
Layout Front Set (Graph + Panel)
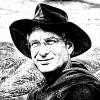
jjweimer
I am frequently having to create a layout that should contain a graph with results and a panel with the inputs that generated the results. The code below will do the trick.
This snippet may also be useful to folks who use Igor Pro in classroom assignments. The layout has a stamp of information giving the experiment, user name, machine, and date + time. This may help you to police against students having the temptation to copy someone else's work.
// Layout Front Set
// v 2021-11-10 jjw
// create a layout with topmost figure to left, topmost panel to right
// respects aspect ratios of graph and panel, override input to have graph fill its region
// puts stamp of information at bottom right
// requires Igor Pro 7 or greater
// *** change these for your preferred layout page size
// US letter page in landscape with 1/4 in margin
Static Constant pgwidth = 792
Static Constant pgheight = 612
Static Constant pgmargin = 18
// set the fraction for the graph on the page left side
Static Constant gfrac = 0.75
Menu "Macros"
"Layout Front Set",/Q, layout_FrontSet()
end
// directly create layout
Function layout_FrontSet()
string gname, pname
gname = WinName(0,1,1)
pname = WinName(0,64,1)
layout_results(gname, pname)
return 0
end
// function available to call from other routines
// set gfill = 1 to have graph fill its region
Function layout_results(string graphname, string panelname, [variable gfill])
// parameters
variable gwidth, gheight, pwidth, pheight
variable grwidth, prwidth
variable gratio, pratio, wthratio
variable toffset, tsize
// sizes of regions for appending
grwidth = gfrac*(pgwidth-2*pgmargin)
prwidth = (1 - gfrac)*(pgwidth-3*pgmargin)
gratio = grwidth/(pgheight-2*pgmargin)
pratio = prwidth/(pgheight-2*pgmargin-4*pgmargin)
// validity checks and window sizes
DoWindow/F $graphname
if (v_flag == 0)
return -1
endif
GetWindow/Z $graphname wsize
gwidth = V_right - V_left; gheight = V_bottom - V_top
//print gwidth, gheight
DoWindow/F $panelname
if (v_flag == 0)
return -1
endif
SavePICT/SNAP=1/O/WIN=$panelname/P=_PictGallery_/E=-5 as "PanelSnapShot"
GetWindow/Z $panelname wsize
pwidth = V_right - V_left; pheight = V_bottom - V_top
// strings for stamp
string enstr, strdt, strsys, strprbx
enstr = IgorInfo(1) + "\r"
strdt = date() + " " + time()
strsys = IgorInfo(7) + " : " + IgorInfo(2)
strprbx = "\\Z10\\JR" + enstr + strsys
strprbx += "\r" + strdt
// remove existing layout window to refresh
KillWindow/Z SnapshotResultsLayout
NewLayout/K=1/W=(30,50,600,500) as "Results Layout"
DoWindow/C SnapshotResultsLayout
LayoutPageAction size=(pgwidth,pgheight),margins=(pgmargin,pgmargin,pgmargin,pgmargin)
ModifyLayout mag=0.5
// append graph
if (ParamIsDefault(gfill))
wthratio = gwidth/gheight
if (wthratio > gratio)
// too wide, shrink height by ratio
tsize = gheight*(grwidth/gwidth)
toffset = 2*pgmargin
AppendLayoutObject/F=0/T=0/R=(pgmargin,toffset,grwidth+pgmargin,tsize+toffset) Graph $graphname
//print "too wide", wthratio, grwidth/tsize
else
// too tall, shrink width by ratio
toffset = pgmargin
tsize = gwidth*(pgheight-2*pgmargin)/gheight
AppendLayoutObject/F=0/T=0/R=(pgmargin,toffset,tsize+pgmargin,pgheight-pgmargin) Graph $graphname
//print "too tall", wthratio, tsize/(pgheight-2*pgmargin)
endif
else
AppendLayoutObject/F=0/T=0/R=(pgmargin,pgmargin,grwidth+pgmargin,pgheight-2*pgmargin) Graph $graphname
endif
// append panel
wthratio = pwidth/pheight
SetDrawLayer ProgBack
if (wthratio > pratio)
//print "wide panel", pwidth, pheight, wthratio, pratio
toffset = pratio*pheight - 2*pgmargin
pratio = prwidth/pwidth
else
//print "tall panel", pwidth, pheight
toffset = pgmargin
pratio = (pgheight-2*pgmargin-4*pgmargin)/pheight
endif
DrawPICT grwidth+pgmargin,toffset,pratio,pratio,PanelSnapShot
// add text stamp in draw layer (keeping it "immutable")
DrawText grwidth+2*pgmargin, pgheight-1.5*pgmargin, strprbx
SetDrawLayer UserFront
return 0
end
// v 2021-11-10 jjw
// create a layout with topmost figure to left, topmost panel to right
// respects aspect ratios of graph and panel, override input to have graph fill its region
// puts stamp of information at bottom right
// requires Igor Pro 7 or greater
// *** change these for your preferred layout page size
// US letter page in landscape with 1/4 in margin
Static Constant pgwidth = 792
Static Constant pgheight = 612
Static Constant pgmargin = 18
// set the fraction for the graph on the page left side
Static Constant gfrac = 0.75
Menu "Macros"
"Layout Front Set",/Q, layout_FrontSet()
end
// directly create layout
Function layout_FrontSet()
string gname, pname
gname = WinName(0,1,1)
pname = WinName(0,64,1)
layout_results(gname, pname)
return 0
end
// function available to call from other routines
// set gfill = 1 to have graph fill its region
Function layout_results(string graphname, string panelname, [variable gfill])
// parameters
variable gwidth, gheight, pwidth, pheight
variable grwidth, prwidth
variable gratio, pratio, wthratio
variable toffset, tsize
// sizes of regions for appending
grwidth = gfrac*(pgwidth-2*pgmargin)
prwidth = (1 - gfrac)*(pgwidth-3*pgmargin)
gratio = grwidth/(pgheight-2*pgmargin)
pratio = prwidth/(pgheight-2*pgmargin-4*pgmargin)
// validity checks and window sizes
DoWindow/F $graphname
if (v_flag == 0)
return -1
endif
GetWindow/Z $graphname wsize
gwidth = V_right - V_left; gheight = V_bottom - V_top
//print gwidth, gheight
DoWindow/F $panelname
if (v_flag == 0)
return -1
endif
SavePICT/SNAP=1/O/WIN=$panelname/P=_PictGallery_/E=-5 as "PanelSnapShot"
GetWindow/Z $panelname wsize
pwidth = V_right - V_left; pheight = V_bottom - V_top
// strings for stamp
string enstr, strdt, strsys, strprbx
enstr = IgorInfo(1) + "\r"
strdt = date() + " " + time()
strsys = IgorInfo(7) + " : " + IgorInfo(2)
strprbx = "\\Z10\\JR" + enstr + strsys
strprbx += "\r" + strdt
// remove existing layout window to refresh
KillWindow/Z SnapshotResultsLayout
NewLayout/K=1/W=(30,50,600,500) as "Results Layout"
DoWindow/C SnapshotResultsLayout
LayoutPageAction size=(pgwidth,pgheight),margins=(pgmargin,pgmargin,pgmargin,pgmargin)
ModifyLayout mag=0.5
// append graph
if (ParamIsDefault(gfill))
wthratio = gwidth/gheight
if (wthratio > gratio)
// too wide, shrink height by ratio
tsize = gheight*(grwidth/gwidth)
toffset = 2*pgmargin
AppendLayoutObject/F=0/T=0/R=(pgmargin,toffset,grwidth+pgmargin,tsize+toffset) Graph $graphname
//print "too wide", wthratio, grwidth/tsize
else
// too tall, shrink width by ratio
toffset = pgmargin
tsize = gwidth*(pgheight-2*pgmargin)/gheight
AppendLayoutObject/F=0/T=0/R=(pgmargin,toffset,tsize+pgmargin,pgheight-pgmargin) Graph $graphname
//print "too tall", wthratio, tsize/(pgheight-2*pgmargin)
endif
else
AppendLayoutObject/F=0/T=0/R=(pgmargin,pgmargin,grwidth+pgmargin,pgheight-2*pgmargin) Graph $graphname
endif
// append panel
wthratio = pwidth/pheight
SetDrawLayer ProgBack
if (wthratio > pratio)
//print "wide panel", pwidth, pheight, wthratio, pratio
toffset = pratio*pheight - 2*pgmargin
pratio = prwidth/pwidth
else
//print "tall panel", pwidth, pheight
toffset = pgmargin
pratio = (pgheight-2*pgmargin-4*pgmargin)/pheight
endif
DrawPICT grwidth+pgmargin,toffset,pratio,pratio,PanelSnapShot
// add text stamp in draw layer (keeping it "immutable")
DrawText grwidth+2*pgmargin, pgheight-1.5*pgmargin, strprbx
SetDrawLayer UserFront
return 0
end
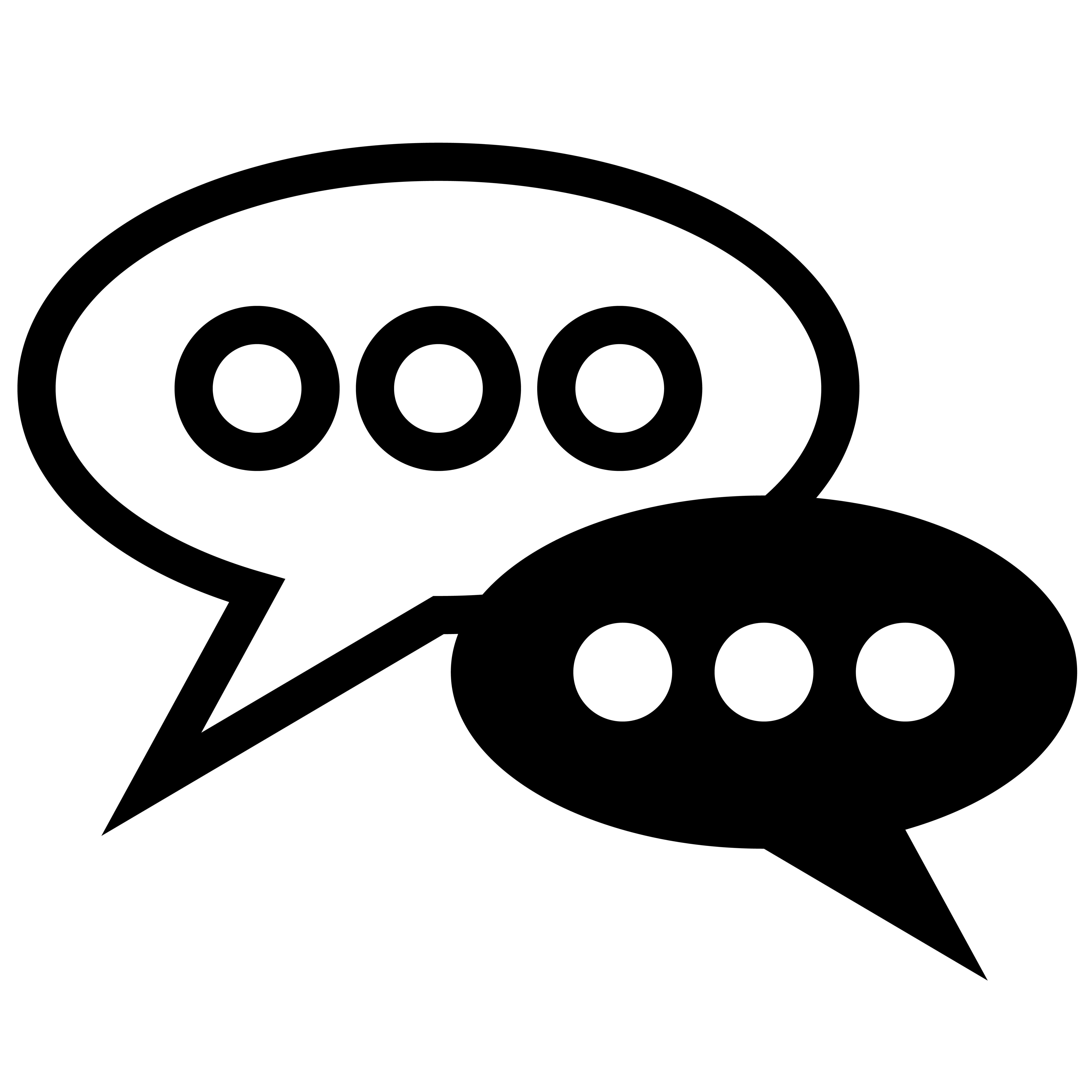
Forum
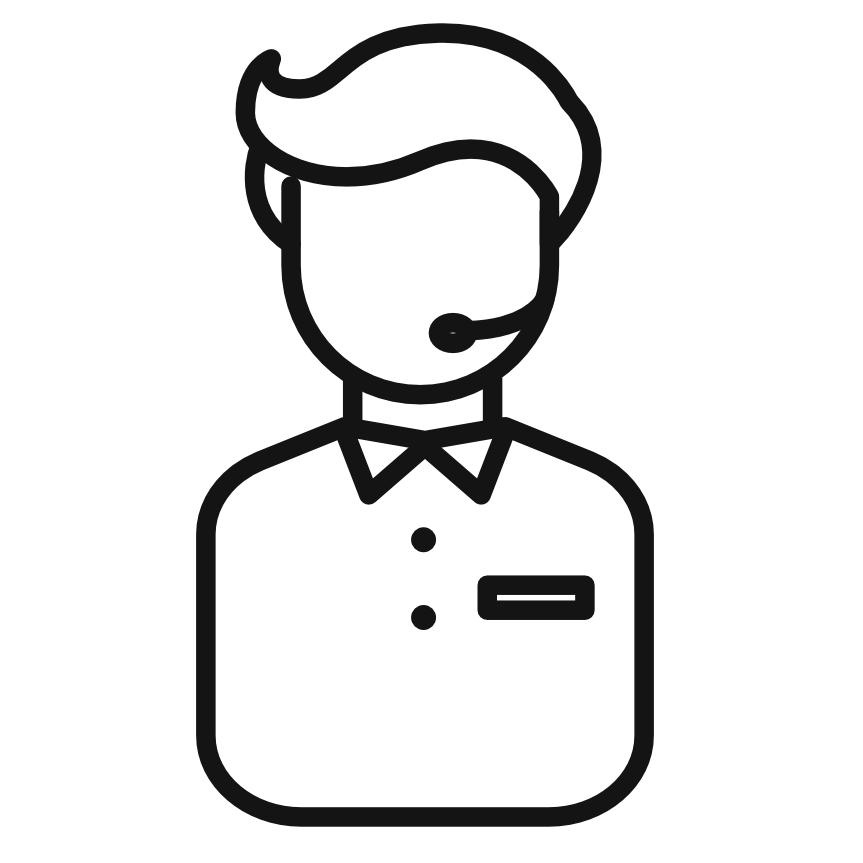
Support
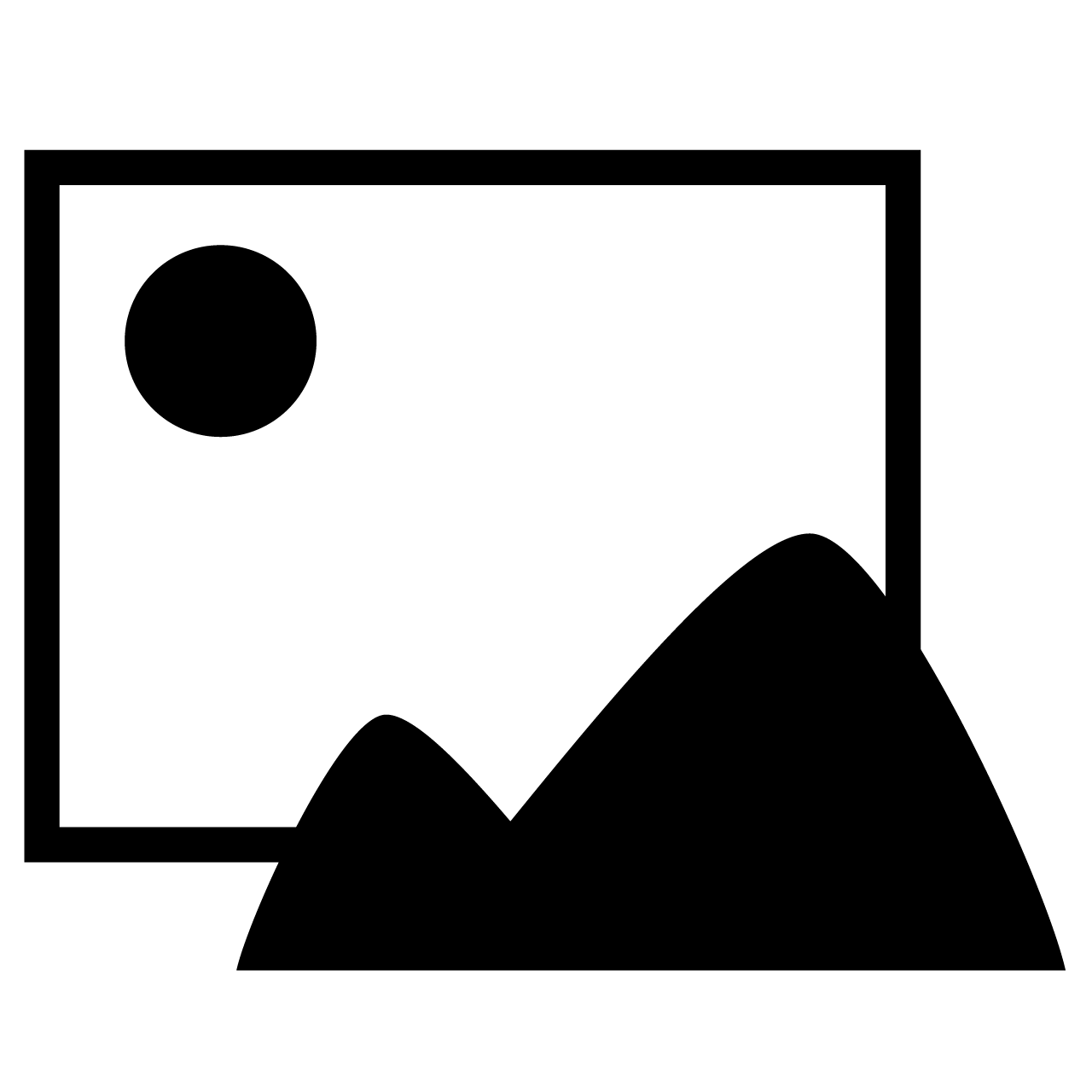
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More