
Faster cycling through waves
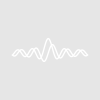
mouthbag
Hello everybody,
I am currently implementing a thresholding algorithm in Igor. I want to decompose my original image wave in smaller section. A threshold will be applied to every single section. Afterwards I want to compose a new wave from the single smaller sections.
I am currently using two for loops in order to paste the smaller waves into the big output wave. See the example below:
for(rowCounter=0;rowCounter<dimsize(smallWave,0):rowCounter++)
for(colCounter=0;colCounter<dimsize(smallWave,1);colCounter++)
bigWave[rowOffset+rowCounter][colOffset+colCounter]=smallWave[rowCounter][colCounter]
endfor
endfor
for(colCounter=0;colCounter<dimsize(smallWave,1);colCounter++)
bigWave[rowOffset+rowCounter][colOffset+colCounter]=smallWave[rowCounter][colCounter]
endfor
endfor
However, my approach seem to be very slow. I was wondering if there is a faster way to assign values to a wave. Maybe something similar to pointers and iterators in C++?
Best regards,
mouthbag
Hi,
Your existing code would go a bit faster by holding the dimsize outputs as variables and not calling them for every pixel.
You can also do the same thing without looping doing a direct wave assignment, like this(untested):
bigWave[rowOffset,rowOffset + (dimsize(SmallWave,0) - 1) ][colOffset,colOffset + (dimsize(SmallWave,1) - 1)] = smallWave[p-rowOffset][q-colOffset]
Where you specify the start and end of your pasted region and then assign the values from smallWave starting at [0][0].
But to answer the question, for this kind of stuff MatrixOp and the Image operations are very fast. e.g. I'm pretty sure one of the ImageTransform keywords is for a stitching operation that you might be able to use for your needs.
September 11, 2018 at 03:45 am - Permalink
After you obtain the smaller image you can insert them into the larger image using ImageTransform with the keyword insertImage.
September 11, 2018 at 01:06 pm - Permalink
I don't know if your custom algorithm can use or accommodate to any of the inbuilt thresholding algorithms, but if it can, then something like this might be the fastest solution:
duplicate/o w_img, w_roi
redimension/b/u w_roi
fastop w_roi = 100 // any non zero value
w_roi[0,127][0,127] = 0 // define the roi
ImageThreshold/o/q/m=1/r=w_roi w_img // overwrite w_img due to the /o flag
fastop w_roi = 100
w_roi[128,255][128,255] = 0
ImageThreshold/o/q/m=1/r=w_roi w_img
This is the closes you'd get to operating on a region of the image wave without memory copy and duplication.
best,
_sk
September 13, 2018 at 03:02 am - Permalink