
Bring to front multiple windows
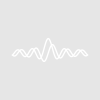
MG
I'm trying to bring up multiple windows when clicking on one of them (e.g. bring to front Graph1, Graph2 and Graph3 -- with Graph2 being the topmost -- when clicking on Graph2). The only solution I came up so far is to define a window hook for all windows I want to show up together like this:
Function myHook(s) STRUCT WMWinHookStruct &s NVAR clicked = root:clicked Variable i String WindowList = "Graph1;Graph2;Graph3;" String clickedWin = s.winName, currWin if ( s.eventCode == 0 ) if ( abs(clicked - s.ticks) < 1 ) return 0 else clicked = s.ticks endif for (i=0; i<ItemsInList(WindowList); i+=1) currWin = StringFromList(i, WindowList) DoWindow /F $currWin endfor DoWindow /F $clickedWin endif End Function DemoWindows() Display /N=Graph1; SetWindow Graph1, hook(myHook) = myHook Display /N=Graph2; SetWindow Graph2, hook(myHook) = myHook Display /N=Graph3; SetWindow Graph3, hook(myHook) = myHook End
The reason I have to use the global variable ("clicked") is to avoid recursion, that is when clicking on Graph2, Graph1 is going to front, which in turn results in a call of "myHook".
Is there a more elegant way of doing this?
Cheers
January 17, 2013 at 07:58 am - Permalink
January 17, 2013 at 09:36 am - Permalink
January 17, 2013 at 12:14 pm - Permalink
However, Dowindow/F is a bit too powerful, it not only brings a window to the front, but also activates it.
The simple solution is put everything else behind it! Just use Dowindow/B inside a for-loop over the items of WinList. Import is the beginning, end and direction of the loop. And exclude the one you need to keep on top. Here's an example (I didn't run it, but it gets the point across):
January 19, 2013 at 09:59 am - Permalink
Just to close this thread and if anybody has a similar problem, I ended up with this solution:
The window that is clicked on is brought to front automatically (which can't be prevented anyway) and all other windows that should go to front are brought to the background just behind the topmost window.
Cheers
February 15, 2013 at 08:09 am - Permalink