
Exterminate Wave
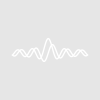
harneit
function ExterminateWave(w) wave w variable retVal = 0 if( WaveExists(w) ) RemoveWaveFormAllWindows(w) CheckDisplayed /A w // successfully removed (e.g. subwindows not treated yet) ? if( V_Flag ) retVal = -1 // code for "in use in windows" endif try // this may fail since do not treat user functions (yet) KillWaves w // no /Z flag, since that does not generate a V_Flag return value catch // but any user of this function really wants to know if it did not work retVal = -2 // code for "in use in functions" endtry endif return retVal end function RemoveWaveFormAllWindows(w) wave w CheckDisplayed /A w if( V_Flag ) RemoveWaveFromAllGraphs(w) RemoveWaveFromAllTables(w) endif end function RemoveWaveFromAllGraphs(w) wave w string graphList = WindowsUsingWave(w, 1) variable k for( k = 0; k < ItemsInList(graphList); k += 1 ) RemoveWaveFromGraph(StringFromList(k, graphList), w, -1) endfor end function RemoveWaveFromAllTables(w) wave w string table, tableList = WindowsUsingWave(w, 2) variable numCols, oldCols, k for( k = 0; k < ItemsInList(tableList); k += 1 ) table = StringFromList(k, tableList) numCols = NumberByKey("COLUMNS", TableInfo(table, -2)) do oldCols = numCols RemoveFromTable /W=$table w // RemoveFromTable is not picky about column specs... numCols = NumberByKey("COLUMNS", TableInfo(table, -2)) while( oldCols > numCols ) if( numCols == 0 ) DoWindow /K $table endif endfor end function/S WindowsUsingWave(w, windowtype) wave w variable windowtype string win, wins = WinList("*", ";", "WIN:"+num2str(windowtype)) // should be modified to include subwindows string result = "" variable k for( k = 0; k < ItemsInList(wins); k += 1 ) win = StringFromList(k, wins) CheckDisplayed /W=$win w // this may not be the best way... if( V_Flag ) result = AddListItem(win, result) endif endfor return result end function RemoveWaveFromGraph(graph, w, options) string graph wave w variable options // bit-wise: 1=waves, 2=contours, 4=images; pass -1 for "all" string wavPath = GetWavesDataFolder(w, 2) // full path of data folder, including possibly quoted wave name string allObjects = "" string instanceList, instance, objPath, xwavePath variable k if( options & 1 ) // traces instanceList = TraceNameList(graph, ";", 1) allObjects += instanceList for( k = ItemsInList(instanceList); k > 0 ; k -= 1 ) // need backward loop since ... instance = StringFromList(k-1, instanceList) objPath = GetWavesDataFolder(TraceNameToWaveRef(graph, instance), 2) wave xwave = XWaveRefFromTrace(graph, instance) if( WaveExists(xwave) ) xwavePath = GetWavesDataFolder(xwave, 2) else xwavePath = "" endif if( !cmpstr(wavPath, objPath) || !cmpstr(wavPath, xwavePath) ) RemoveFromGraph /W=$graph $instance // ... this changes instance numbers allObjects = RemoveFromList(instance, allObjects) endif endfor endif if( options & 2 ) // contours instanceList = ContourNameList(graph, ";") allObjects += instanceList for( k = ItemsInList(instanceList); k > 0 ; k -= 1 ) // need backward loop since ... instance = StringFromList(k-1, instanceList) objPath = GetWavesDataFolder(ContourNameToWaveRef(graph, instance), 2) if( !cmpstr(wavPath, objPath) ) RemoveContour /W=$graph $instance // ... this changes instance numbers allObjects = RemoveFromList(instance, allObjects) endif endfor endif if( options & 4 ) // images instanceList = ImageNameList(graph, ";") allObjects += instanceList for( k = ItemsInList(instanceList); k > 0 ; k -= 1 ) // need backward loop since ... instance = StringFromList(k-1, instanceList) objPath = GetWavesDataFolder(ImageNameToWaveRef(graph, instance), 2) if( !cmpstr(wavPath, objPath) ) RemoveImage /W=$graph $instance // ... this changes instance numbers allObjects = RemoveFromList(instance, allObjects) endif endfor endif if( ItemsInList(allObjects) == 0 ) DoWindow /K $graph endif end
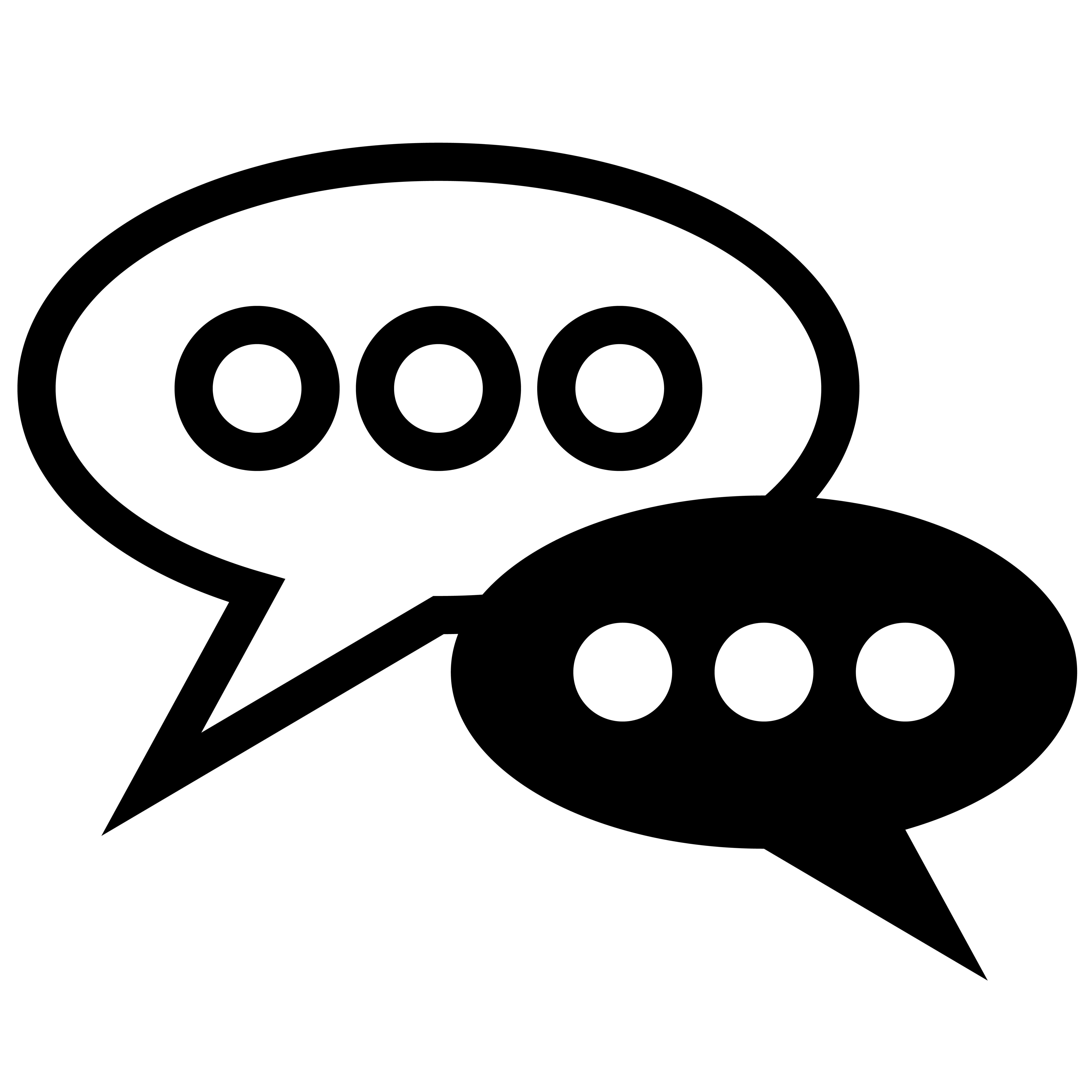
Forum
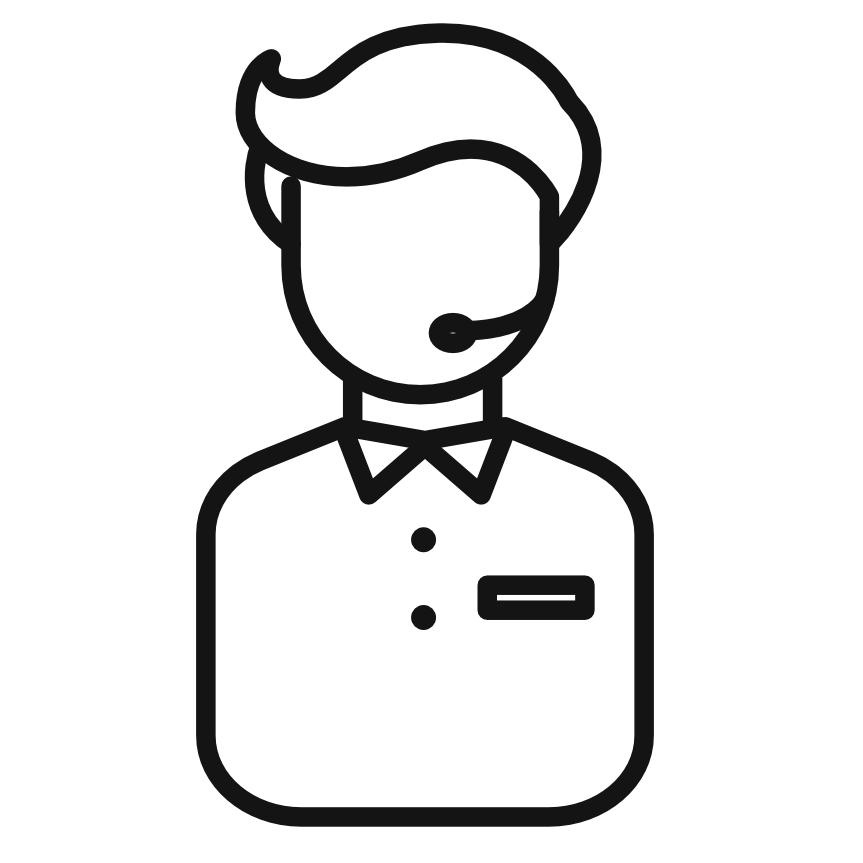
Support
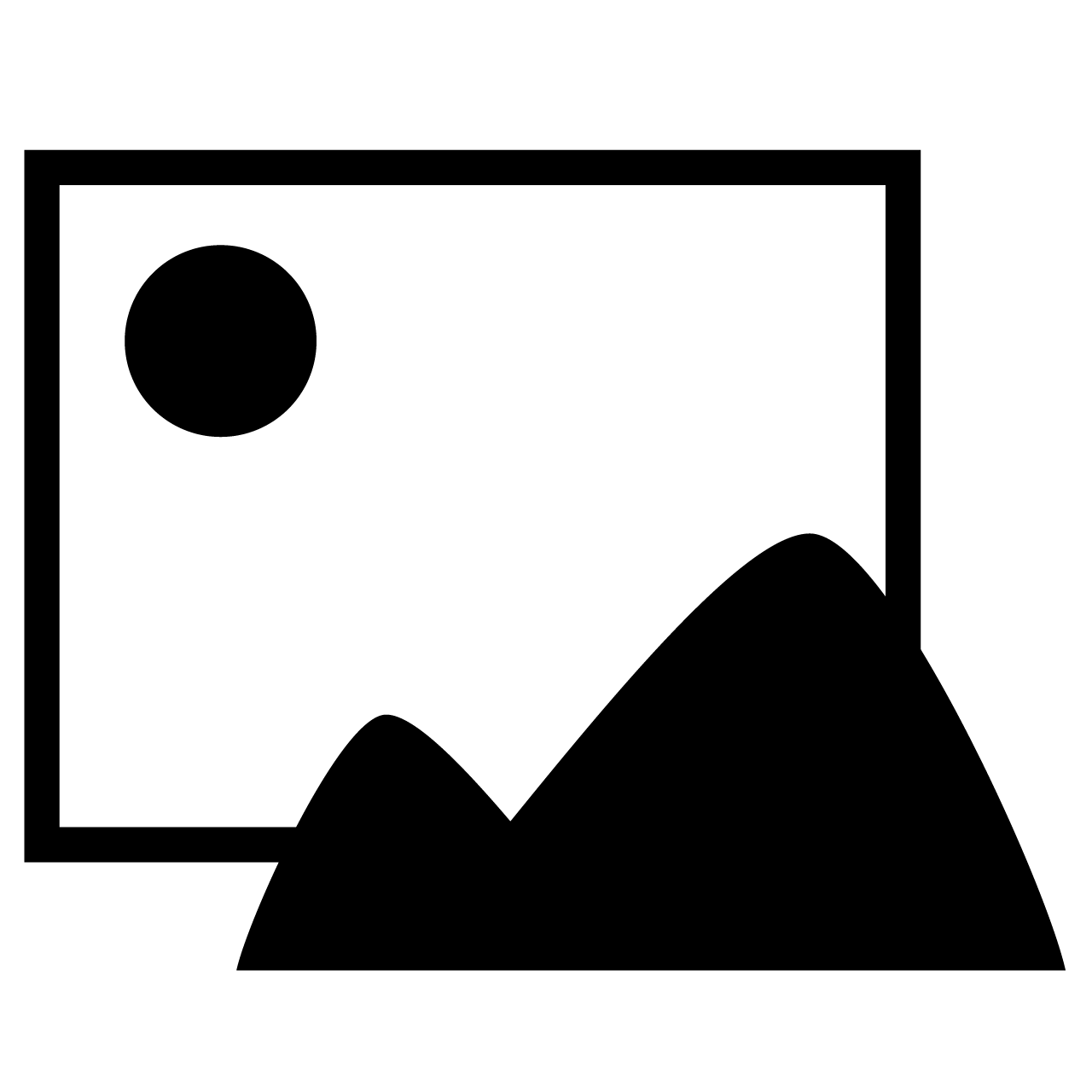
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More