Replaces commas with tabs which make a more readable file textOut = ReplaceString("f", textOut, "E-15", 1) // Replace f (femto) textOut = ReplaceString("p", textOut, "E-12", 1) // Replace p (pico) textOut = ReplaceString("n", textOut, "E-09", 1) // Replace n (nano) textOut = ReplaceString("u", textOut, "E-06", 1) // Replace u (micro) textOut = ReplaceString("m", textOut, "E-03", 1) // Replace m (milli) textOut = ReplaceString("k", textOut, "E+03", 1) // Replace k (kilo) textOut = ReplaceString("M", textOut, "E+06", 1) // Replace M (mega) textOut = ReplaceString("G", textOut, "E+09", 1) // Replace G (giga) textOut = ReplaceString("T", textOut, "E+12", 1) // Replace T (tera) textOut = ReplaceString("P", textOut, "E+15", 1) // Replace P (peta) return textOut End static Function/S GetColumnInfoStr() // Sets wave names String columnInfoStr = "" columnInfoStr += "N='Sweep';" columnInfoStr += "N='MeanMinus80C';" columnInfoStr += "N='MeanPlus80C';" columnInfoStr += "N='Timer';" columnInfoStr += "N='RSeries';" columnInfoStr += "N='CSlow';" return columnInfoStr End // LoadPatchmaster2X90File(pathName, fileName, autoLoadAndGo, createTable) Function LoadPatchmaster2X90File(pathName, fileName, autoLoadAndGo, createTable) String pathName // Name of an Igor symbolic path or "" String fileName // Name of file or full path to file Variable autoLoadAndGo // If non-zero, loads with default names, overwriting any pre-existing waves with those names Variable createTable // 0=no table; 1=create table Variable refNum // First get a valid reference to a file. if ((strlen(pathName)==0) || (strlen(fileName)==0)) // Display dialog looking for file. String fileFilters = "Text Files (*.asc,*.txt,*.dat,*.csv):.asc,.txt,.dat,.csv;" fileFilters += "All Files:.*;" String promptStr = "Choose Patchmaster 2X90 Data File" Open /D /R /P=$pathName /F=fileFilters /M=promptStr refNum as fileName fileName = S_fileName // S_fileName is set by Open/D if (strlen(fileName) == 0) // User cancelled? return -1 endif endif // Open source file and read the raw text from it into a string variable Open/Z=1/R/P=$pathName refNum as fileName if (V_flag != 0) return -1 // Error of some kind endif FStatus refNum // Sets V_logEOF Variable numBytesInFile = V_logEOF String text = PadString("", numBytesInFile, 0x20) FBinRead refNum, text // Read entire file into variable. Close refNum // Fix the text text = FixText(text) // Create cleaned-up version of the file // Write the cleaned up text to a temporary file String tempFileName = fileName + ".noindex" // Use of .noindex prevents Spotlight from indexing the file. Otherwise we get an error when we try to delete the file because Spotlight has it open. Open refNum as tempFileName FBinWrite refNum, text Close refNum // Load the temporary file int dataStartLine = 2 String columnInfoStr = GetColumnInfoStr() // Sets wave names if (autoLoadAndGo) LoadWave/J/D/A/O/E=(createTable)/Q/L={0,dataStartLine,0,0,0}/B=columnInfoStr/P=$pathName tempFileName else LoadWave/J/D/E=(createTable)/Q/L={0,dataStartLine,0,0,0}/B=columnInfoStr/P=$pathName tempFileName endif if (V_flag == 0) Printf "An error occurred while loading data from \"%s\"\r", S_fileName else Printf "Loaded data from \"%s\"\r", S_fileName endif // Delete the temporary file DeleteFile /P=$pathName tempFileName return 0 End
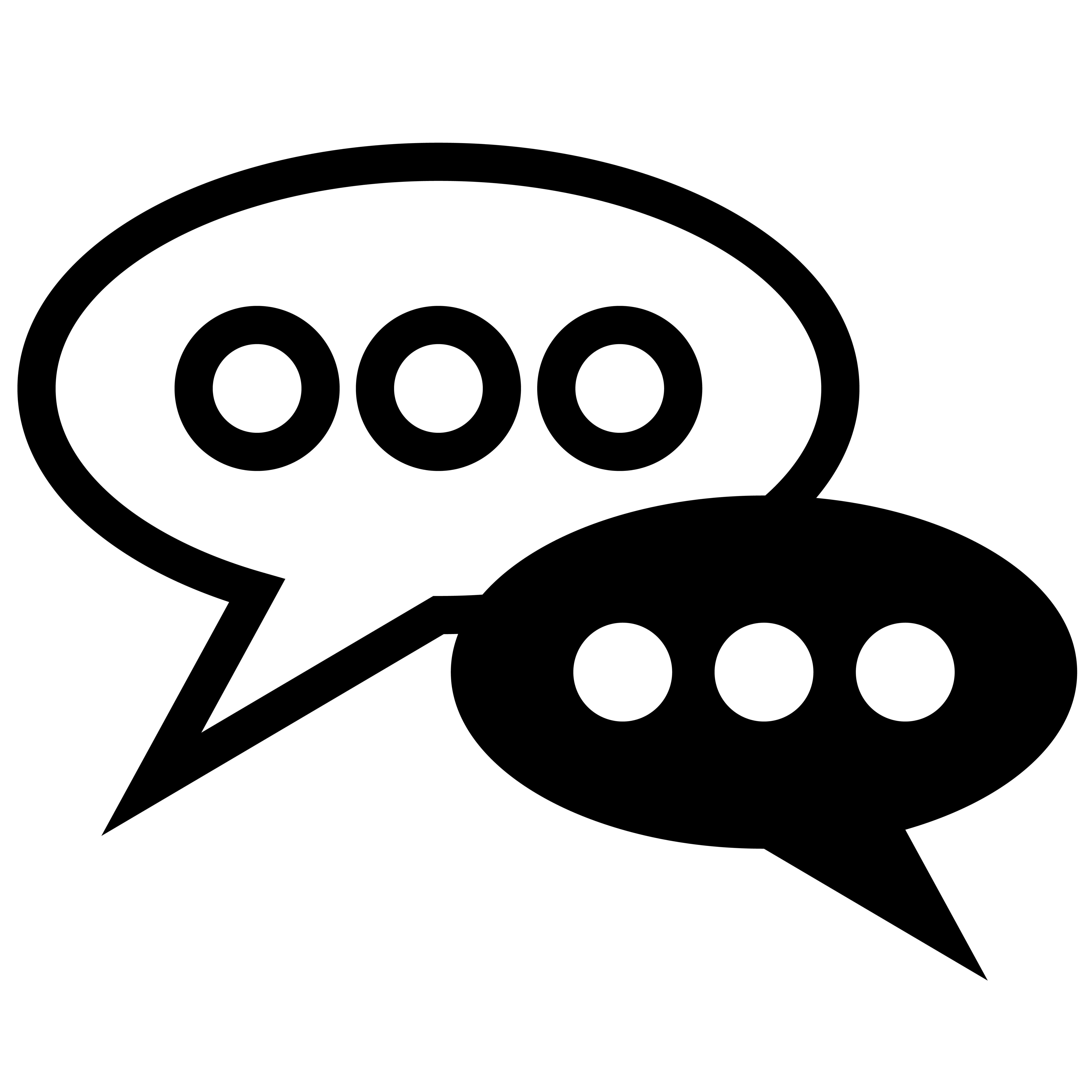
Forum
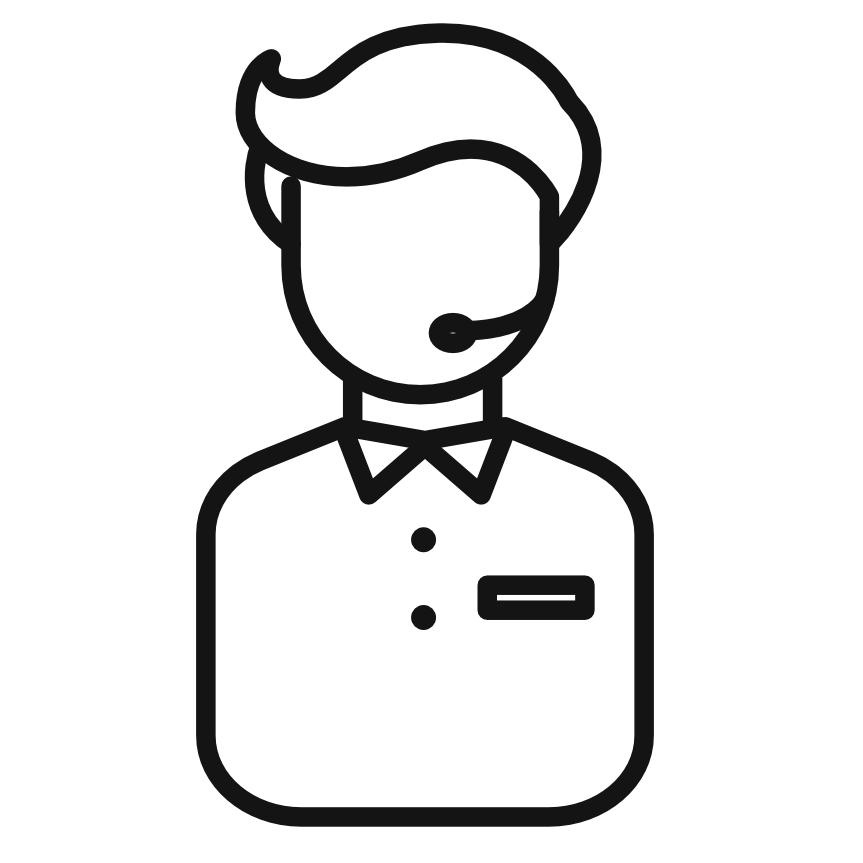
Support
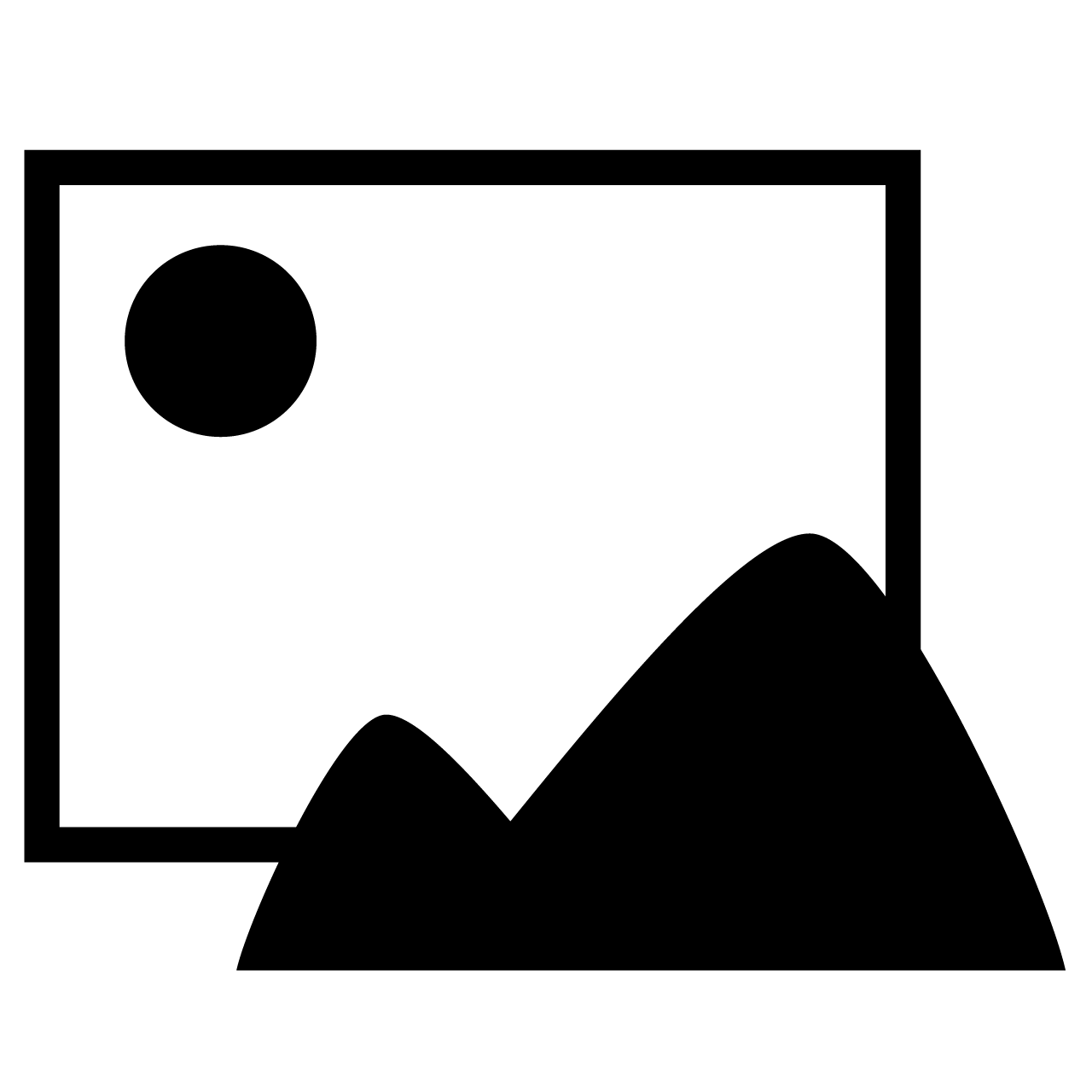
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More