
Popup menu with human-readable entries to select wave
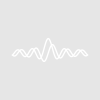
bendrinksalotofcoffee
I have several dozen waves whose names are formatted like type_S_P_D, where "type" is the name of a machine, and S, P, and D are numbers corresponding to test conditions. I would like to populate a Popup menu such that the user sees something human-readable. For example, if the wave is named "Mod1_150_2_20", I would like the popup menu entry to be something like "Mod1, 150 m/s, P=2, D=20". Once selected, the routine would then proceed with analysis of the mod1_150_2_20 wave.
Furthermore, I would like to be able to build this entire human-readable list based on the contents of the data folder when the panel is created. How would I go about this?
> How would I go about this?
With some care.
* Generate a list of waves in the folder: LoWaves
* Translate the text of each list item into human-readable format, generating a new list LoNames
* Use LoNames for the popup selections
* Obtain the wave name by the returned pop value to select from LoWaves
Since you want to do this only once, you can save some effort by storing LoWaves and LoNames as global strings, generated before the panel control uses them. Otherwise, you can create string function to return either case.
You may want to test this first by including a DO IT button on your panel rather than by having a change in the pop up cause the action to happen. Once you are happy with the approach, you could remove the DO IT button and have the action triggered when the pop value changes.
October 28, 2024 at 04:11 pm - Permalink
The following should work:
NOTE: Your 'type' should not contain a underscore character. If it does sometimes, then the parsing needs to be done more carefully (I didn't bother for now).
Try it out:
If you really want to have a static selection upon panel creation instead of a dynamic menu, you can simply save the output of parsePopList() into a string and plug this into PopupMenu upon creation.
October 28, 2024 at 05:44 pm - Permalink