
Using wave elements in a Tag in a function
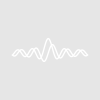
michael.sear
I have a wave named w. I have a function that takes w as an input and does a fit on it, then generates w_fit, w_coef and w_sigma.
I draw w_fit on a graph.
Now I want to write a Tag on w_fit using values from w_coef and w_sigma.
Is this right? Is there a neater way, because this feels to me like I am being clumsy.
Function fitthething(winput)
//... a bunch of fitting stuff
AppendtoGraph $(NameOfWave(winput)+"_fit")
WAVE tempwave1 = $(NameOfWave(winput)+"_coef")
WAVE tempwave2 = $(NameOfWave(winput)+"_sigma")
VARIABLE center = tempwave1[2]
STRING tempa
sprintf tempa,"%0.1f",3.98486*tempwave1[3]
STRING tempb
sprintf tempb,"%0.1f",3.98486*tempwave2[3]
STRING tagtext = tempa + U+00B1 + tempb
Tag $(NameOfWave(winput)+"_fit"), center, tagtext
End
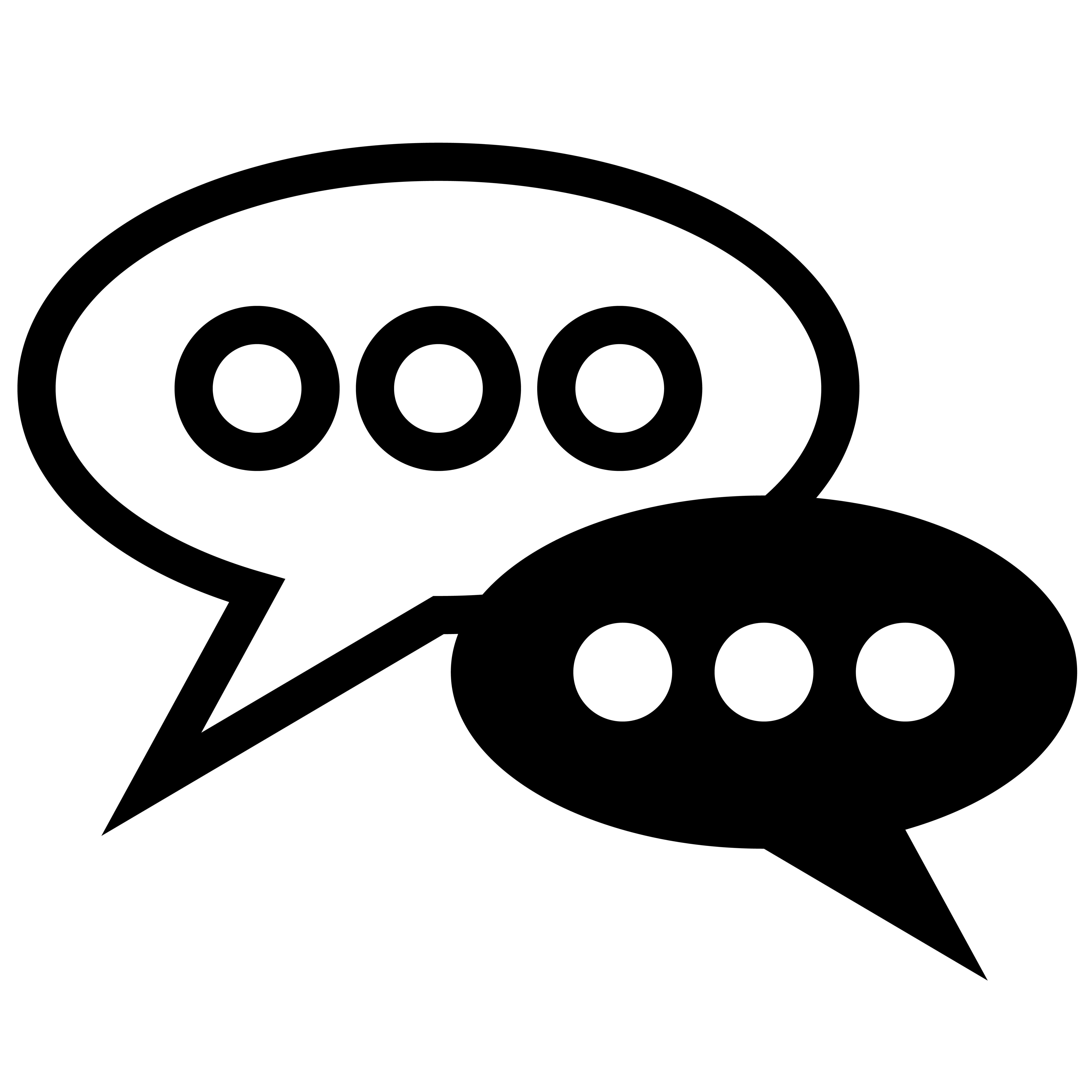
Forum
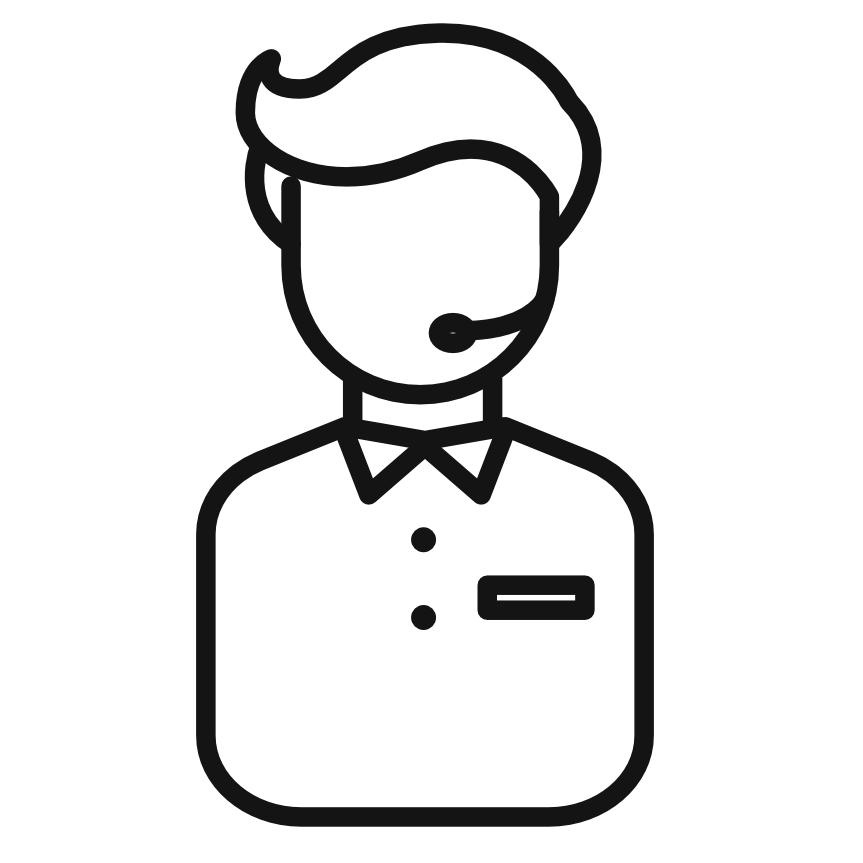
Support
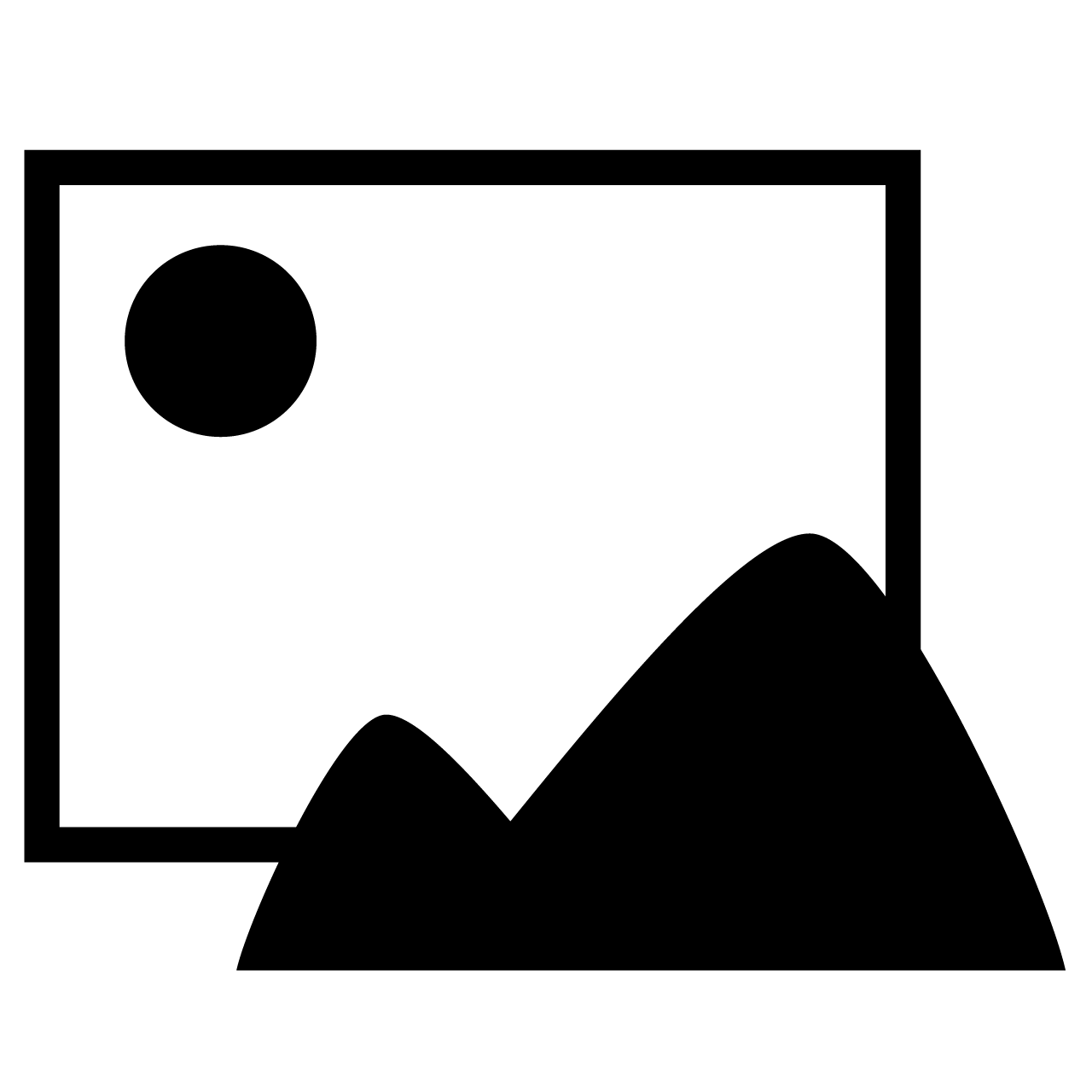
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More
This is the general approach that I would take.
You might separate the function that does the tagging from the function that does the fitting, essentially having a DoMyFit(...) function and a TagMyFit(...) function that are called from a master function FitandShow(...). The DoMyFit function can be set to append the fit curve automatically to the graph. All you will need to do is tag that curve. Since you use NameOfWave more than once, define it as string wname = NameofWave(winput). In the same vein, define variable cf = 3.98486 and do sprintf ... cf*tempwave... for clarity.
August 2, 2019 at 06:56 am - Permalink
When you are dealing with existing waves as in this case, it is best to create a wave reference rather than using $<string> repeatedly. This is more readable, easier to debug, and avoids repetition.
Here is how I would write your function:
Wave wInput
//... a bunch of fitting stuff
String wInputName = NameOfWave(wInput)
String fitWaveName = wInputName + "_fit"
Wave fitWave = $fitWaveName
String coefWaveName = wInputName + "_coef"
Wave coefWave = $coefWaveName
String sigmaWaveName = wInputName + "_sigma"
Wave sigmaWave = $sigmaWaveName
AppendtoGraph fitWave
VARIABLE center = coefWave[2]
STRING tempa
sprintf tempa,"%0.1f",3.98486*coefWave[3]
STRING tempb
sprintf tempb,"%0.1f",3.98486*sigmaWave[3]
STRING tagtext = tempa + U+00B1 + tempb
Tag fitWave, center, tagtext
End
When dealing with waves that may or may not exist, use Wave/Z and then WaveExists to test for the wave's existence.
If you are making a wave that may not yet exist, use Make $<string> or Make/O $<string> before making the wave reference.
I recommend, even for experienced Igor programmers who may need a reminder, reviewing this help topic:
DisplayHelpTopic "Accessing Global Variables And Waves"
August 2, 2019 at 08:01 am - Permalink