
Assess whether the fit gives error in a function
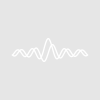
ankit7540
Wed, 05/08/2024 - 07:09 am
I am performing curve fit in a defined function for 2D arrays. Each column in the 2D wave is fit one by one in a for loop. For some of the columns, the fit likely fails since it gives message box at the end of the run saying that
`While executing FuncFit, the following error occurred: singular matrix or other numeric error`
as shown in the image below.
I want to find out for which columns the FuncFit fails. Is there a way to return the fit status from FuncFit or the fit status stored in a variable. It seems that the documentation does not make this clear.
Thanks.
Take a look at this curve fitting help topic: DisplayHelpTopic "Special Variables for Curve Fitting"
In particular, you need the variable V_FitError. You might also want V_FitQuitReason, but the singular matrix error will be caught by V_FitError.
To use it, you must create the variable and set it to zero. After the fit, check it for non-zero:
FuncFit ....
if (V_fitError)
.... do something with the error ....
endif
May 8, 2024 at 09:41 am - Permalink
Thank you for the direction. I checked the help topic as suggested and found it very useful.
May 8, 2024 at 08:15 pm - Permalink
If you expect occasional fit errors in a loop, you might want to suppress these error messages and handle them in code only. For this you can use the try-catch environment. Here is some dummy code:
FuncFit/Q myFitFunc W_coef, myData; AbortOnRTE
catch
if (V_AbortCode == -4) // AbortOnRTE triggered
variable cfError = GetRTError(1) // 1 to clear the error
Print "Error:", GetErrMessage(cfError,3) // debug
endif
endtry
You can read more here:
DisplayHelpTopic "try-catch-endtry"
May 9, 2024 at 12:09 am - Permalink
In reply to If you expect occasional fit… by chozo
Thank you for this example on try-catch. This is exactly what I needed.
May 12, 2024 at 06:41 pm - Permalink