
Batch savePict
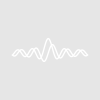
gsb
//saves one or more graphs as enhanced metafiles, or other image file types as specified by the optional type parameter.
#pragma TextEncoding = "Windows-1252"
#pragma rtGlobals=3 // Use modern global access method and strict wave access.
function SavePICT_batch(matchStr,numGraphs, pathName, [h, w, res, nStr,cmdStr,type])
String matchStr //optional matchStr for window names -- pass "" or "*" for top-most graphs
Variable numGraphs
String pathName //specify a symbolic path to the directory for saving the files. User is prompted to select a directory if the symbolic path does not exist
Variable h, w //optionally set height. Uses inches by multiplying input value by 72
Variable res //see otions in switch statement below. 0 or 8 is 8x screen resolution, 1 is 1x, so on
String nStr //when passed, this is used as start of output name. If more than one graph being saved, naming is [nStr]0, [nStr]1, ...
string cmdStr //when passed, disp_general(cmdStr) is run on each graph before saving, e.g. "modifygraph fsize=16"
Variable type //-2 for enhanced metafile, -5 for png, -7 for tiff. See the savePICT /E flag for more details
Variable usedType
if (ParamIsDefault(type)) //no type speficiation, use default
usedType = -2 //-2 for high res enhanced metafile (good for illustrator), -5 for png, -7 for tiff
else
usedType = type
endif
Variable spbt = 1 //Save PNG Background as Transparent (only affects PNGs)
PathInfo $pathName
if (!V_flag)
Newpath/O $pathName
if (V_flag)
Print "SavePICT_batch(): path set canceled; aborting."
endif
endif
if (ParamIsDefault(res))
res = 8
endif
Variable resVal = res*72 //1 to 8 are options for res
if (strlen(matchStr) < 1) //default to all windows
matchStr="*"
endif
String graphWindows = WinList(matchStr, ";", "WIN:1" )
if (!ParamIsDefault(cmdStr))
disp_general(cmdStr, "*", numGraphs)
endif
Variable i, count; String sname
Variable num=min(numGraphs,itemsinlist(graphWindows))
for (i=0; i<num ; i+=1)
//check for height, width changes
if (!ParamIsDefault(h))
ModifyGraph/W=$StringFromList(i, graphWindows) height = h*72; doupdate
ModifyGraph/W=$StringFromList(i, graphWindows) height = 0 //makes height adjustable again
endif
if (!ParamIsDefault(w))
ModifyGraph/W=$StringFromList(i, graphWindows) width = w*72; doupdate
ModifyGraph/W=$StringFromList(i, graphWindows) width = 0 //makes height adjustable again
endif
//check for a custom name
if (ParamIsDefault(nStr)) //no custom name
SavePICT/Z/O/P=$pathName/EF=2/Q=1/B=(resVal)/E=(usedType)/TRAN=(spbt)/WIN=$StringFromList(i, graphWindows)
Print "Saved: " + StringFromList(i, graphWindows)
else
if (numGraphs < 2) //just one input, don't append a number to give it a unique name
sname = nStr
else //multiple windows to save, append a number to make sure output name is unique for each one
sname = nStr + num2str(count)
endif
switch (usedType)
case -2: //emf
sname += ".emf"
break
case -5: //png
sname += ".png"
break
case -7:
sname += ".tif"
break
endswitch
SavePICT/Z/O/P=$pathName/EF=2/E=(usedType)/Q=1/B=(resVal)/TRAN=(spbt)/WIN=$StringFromList(i, graphWindows) as sname
Print "Saved: " + StringFromList(i, graphWindows),"save name =", sname
endif
if (V_flag == 0)
count+=1
else
Print "Error in SavePICT_batch() from SavePICT. Error code = " + num2str(V_flag)
endif
endfor
Print "total num saved = " + num2str(count) + " of target num = " + num2str(numGraphs)
end
function disp_general(cmdStr, matchStr, numGraphs)
String cmdStr, matchStr; Variable numGraphs
if (strlen(matchStr) < 1)
matchStr = "*" //defaul to all graphs
endif
String list = winlist(matchStr,";","WIN:1")
Variable i,numWins=min(numGraphs,itemsinlist(list)); String winN;
for (i=0;i<numWins;i+=1)
winN = StringFromList(i, list)
Dowindow/F $winN //bring to front
Execute cmdStr
endfor
dowindow/H/F //send the command window back to the top of the desktop.
end
#pragma TextEncoding = "Windows-1252"
#pragma rtGlobals=3 // Use modern global access method and strict wave access.
function SavePICT_batch(matchStr,numGraphs, pathName, [h, w, res, nStr,cmdStr,type])
String matchStr //optional matchStr for window names -- pass "" or "*" for top-most graphs
Variable numGraphs
String pathName //specify a symbolic path to the directory for saving the files. User is prompted to select a directory if the symbolic path does not exist
Variable h, w //optionally set height. Uses inches by multiplying input value by 72
Variable res //see otions in switch statement below. 0 or 8 is 8x screen resolution, 1 is 1x, so on
String nStr //when passed, this is used as start of output name. If more than one graph being saved, naming is [nStr]0, [nStr]1, ...
string cmdStr //when passed, disp_general(cmdStr) is run on each graph before saving, e.g. "modifygraph fsize=16"
Variable type //-2 for enhanced metafile, -5 for png, -7 for tiff. See the savePICT /E flag for more details
Variable usedType
if (ParamIsDefault(type)) //no type speficiation, use default
usedType = -2 //-2 for high res enhanced metafile (good for illustrator), -5 for png, -7 for tiff
else
usedType = type
endif
Variable spbt = 1 //Save PNG Background as Transparent (only affects PNGs)
PathInfo $pathName
if (!V_flag)
Newpath/O $pathName
if (V_flag)
Print "SavePICT_batch(): path set canceled; aborting."
endif
endif
if (ParamIsDefault(res))
res = 8
endif
Variable resVal = res*72 //1 to 8 are options for res
if (strlen(matchStr) < 1) //default to all windows
matchStr="*"
endif
String graphWindows = WinList(matchStr, ";", "WIN:1" )
if (!ParamIsDefault(cmdStr))
disp_general(cmdStr, "*", numGraphs)
endif
Variable i, count; String sname
Variable num=min(numGraphs,itemsinlist(graphWindows))
for (i=0; i<num ; i+=1)
//check for height, width changes
if (!ParamIsDefault(h))
ModifyGraph/W=$StringFromList(i, graphWindows) height = h*72; doupdate
ModifyGraph/W=$StringFromList(i, graphWindows) height = 0 //makes height adjustable again
endif
if (!ParamIsDefault(w))
ModifyGraph/W=$StringFromList(i, graphWindows) width = w*72; doupdate
ModifyGraph/W=$StringFromList(i, graphWindows) width = 0 //makes height adjustable again
endif
//check for a custom name
if (ParamIsDefault(nStr)) //no custom name
SavePICT/Z/O/P=$pathName/EF=2/Q=1/B=(resVal)/E=(usedType)/TRAN=(spbt)/WIN=$StringFromList(i, graphWindows)
Print "Saved: " + StringFromList(i, graphWindows)
else
if (numGraphs < 2) //just one input, don't append a number to give it a unique name
sname = nStr
else //multiple windows to save, append a number to make sure output name is unique for each one
sname = nStr + num2str(count)
endif
switch (usedType)
case -2: //emf
sname += ".emf"
break
case -5: //png
sname += ".png"
break
case -7:
sname += ".tif"
break
endswitch
SavePICT/Z/O/P=$pathName/EF=2/E=(usedType)/Q=1/B=(resVal)/TRAN=(spbt)/WIN=$StringFromList(i, graphWindows) as sname
Print "Saved: " + StringFromList(i, graphWindows),"save name =", sname
endif
if (V_flag == 0)
count+=1
else
Print "Error in SavePICT_batch() from SavePICT. Error code = " + num2str(V_flag)
endif
endfor
Print "total num saved = " + num2str(count) + " of target num = " + num2str(numGraphs)
end
function disp_general(cmdStr, matchStr, numGraphs)
String cmdStr, matchStr; Variable numGraphs
if (strlen(matchStr) < 1)
matchStr = "*" //defaul to all graphs
endif
String list = winlist(matchStr,";","WIN:1")
Variable i,numWins=min(numGraphs,itemsinlist(list)); String winN;
for (i=0;i<numWins;i+=1)
winN = StringFromList(i, list)
Dowindow/F $winN //bring to front
Execute cmdStr
endfor
dowindow/H/F //send the command window back to the top of the desktop.
end
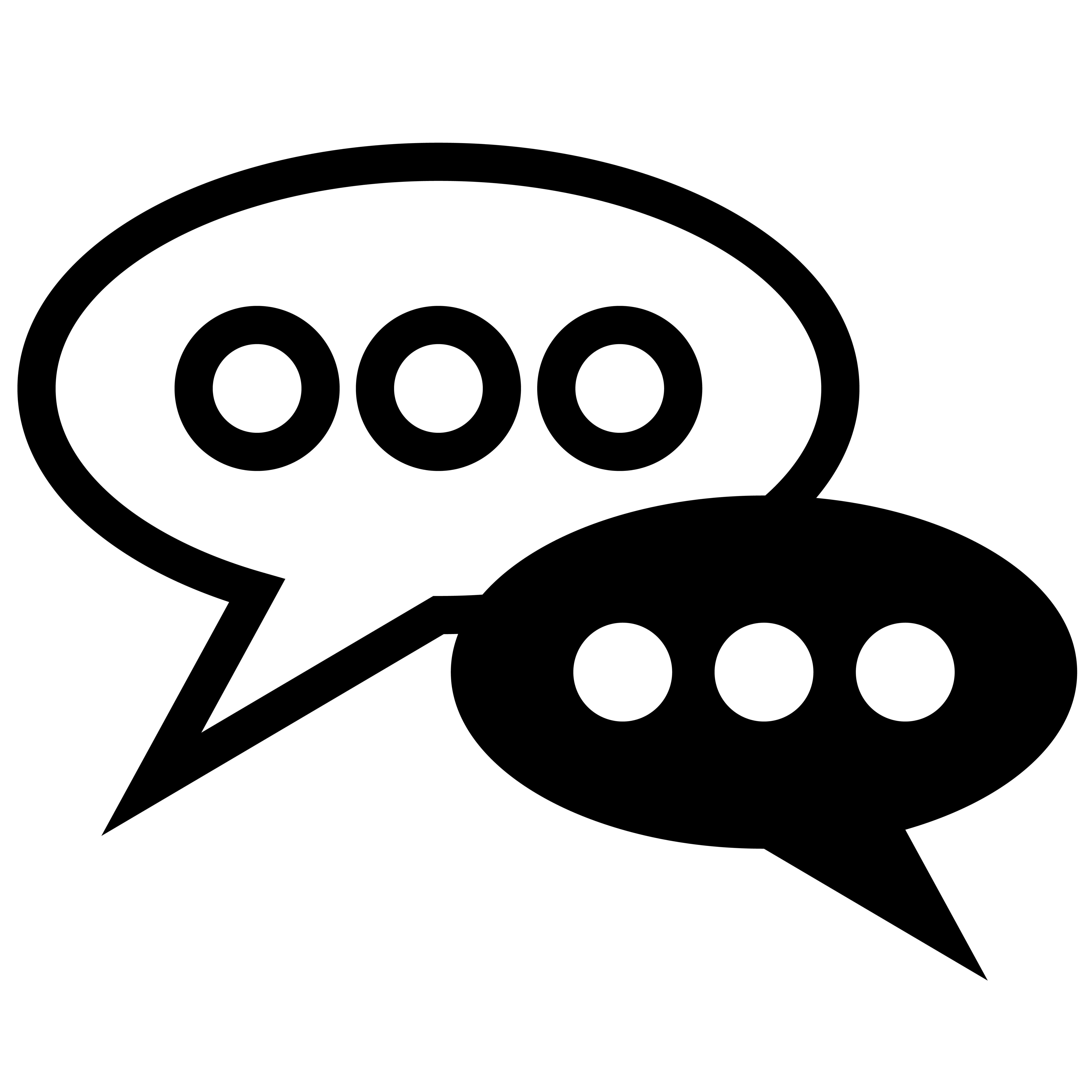
Forum
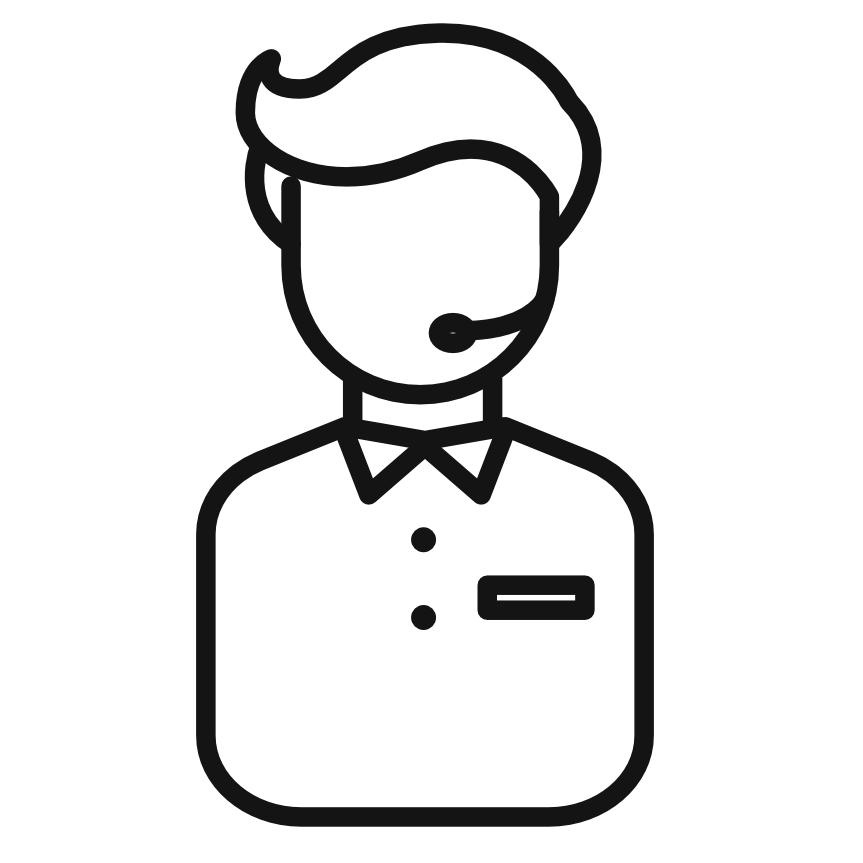
Support
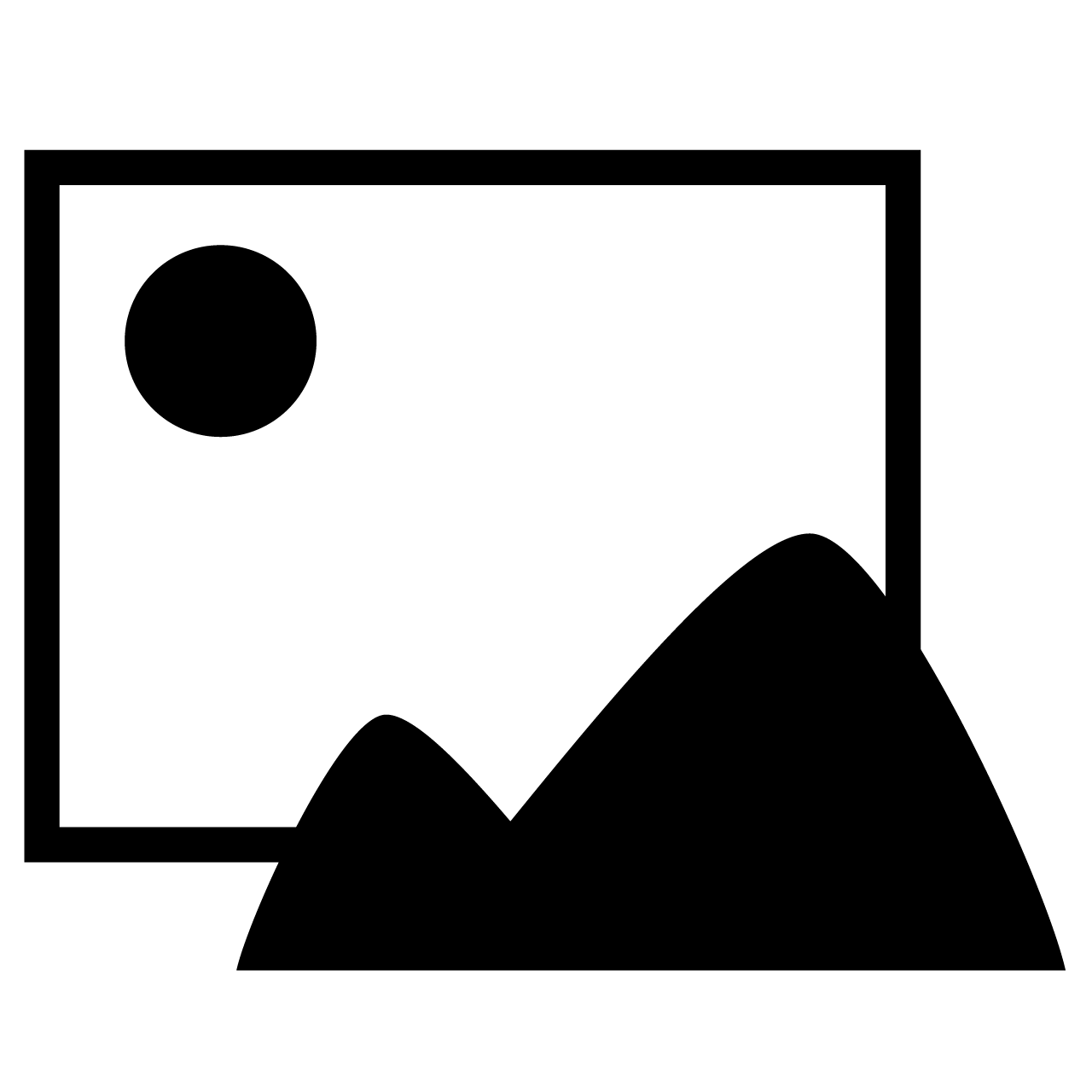
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More