
Polar Image

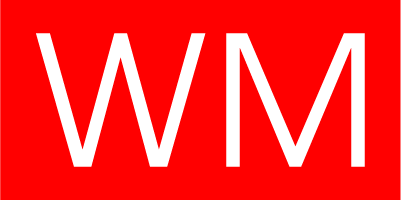
JimProuty
The method involves interpolating to a grid, using NaN to render the portion outside of the polar circle transparent.
This version adds two parameters to set the angle=0 location and whether angles increase clockwise or counter-clockwise.
#pragma rtGlobals=1 // Use modern global access method. #include <MatrixToXYZ>, menus=0 #include <XYZToMatrix>, menus=0 #include <XYZToTripletToXYZ>, menus=0 Macro DisplayPolarImage(mat,degreesOrRadians,xIsAngle, angle0WhereStr, angleDirectionStr) String mat Prompt mat,"radius/angle matrix",popup,WaveList("*",";","DIMS:2") Variable degreesOrRadians=2 // initially radians Prompt degreesOrRadians,"angle units",popup,"degrees;radians;" // 1,2 Variable xIsAngle=1 // X Scaling Prompt xIsAngle,"Angle from",popup,"X scaling;Y scaling;" // 1,2 String angle0WhereStr= "right" // 0 degrees Prompt angle0WhereStr, "Angle 0 at:", popup, "top;left;right;bottom;" String angleDirectionStr="counter-clockwise" Prompt angleDirectionStr, "Angles Increase:", popup, "clockwise;counter-clockwise;" Silent 1; PauseUpdate MatrixToXYZ(mat,mat,2,2) // creates mat+"X", mat+"Y", mat+"Z" waves String zWave= mat+"Z" String angleWave, radiusWave if( xIsAngle == 1 ) // X dimension is angle angleWave= mat+"X" radiusWave= mat+"Y" else // Y dimension is angle angleWave= mat+"Y" radiusWave= mat+"X" endif // WMRadiusAndAngleInRadianstoXY needs radians if( degreesOrRadians == 1 ) // degrees $angleWave *= pi/180 // convert to radians endif // Apply polar to rectangular conversion into an XYZ matrix Variable zeroAngleWhere=0 if( CmpStr(angle0WhereStr,"top") == 0 ) zeroAngleWhere= 90 endif if ( CmpStr(angle0WhereStr,"left") == 0 ) zeroAngleWhere= 180 endif if ( CmpStr(angle0WhereStr,"bottom") == 0 ) zeroAngleWhere= -90 endif Variable angleDirection = 1 // clockwise if( CmpStr(angleDirectionStr, "counter-clockwise") == 0 ) angleDirection= -1 endif WMRadiusAndAngleInRadianstoXY($radiusWave,$angleWave,zeroAngleWhere,angleDirection) // Change of Variables String xWave= radiusWave String yWave= angleWave // Create and display a (rectangular) image from the resulting XY (circular) domain String matName= UniqueName(mat+"Image",1,0) XYZtoMatrix(xWave,matName,yWave,,zWave,,2,) // specify your own density and whether to display End // convert two waves in-place from radius, angle (in radians!) to x,y Function WMRadiusAndAngleInRadianstoXY(rw,aw, zeroAngleWhereDegrees, positiveForClockwise) Wave rw,aw // radius, angle in radians Variable zeroAngleWhereDegrees // 0 is right, -90 is bottom, 90 is top, +/180 is left Variable positiveForClockwise Variable zeroAngleRadians= zeroAngleWhereDegrees * pi/180 Variable isClockwise= positiveForClockwise > 0 Variable i, n= numpnts(rw) Variable xx, yy for(i=0; i<n; i+=1 ) Variable angle= aw[i] if( isClockwise ) angle= zeroAngleRadians - angle else angle= zeroAngleRadians + angle endif xx= rw[i] * cos(angle) yy= rw[i] * sin(angle) rw[i]= xx aw[i]= yy endfor end Macro MakeDemoRadialMatrix() Variable nx=25 Variable ny=50 Make/O/N=(nx,ny) matrix SetScale x -180, 180, "deg", matrix // x dimension is angle, +/- pi SetScale y 3,50,"m", matrix // y dimension is radius, 3 to 50 matrix= y * sin(x/180*pi) * sin(x/180*pi) Newimage matrix ModifyGraph nticks(top)=5 End
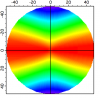
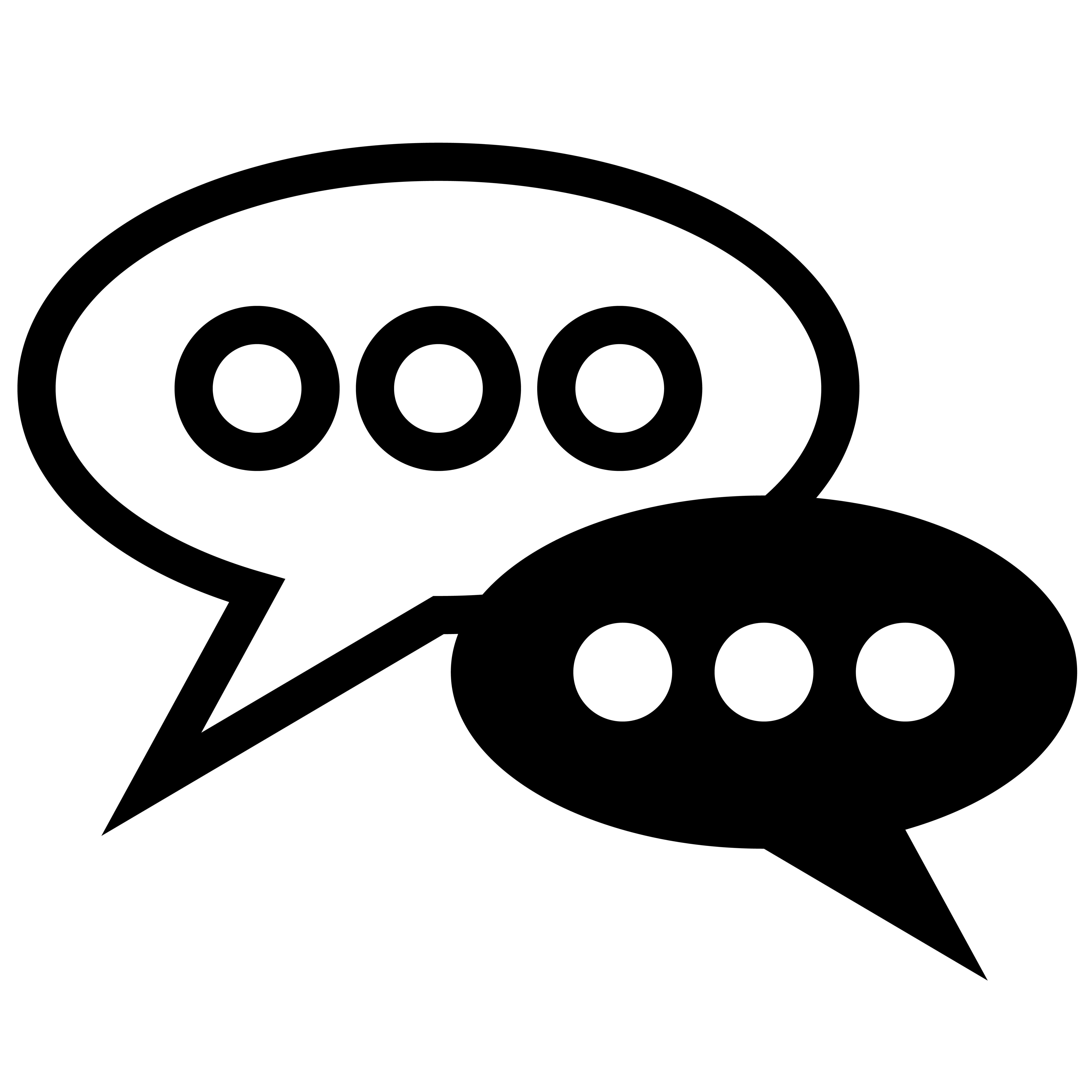
Forum
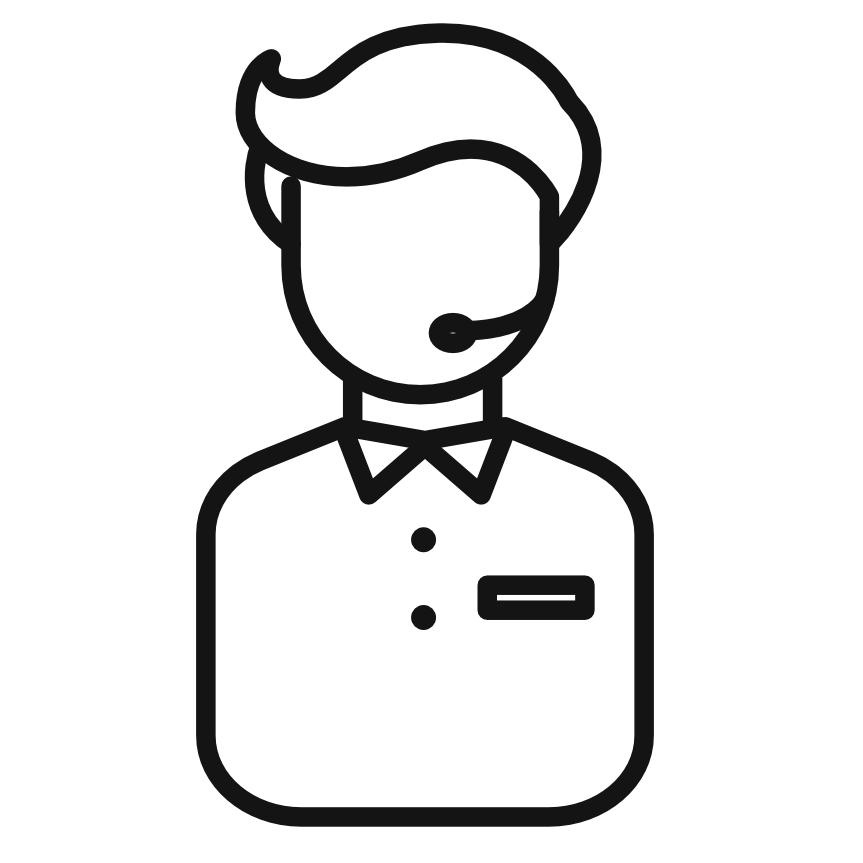
Support
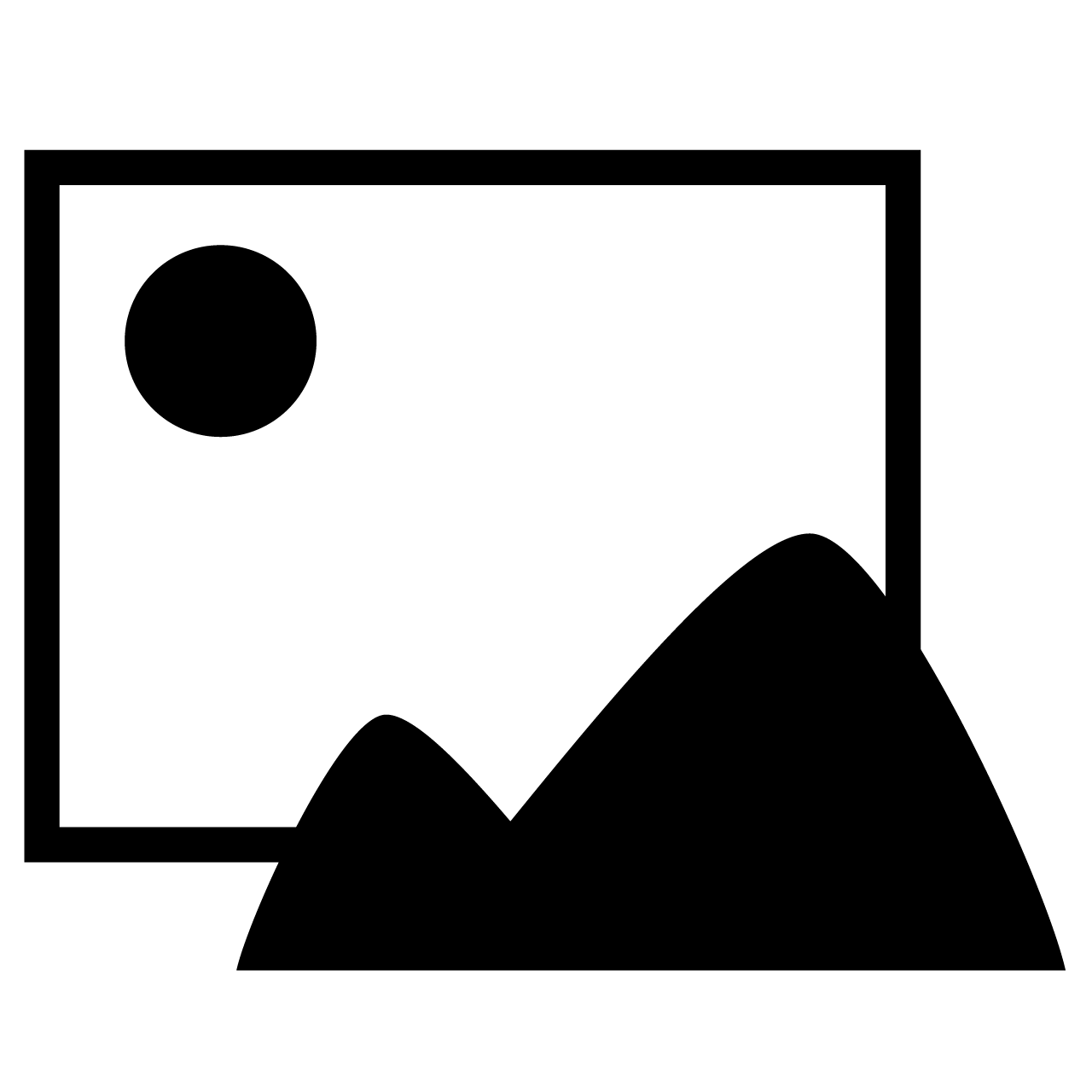
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More