
Find all possible distances between two sets of xy coords
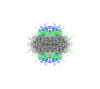
sjr51
Currently I do this:
•make/o/n=(10,2) aa=p*(q+2) // make some integer coords for illustration •make/o/n=(20,2) bb •bb[][0] += 3 •bb[][1] += 4 // second set is 5 units away •make/o/n=(dimsize(aa,0),dimsize(bb,0),2) cc // to contain the difference between each point, x in layer 0, y in layer 1 •matrixtranspose bb •cc[][][0] = aa[p][0] - bb[0][p] •cc[][][1] = aa[p][1] - bb[1][p] // subtract each point in bb from each point in aa •matrixop/o dd = cc * cc •matrixop/o ee = sumbeams(dd) •matrixop/o ff = sqrt(ee) // 2D wave of all distances
I can't do the last three MatrixOps as a compound expression because of sumbeams.
Is there a simple command to do this? Or any other way to simplify these steps? Any input would be appreciated.
Elsewhere in my code I turn these coordinate sets into image masks using
ImageBoundaryToMask
and I looked at image operations to find the distances but couldn't figure out a good way to do this.
Otherwise, here is my way of using matrixop. I broke it down to individual instructions and it is past midnight here so you may want to check it twice.
Now you have to pick the relevant data (array) out of the full matrix. It is not the most efficient but it is probably worth trying.
I hope this helps,
A.G.
WaveMetrics, Inc.
February 28, 2018 at 12:31 am - Permalink
I'm not looking at clusters right now. I actually just want to find the closest distance between the two sets of points.
February 28, 2018 at 04:35 am - Permalink
Look at FPClustering. I think that if you set it correctly you could get the closest distance.
February 28, 2018 at 08:33 am - Permalink
ImageStats can also provide the matrix location of the minimum distance at V_minColLoc and V_minRowLoc. You will have to figure out whether or how to search for other equal minimum distances in your data set.
February 28, 2018 at 09:08 am - Permalink
FPClustering can't work here because it normalizes each column of the position data. It is also difficult to make it output distances that are not clustered. I will look into adding such an option for IP8.
A.G.
WaveMetrics, Inc.
February 28, 2018 at 10:42 am - Permalink
February 28, 2018 at 11:36 am - Permalink
@s.r.chinn thank you for the input. I am using exactly this in my code right now.
February 28, 2018 at 12:24 pm - Permalink