
Using ExecuteScriptText to get a list of all environment variables and their values
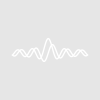
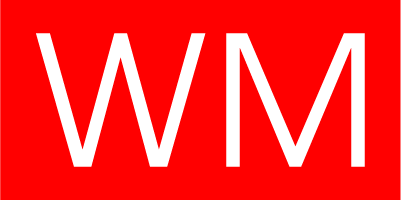
aclight
NOTE: If you're using Igor 7 or above, there's a much easier way to do this. Use the new built-in function
GetEnvironmentVariable
. If you need the values of all environment variables, call GetEnvironmentVariable("=")
. Otherwise, to get the value of a specific environment variable, call GetEnvironmentVariable("USER")
, for example, to get the value of the "USER" environment variable.//** // Using ExecuteScriptText, gets the environment variables. // Variables are returned as a carriage-return-separated // string. // // Usage examples: // print GetEnvironmentVariables() // Prints all variables to Igor's command history // String sys_path = StringByKey("PATH", GetEnvironmentVariables(), "=", "\r") //* Function/S GetEnvironmentVariables() String envInfoString = "" // This has to be done differently depending on the operating system. String platform = IgorInfo(2) String errorMessage StrSwitch (platform) Case "Windows": try // Do some setup. String tmpDir = SpecialDirPath("Temporary", 0, 0, 0) AbortOnValue (numtype(strlen(tmpDir)) != 0 || !(strlen(tmpDir) > 0)), 1 // Make sure that the directory we just got is, in fact, a directory. GetFileFolderInfo/Q tmpDir AbortOnValue (V_Flag >= 0 && !V_isFolder), 3 // Set an Igor symbolic path to the temporary directory. String tmpDirectoryPath = UniqueName("tmpPath", 12, 0) NewPath/Q $(tmpDirectoryPath), tmpDir AbortOnValue (V_flag), 5 // Setting of the new path failed. // Write a temporary batch file to the temporary directory that will // call the "set" command from the command shell and save the // output of the command to a temporary file. String tempBatFileName, tempResultsFileName String tempBatFileFullPath, tempResultsFileFullPath Variable tempBatFileRefNum, tempResultsFileRefNum sprintf tempBatFileName, "IgorGetEnvironmentVars_%.4u.bat", abs(trunc(StopMsTimer(-2))) Open/P=$(tmpDirectoryPath)/T=".bat"/Z=1 tempBatFileRefNum as tempBatFileName AbortOnValue (V_flag != 0), 7 // Add a path separator character to the end of the path, if necessary, and add on the file name. tempBatFileFullPath = ParseFilePath(2, tmpDir, ":", 0, 0) + tempBatFileName // Convert the path into a windows path that uses "\" as the path separator. tempBatFileFullPath = ParseFilePath(5, tempBatFileFullPath, "\\", 0, 0) sprintf tempResultsFileName, "IgorGetEnvironmentVars_%.4u.txt", abs(trunc(StopMsTimer(-2))) // Add a path separator character to the end of the path, if necessary, and add on the file name. tempResultsFileFullPath = ParseFilePath(2, tmpDir, ":", 0, 0) + tempResultsFileName // Convert the path into a windows path that uses "\" as the path separator. tempResultsFileFullPath = ParseFilePath(5, tempResultsFileFullPath, "\\", 0, 0) fprintf tempBatFileRefNum, "set > \"%s\"\r\n", tempResultsFileFullPath Close tempBatFileRefNum // Call the batch file we just created. Timeout after 2 seconds if this doesn't succeed. String scriptText sprintf scriptText, "cmd.exe /C \"%s\"", tempBatFileFullPath ExecuteScriptText/W=2/Z scriptText // Check for an error. AbortOnValue (V_flag != 0), 9 // Check that the temporary results file exists. GetFileFolderInfo/Z=1/Q/P=$(tmpDirectoryPath) tempResultsFileName AbortOnValue (V_flag != 0 || !(V_isFile)), 10 // Get the results from the temporary file created by the batch file. Open/P=$(tmpDirectoryPath)/R/Z=1 tempResultsFileRefNum as tempResultsFileName AbortOnValue (V_flag != 0), 12 String buffer do FReadLine tempResultsFileRefNum, buffer if (strlen(buffer) <= 0) break else envInfoString += buffer endif while (1) Close tempResultsFileRefNum // Delete the temporary batch file and temporary results file. DeleteFile/P=$(tmpDirectoryPath)/Z=1 tempBatFileName DeleteFile/P=$(tmpDirectoryPath)/Z=1 tempResultsFileName // If we get an error here we don't really care. We've already got // the goods, so just run. break catch // Error handling Switch (V_abortCode) Case 1: errorMessage = "Could not locate the operating system's temporary directory, so can't get environment variables." break Case 3: errorMessage = "Could not locate the operating system's temporary directory, so can't get environment variables." break Case 5: errorMessage = "Igor was unable to create a symbolic path to the temporary file directory." break Case 7: errorMessage = "Igor was unable to create a batch file in the temporary directory." break Case 9: errorMessage = "Igor was unable to execute the batch file to read the environment variables." break Case 10: errorMessage = "Igor was unable to locate or read the temporary file containing environment variables." break Case 12: errorMessage = "Igor was unable to open the temporary file containing environment variables." break default: errorMessage = "An unspecified error occurred while trying to read the environment variables." EndSwitch print errorMessage KillPath/Z $(tmpDirectoryPath) return "" endtry Case "Macintosh": // This is a lot easier on the Macintosh because we can just use AppleScript. try String appleScriptCommand = "do shell script \"env\"" ExecuteScriptText/Z appleScriptCommand AbortOnValue V_flag, 1 envInfoString = S_Value catch errorMessage = "Could not execute AppleScript command." print errorMessage return "" endtry break EndSwitch return envInfoString End
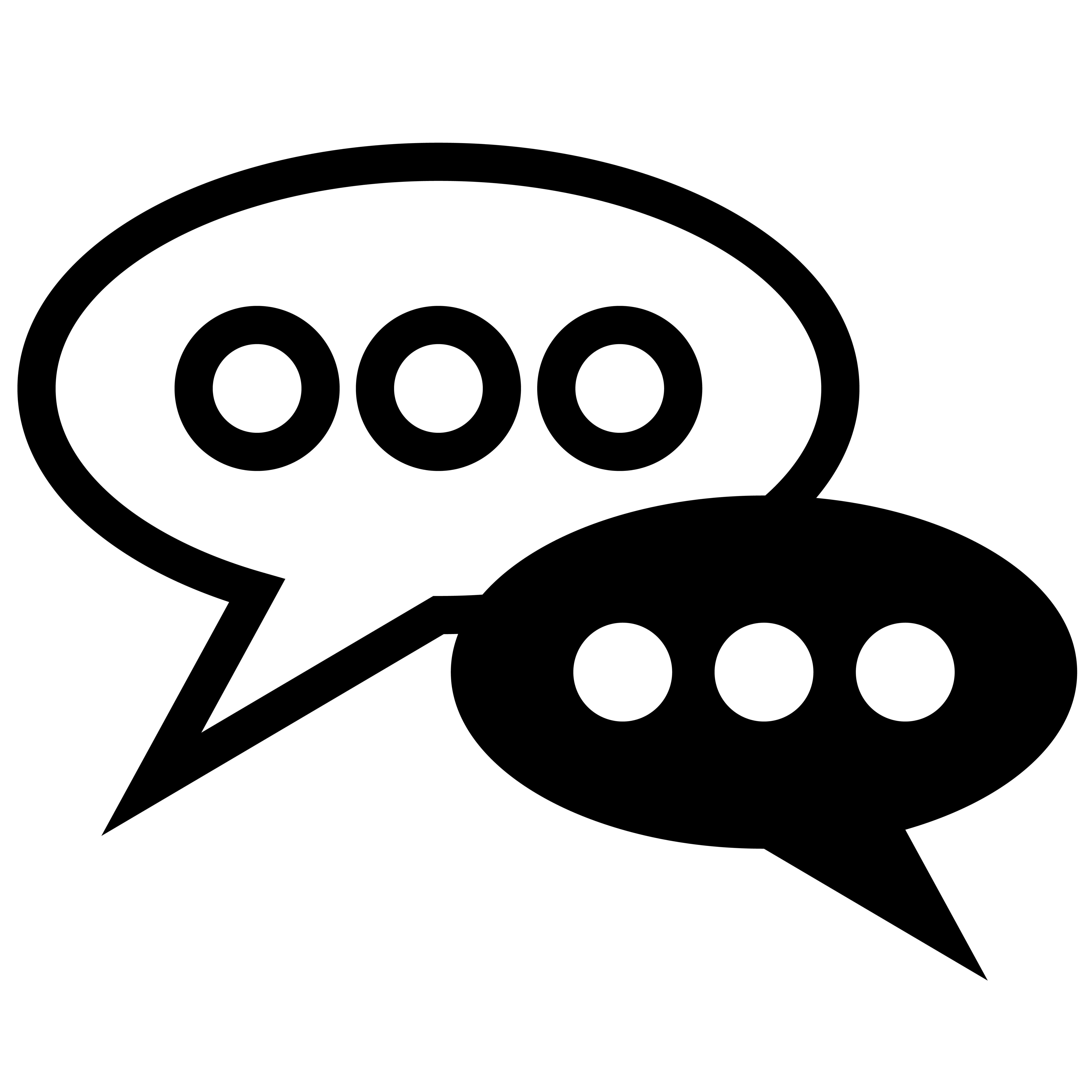
Forum
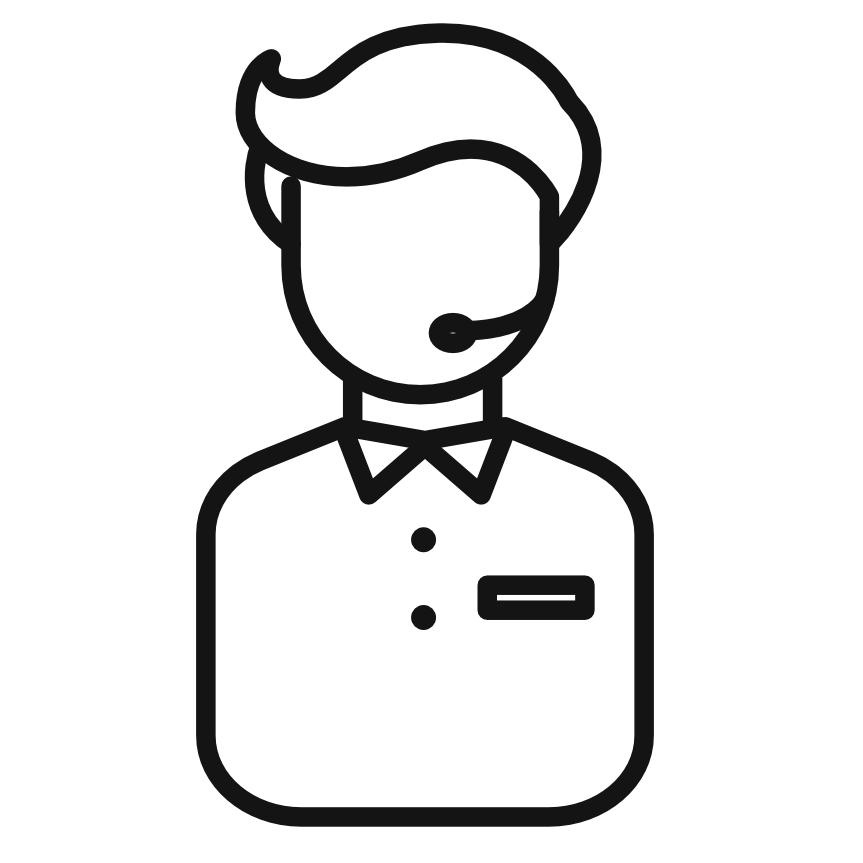
Support
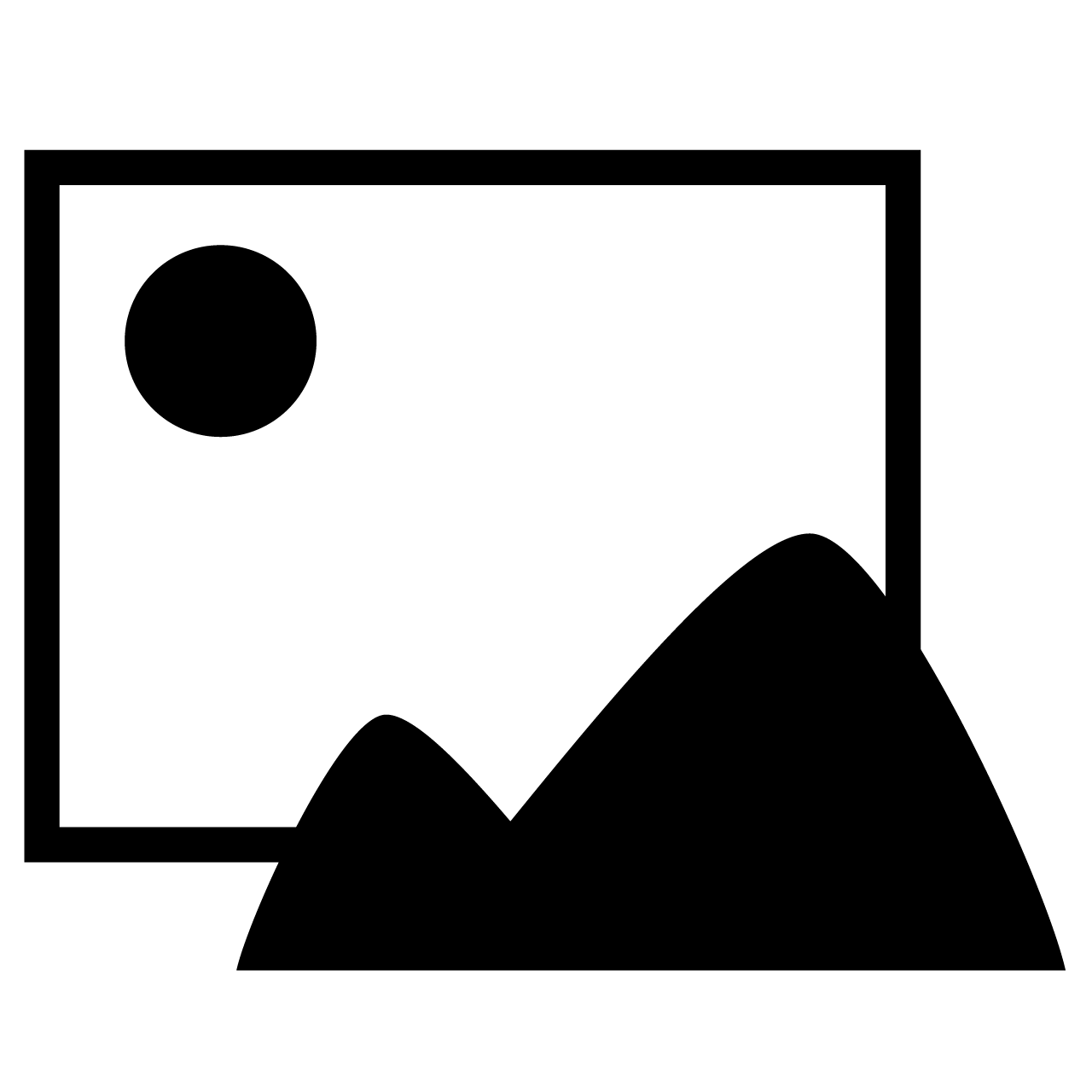
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More