
Ethernet connection with Keithley devices
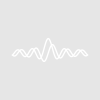
mike365
I am currently working on setting up part of a physics lab as my graduate work and have encountered multiple problems. As right now the biggest problem I am having is trying to interface a Keithley 2701 (with 7711 Switching Module). Previously we have established communication with a Keithley 2450 using the VISA XOP but in attempting to use a similar program we are unable to find the 2701. What is confusing is that I can ping the 2701 at its IP address and get a response, but when I search for it I am unable to locate it using Igor, I have thought about trying to use the SOCKIT XOP but wanted to see what advice if any you all might be able to give me.
Thanks,
Michael
I used SOCKIT XOP to communicate to the switch which was the hub for the 2600. The communication was straight forward though the programming of the Keithley was a bit involved. I had to support multiple forma factors and tests routines which I crafted within Igor and sent them as text tot he Keithley. There was a second set of programming on the Keithey side to handle the necessary sweeps and loops and data arrangement before it was sent back to Igor.
I am rusty on the specifics since it was >6 years ago and I m no longer at that firm. I could try to get a copy of the code used (no guarantees).
Andy
May 12, 2015 at 05:20 pm - Permalink
Also, from http://digital.ni.com/public.nsf/allkb/92D475E7246846E786256ED700556D98, the Keithley 2701 is not a:
This means that you can't control the 2701 using the VISA TCPIP::INSTR protocol. As the second link explains, you have to control it using the TCPIP::SOCKET protocol. This means you are programming it at a low level. You could use VISA XOP for this - it would similar to using the Sockit XOP.
I suspect that using TCPIP::SOCKET via VISA XOP or using the Sockit XOP is a lot more complicated than using the VISA TCPIP::INSTR protocol.
Here is a useful NI link entitled "Ethernet Instrument Control Tutorial" - http://www.ni.com/tutorial/3325/en/
May 12, 2015 at 09:05 pm - Permalink
I appreciate the response, our setup is using a Keithley 2450 SMU and the 2701 with switching cards, we are using them to make measurement on solar cell devices so if you could get the code to me that might be greatly useful to us. I do not know much about the SOCKIT XOP, I have been able to connect to the device but I want to write SCPI instructions to the device using the SOCKITsendmsg but it does not reset when I send *RST. I must be making a mistake but do not know what.
Michael
May 13, 2015 at 01:24 pm - Permalink
Here is the Keithley communication code. We also use the Keithley to control the shutter to the light source. Hope this helps. If I recall once communication was set up, we just passed text back and forth.
May 13, 2015 at 02:50 pm - Permalink